Building a full-stack React application combines the power of a dynamic frontend with a robust backend, enabling developers to create seamless, feature-rich user experiences. Whether you’re a seasoned React JS developer or just starting your journey, mastering the full-stack setup is an essential skill.
In this blog post, we’ll walk you through the steps to set up a full-stack React application. If you’re part of a React JS development company or providing React JS development services, this guide will help streamline your development process.
What is a Full-Stack React Application?
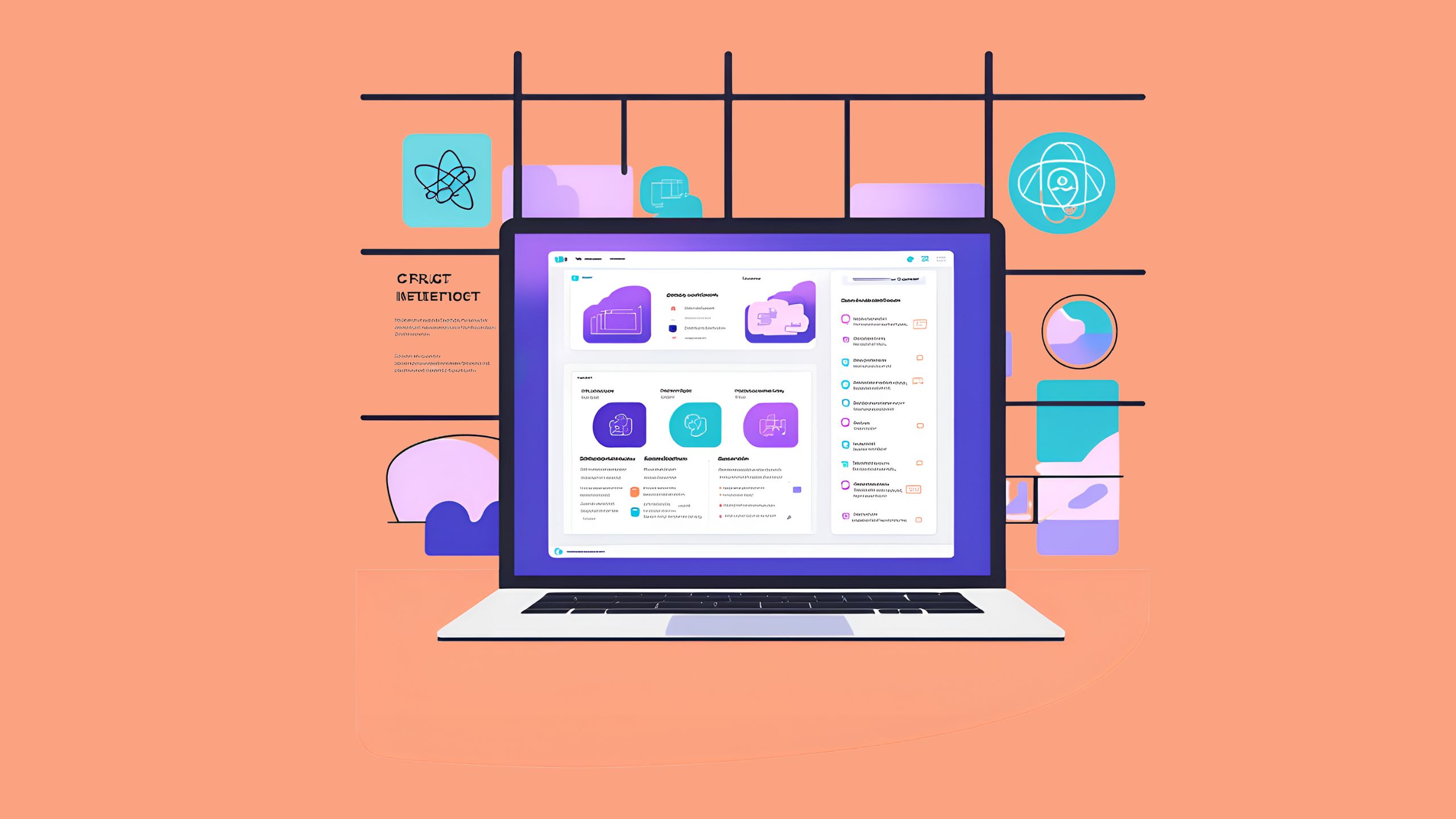
A full-stack React application combines:
Frontend: Built with React, delivering an interactive user interface.
Backend: Typically powered by Node.js and Express, handling data processing and APIs.
Database: A database like MongoDB, MySQL, or PostgreSQL to store and manage data.
Full-stack React apps are popular for React web development, React mobile app development, and more due to their scalability and performance.
