Introduction
Creating a video game is not just about creativity; it’s also about handling a lot of coding and problem-solving behind the scenes. Many people think it’s all about designing cool characters or coming up with a fun story, but there’s a technical side that is equally important. This guide will walk you through the technical process, so whether you’re working on your own or with a video game development company, you’ll know what to expect and how to tackle it.
Unlock the Origins of Gaming Technology Today
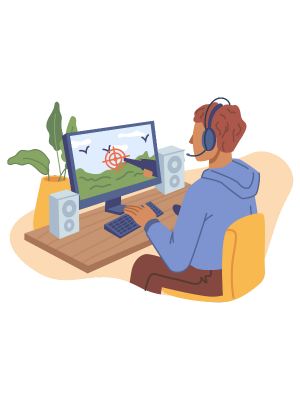
Step 1: Choosing the Right Game Development Engine
One of the first and most important decisions you’ll make is choosing the game development engine. A game engine is like the foundation of your game. It provides the tools and framework to build everything from characters to environments.
The most popular engines are Unity, Unreal Engine, and Godot, each offering different benefits:
- Unity: A favorite for both 2D and 3D games, Unity is known for its user-friendly interface and large community. Plus, there are plenty of resources to help beginners.
- Unreal Engine: Known for its high-quality graphics, Unreal Engine is often used in AAA games. It’s powerful but can be a bit more challenging if you’re just starting out.
- Godot: If you’re looking for something lightweight and open-source, Godot is a great option. It’s simple and clean, especially for 2D games.
Beginner Tip: If you’re just starting, Unity or Godot might be easier to begin with because they have user-friendly interfaces. Unity also has a huge community, so if you get stuck, help is always around the corner.
Step 2: Learning a Programming Language
Every video game runs on code. Learning how to code is essential because it’s what brings your game’s mechanics to life. Each game engine has its own language:
- Unity uses C#, which is straightforward and beginner-friendly.
- Unreal Engine uses C++, which is powerful but more complex.
- Godot uses GDScript, a Python-like language that’s easy to learn.
Here’s an example of a simple code in Unity that makes a character move
void Update()
{
float moveHorizontal = Input.GetAxis(“Horizontal”);
float moveVertical = Input.GetAxis(“Vertical”);
Vector3 movement = new Vector3(moveHorizontal, 0.0f, moveVertical);
transform.Translate(movement * speed * Time.deltaTime);
}
What does this code do?
This code makes your character move based on player input. It checks for horizontal and vertical movement (like pressing the arrow keys), then moves the player in the game. The “Translate” function updates the player’s position in real time.
Whether you handle this coding yourself or hire a video game developer, understanding the basics of programming will help you communicate your vision better.
Step 3: Game Design Documentation (GDD)
Before you even start coding or designing, you need a plan. This plan is called a Game Design Document (GDD). It’s like the blueprint of your game, outlining every detail, from mechanics to character abilities, and even how levels are structured.
Think of the GDD like a blueprint for a house. Just as you wouldn’t build a house without a clear plan, you shouldn’t start coding your game without writing down how everything should work. Whether you’re working alone or with a video game development company, a solid GDD helps keep everything organized.
Step 4: Creating Game Assets (Art, Sound, and Animation)
Your game needs more than just code. It needs visuals, sounds, and animations to bring it to life. The characters, environments, and music all make a huge difference in how your game feels.
Here are some tools you can use to create game assets:
- Blender for 3D modeling (great for creating characters or environments).
- Photoshop or GIMP for creating textures and 2D art.
- Audacity for sound editing and creating effects.
Beginner Tip: If you’re looking for free alternatives, try GIMP (a free tool similar to Photoshop) or MagicaVoxel for simpler 3D modeling. These are great tools for beginners and won’t break the bank.
Step 5: Building the Game World
Now that you have your assets, it’s time to build the world where your game takes place. Whether it’s a vast city or a simple puzzle room, this is where your creativity really shines.