Benefits of Full-Stack React Development
Unified Development: Use JavaScript for both frontend and backend.
Improved Performance: React’s virtual DOM and backend optimization enhance speed.
Scalability: Easily scale components and backend services.
Developer Productivity: Reusable components and consistent codebases speed up development.
Customizable: Ideal for projects like React software, web platforms, or React website development.
Launch High-Performance ReactJS Applications!
Work with SDLC CORP to transform your ideas into stunning digital products.
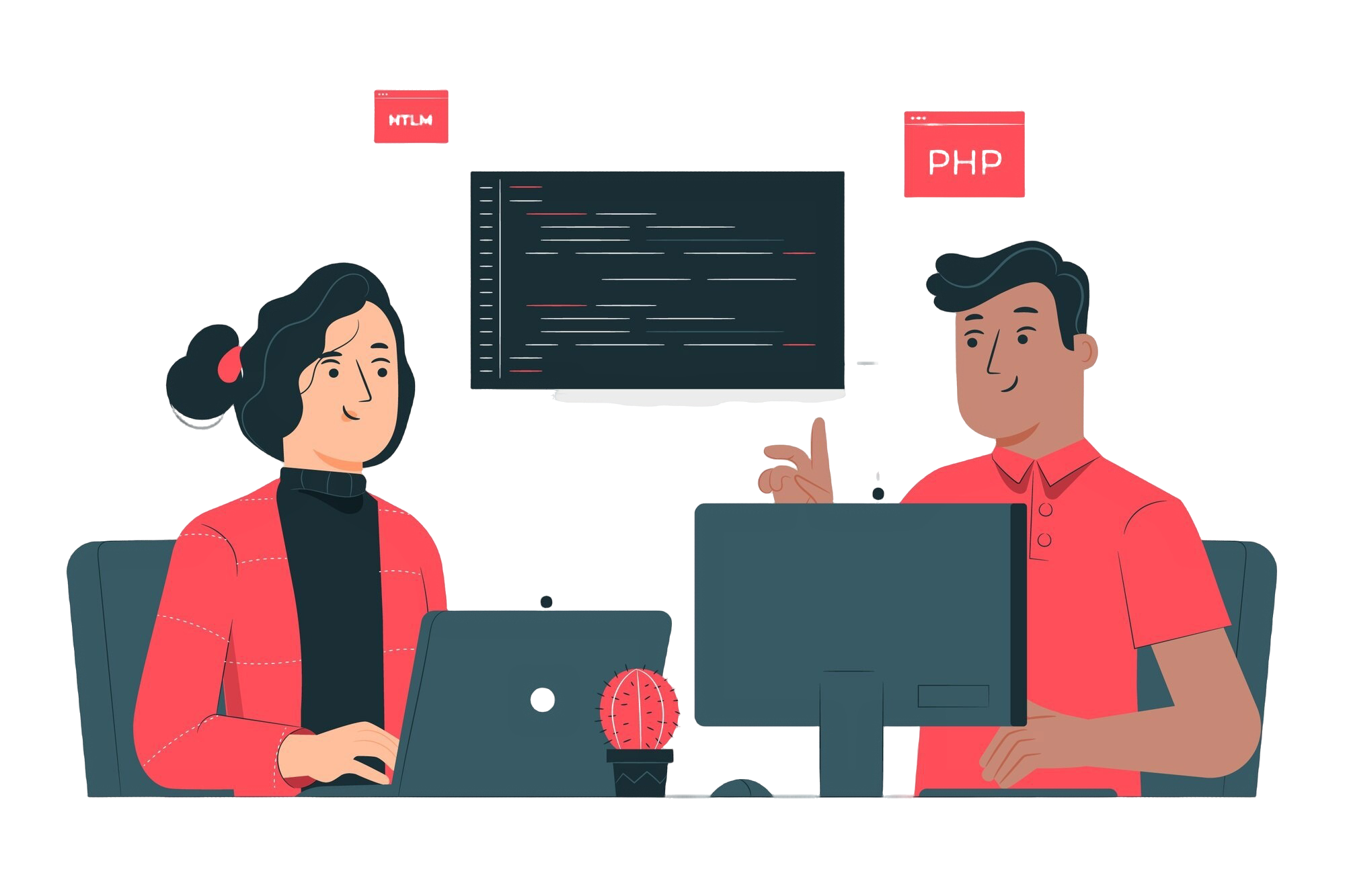
Step-by-Step Guide to Setting Up a Full-Stack React Application
Step 1: Create a React App
Navigate to your project folder and create a React app:
npx create-react-app fullstack-app-frontend
Step 2: Install Axios for API Calls
Axios simplifies API requests between the frontend and backend. Install it in the React project:
npm install axios
Step 3: Connect to the Backend
In the React app, create a services
folder with an api.js
file:
import axios from 'axios';
const API = axios.create({ baseURL: 'http://localhost:5000' });
export const fetchBackendData = () => API.get('/');
Test the connection by creating a simple React component:
import React, { useEffect, useState } from 'react';
import { fetchBackendData } from './services/api';
const App = () => {
const [data, setData] = useState('');
useEffect(() => {
fetchBackendData()
.then(response => setData(response.data))
.catch(error => console.error(error));
}, []);
return <h1>{data}</h1>;
};
export default App;
Run the React app to confirm the connection works.