How do you build it?
Most game engines offer an editor where you can place objects, build landscapes, and create levels. In Unity, for example, you can use the Terrain Editor to sculpt landscapes and add trees, rocks, and water.
If you want your world to be different every time a player enters, you might use procedural generation. Here’s an example using Perlin Noise to randomly generate terrain:
for (int x = 0; x < width; x++)
{
for (int y = 0; y < height; y++)
{
float heightValue = Mathf.PerlinNoise(x * scale, y * scale);
terrainData[x, y] = heightValue;
}
}
This code generates landscapes on the fly, adding variety to your game world. Many game development companies use techniques like this to create dynamic, ever-changing environments.
Step 6: Programming Game Mechanics
Game mechanics are the rules that dictate how your game works. These include anything from jumping, shooting, collecting items, or solving puzzles. Let’s look at a few common mechanics and how you might implement them:
- Jumping: Here’s a basic jumping mechanic in Unity:
void Jump()
{
if (isGrounded && Input.GetKeyDown(KeyCode.Space))
{
rb.AddForce(Vector3.up * jumpForce, ForceMode.Impulse);
}
}
This code makes the player jump when the spacebar is pressed, but only if the character is on the ground.
- Collecting Items: In many games, players collect coins or power-ups. You could write a script that triggers when the player touches an item.
- Solving Puzzles: For puzzle games, you might need to track the player’s progress and check if they’ve solved a certain puzzle before moving on.
Whether you’re building these yourself or working with a video game development company, game mechanics are what make your game fun to play.
Step 7: Implementing Physics and AI
To make your game feel realistic, you need to implement physics. For example, if your character jumps, gravity should pull them back down. Most game engines come with built-in physics, so you don’t need to program everything from scratch.
- Unity uses a system called Rigidbody, which adds physics like gravity and collision detection to objects.
- Unreal Engine has a similar system that handles these interactions.
For AI (artificial intelligence), you might want enemies that follow the player or NPCs that react to certain actions. You can program this using AI pathfinding. Unity’s NavMesh helps AI characters navigate through complex environments.
Step 8: User Interface (UI) Development
Your game also needs a user interface (UI). This includes things like menus, health bars, and score displays. A good UI is essential for giving the player feedback and making the game more enjoyable to navigate.
Example: In Unity, you can create a simple main menu where the player clicks “Play” to start the game:
public void OnPlayButtonClicked()
{
SceneManager.LoadScene(“MainScene”);
}
This code switches scenes when the “Play” button is clicked. Whether you’re working alone or using video game development services, crafting a good UI is crucial to the player experience.
Step 9: Debugging, Optimization, and Playtesting
Once your game is playable, the next step is to test it thoroughly. Debugging involves fixing errors in the game, while optimization ensures that your game runs smoothly without lag or crashes. But don’t just stop there, playtesting is equally important.
Playtesting Tip: Let others play your game before it’s finalized. Playtesting can reveal issues you might miss as a developer. Feedback from real players is crucial to fine-tuning your game and ensuring it’s fun to play.
Whether you hire a video game developer or use video game development services, playtesting and feedback gathering are crucial for a successful game launch.
Step 10: Deployment and Distribution
Finally, you’ll need to deploy your game to different platforms. Unity makes it easy to export your game for multiple platforms, such as PC, mobile, or consoles. Before you release your game, make sure to optimize it for each platform mobile controls, for instance, are very different from PC or console controls.
Most game development companies will help you with this step, ensuring that your game is optimized for its platform and ready for distribution. Consider releasing your game on platforms like Steam, Google Play, or the Apple App Store.
Dive Into Gaming's History for Future Innovation

Conclusion: Start Building Your Dream Game Today!
Every game starts with a small idea, and step by step, that idea can grow into something amazing. No matter how daunting it seems, anyone can create a game with the right dedication and tools. Whether you’re working solo or with a video game development company, you have everything you need to bring your vision to life. So why wait? Start building your dream game today!