Add Database Integration
Step 1: Set Up MongoDB
Install MongoDB locally or use a cloud service like MongoDB Atlas. Update the index.js
file in the backend to connect MongoDB:
mongoose.connect(process.env.MONGO_URI, {
useNewUrlParser: true,
useUnifiedTopology: true,
})
.then(() => console.log('MongoDB Connected'))
.catch(error => console.error('MongoDB connection error:', error));
Add a .env
file to the backend project:
MONGO_URI=mongodb+srv://<username>:<password>@cluster.mongodb.net/<database>
Step 2: Create a Data Model
Create a model for storing data. For example, a simple “todo” model:
Build Fast, Scalable ReactJS Apps Today!
Partner with SDLC CORP for top-notch ReactJS development solutions.
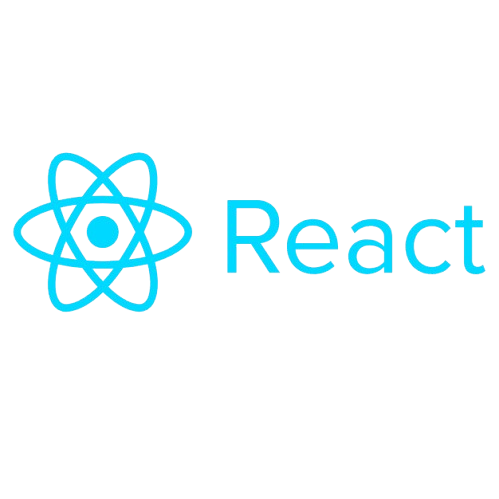
Create API Endpoints
Add API routes to handle data operations:
const express = require('express');
const Todo = require('./models/Todo');
const router = express.Router();
// Get all todos
router.get('/todos', async (req, res) => {
const todos = await Todo.find();
res.json(todos);
});
// Add a new todo
router.post('/todos', async (req, res) => {
const newTodo = new Todo({ task: req.body.task });
const savedTodo = await newTodo.save();
res.json(savedTodo);
});
module.exports = router;
Integrate these routes into your index.js
file:
const todoRoutes = require(‘./routes/todos’);
app.use(‘/api’, todoRoutes);
Fetch Data in React
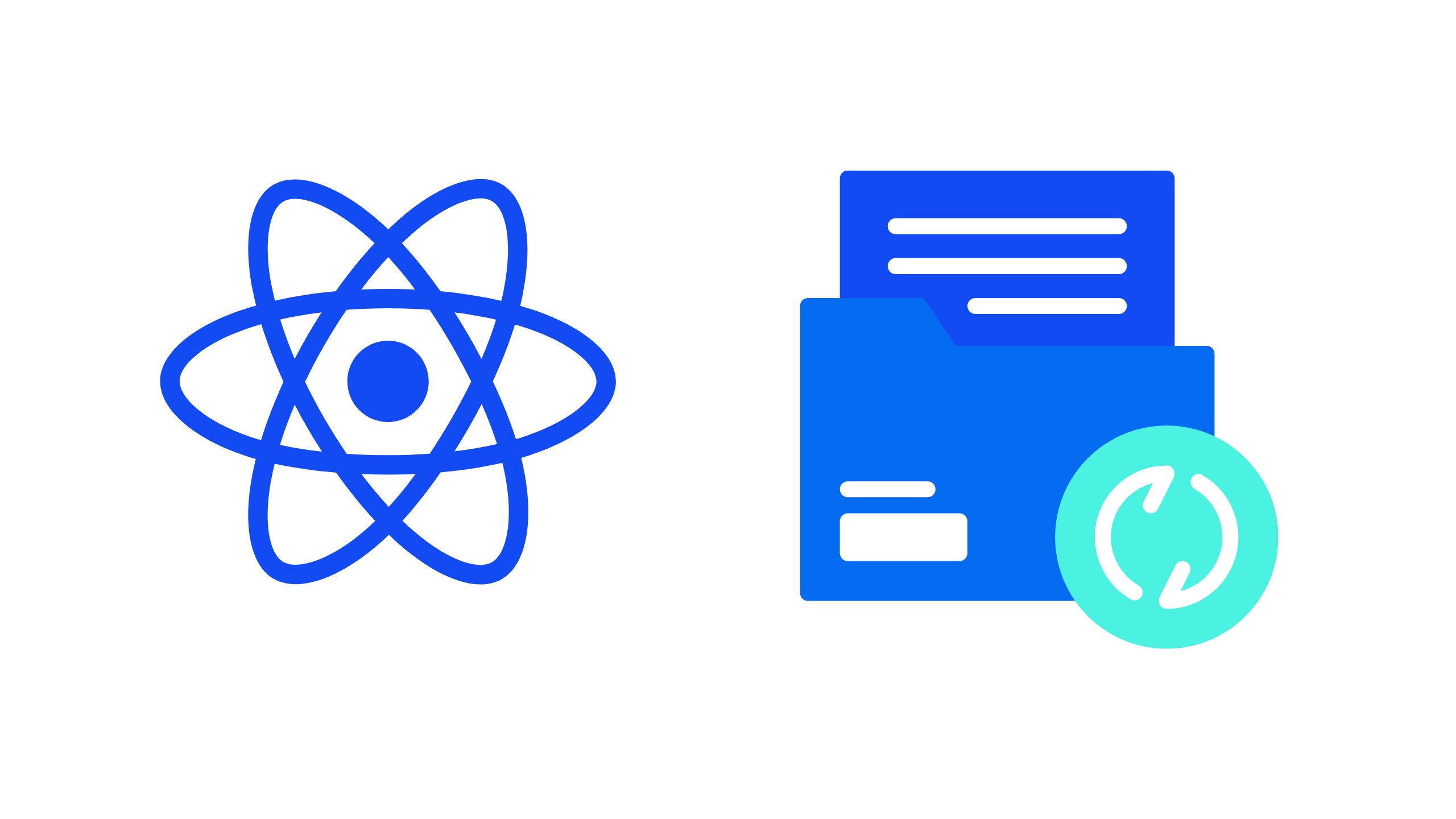
Update your React app to fetch and display todos:
import React, { useEffect, useState } from ‘react’;
import axios from ‘axios’;
const App = () => {
const [todos, setTodos] = useState([]);
useEffect(() => {
axios.get(‘http://localhost:5000/api/todos’)
.then(response => setTodos(response.data))
.catch(error => console.error(error));
}, []);
return (
<ul>
{todos.map(todo => (
<li key={todo._id}>{todo.task}</li>
))}
</ul>
);
};
export default App;
Best Practices for Full-Stack React Development
Use Environment Variables: Secure sensitive information like API keys and database credentials.
Implement Error Handling: Handle API errors gracefully.
Optimize Performance: Use pagination for large datasets.
Collaborate Effectively: Teams offering React JS development services should align on backend and frontend expectations.
Test Thoroughly: Use tools like Jest for React and Mocha for backend testing.
Accelerate Your Business with ReactJS!
Choose SDLC CORP for dynamic, responsive, and scalable ReactJS development.
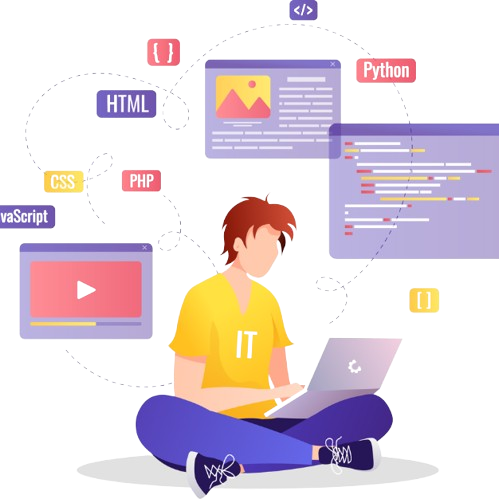
Conclusion
Setting up a full-stack React application is a rewarding process that unlocks endless possibilities for creating dynamic web and mobile applications. Whether you’re developing for a client as part of a React development company or working on personal projects, mastering these skills will enhance your efficiency and expertise.
Ready to build your next app? Dive into full-stack development and explore the power of combining React with a robust backend system!
SDLC CORP ReactJS Development Services Overview
SDLC CORP offers top-notch React website development services to create dynamic, scalable, and user-friendly web applications. Our expert team leverages ReactJS to deliver high-performance solutions tailored to meet your business needs, ensuring a seamless user experience and robust functionality. Partner with us to build innovative, modern websites that drive growth and engagement.