React is widely used for building dynamic and scalable applications. As projects grow in size, the JavaScript bundle also increases. Large bundle sizes result in slower load times. To address this, React provides built-in features like code splitting and lazy loading. These techniques allow developers to split the code into smaller chunks. It loads only what’s needed at a specific time.
Working with a reliable React JS development company ensures your applications are optimized for performance. This approach enhances user experiences by improving speed and efficiency. Let’s understand the importance of these techniques.
What is Code Splitting in React?
Code splitting is a technique that breaks the JavaScript bundle into smaller pieces. Instead of loading the entire app at once, it loads parts of the code on demand. This reduces the initial page load time significantly. Code splitting works well for large applications with many components.
React offers support for code splitting through dynamic imports. It allows you to import specific components only when needed. Tools like Webpack and React Router help implement this technique efficiently. Businesses that provide React JS development services can integrate code splitting seamlessly. This ensures React applications run smoothly, even with complex features.
The benefits of code splitting include reduced load times, improved performance, and enhanced user experiences. It also makes applications more modular and easier to maintain.
ReactJs Development Company
Top ReactJS App and web Development company Services
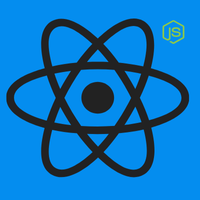
How Does Lazy Loading Work?
Lazy loading complements code splitting by loading components only when necessary. Instead of loading all components upfront, React loads components dynamically. This reduces the overall bundle size at the initial load. Lazy loading is particularly useful for applications with heavy content or unused features.
React provides a React.lazy function to implement lazy loading. Combined with the Suspense component, it allows you to display fallback content until the lazy-loaded component is ready. Companies offering React JS development solutions often use lazy loading to optimize application performance.
Lazy loading works well with features like image galleries, dashboards, and routes. For instance, loading a large dashboard component only when accessed improves app responsiveness. It ensures better resource management and enhances scalability.
Look also this: Mastering Custom Hooks in React: A Beginner’s Guide
Implementing Code Splitting and Lazy Loading in React
Implementing these techniques in React is straightforward. Here’s a step-by-step guide:
Step 1: Use Dynamic Imports
Dynamic imports allow you to split code and load it asynchronously. For example:
import React, { lazy, Suspense } from ‘react’;
const MyComponent = lazy(() => import(‘./MyComponent’));
function App() {
return (
<Suspense fallback={<div>Loading…</div>}>
<MyComponent />
</Suspense>
);
}
This example uses React.lazy to import components dynamically. The Suspense component displays a loading message until the content loads.
Step 2: Code Splitting with React Router
If you’re using React Router, you can combine code splitting with route-based loading:
import { BrowserRouter as Router, Route, Switch } from ‘react-router-dom’;
const Home = lazy(() => import(‘./Home’));
const About = lazy(() => import(‘./About’));
function App() {
return (
<Router>
<Suspense fallback={<div>Loading…</div>}>
<Switch>
<Route path=”/” component={Home} />
<Route path=”/about” component={About} />
</Switch>
</Suspense>
</Router>
);
}
This approach splits routes into smaller chunks and loads them dynamically. Businesses focusing on React web development use this strategy for scalable applications.
Step 3: Use Webpack for Code Splitting
Webpack, a popular bundler, supports code splitting out of the box. It automatically splits code when using dynamic imports. Configure Webpack to optimize your React build, ensuring better performance.
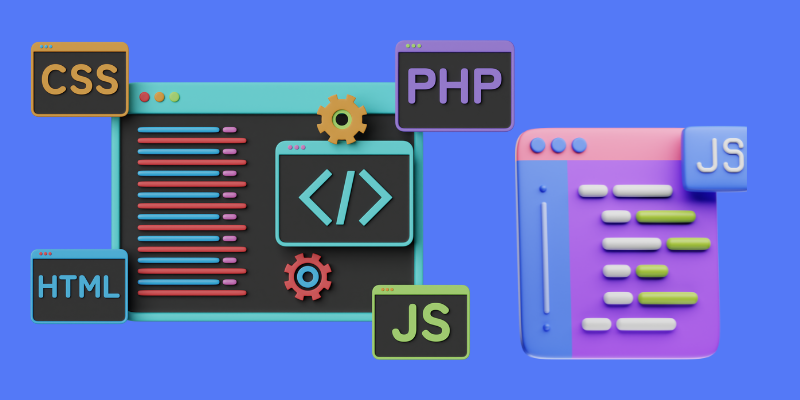
Benefits of Code Splitting and Lazy Loading
The advantages of code splitting and lazy loading are clear. Let’s explore them:
- Faster Load Times: By loading only essential code initially, the page loads faster. This improves user satisfaction and reduces bounce rates.
- Improved Performance: Smaller bundles reduce the strain on browsers, leading to smoother interactions.
- Resource Optimization: Lazy loading ensures resources are used efficiently. Components load only when required.
- Scalability: React applications with modular code splitting scale better as they grow.
Companies offering React development solutions leverage these techniques to deliver high-performing apps. Whether it’s a small project or an enterprise-level app, optimization is key to success.
React Development Best Practices
Optimizing React applications involves following best practices:
- Component-Level Splitting: Break large components into smaller, reusable pieces.
- Optimize Dependencies: Remove unused libraries and optimize imports.
- Monitor Performance: Use tools like React DevTools and Lighthouse for analysis.
- Implement Lazy Loading: Load heavy components only when accessed.
Collaborating with a skilled React developer ensures these practices are implemented effectively. Experienced developers understand how to balance functionality and performance in React apps.
Hire ReactJs Developer
ReactJs Development services offer end-to-end solutions for creating engaging, high-quality ReactJs Development platforms.
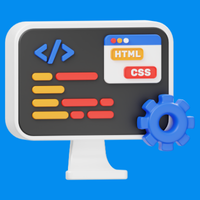
Role of React Development Services
Businesses looking to build optimized applications benefit from professional services. Companies offering React development services provide tailored solutions to meet specific needs. They specialize in implementing performance optimization techniques like code splitting and lazy loading.
These services include app development, optimization, and maintenance. A professional React software development team ensures your applications deliver high performance and scalability. This leads to better user engagement and business growth.
Why Choose React for Web and Mobile Development?
React is versatile and widely used for both web and mobile development. Its component-based architecture allows for modular and reusable code. For businesses, this means faster development cycles and better scalability.
When it comes to React mobile app development, React Native enables the creation of cross-platform apps. It allows businesses to reach both Android and iOS users efficiently. Similarly, React app development for the web ensures responsive and dynamic applications.
Businesses looking to build user-friendly platforms rely on React website development solutions. Whether it’s an e-commerce site or a SaaS product, React provides flexibility and performance.
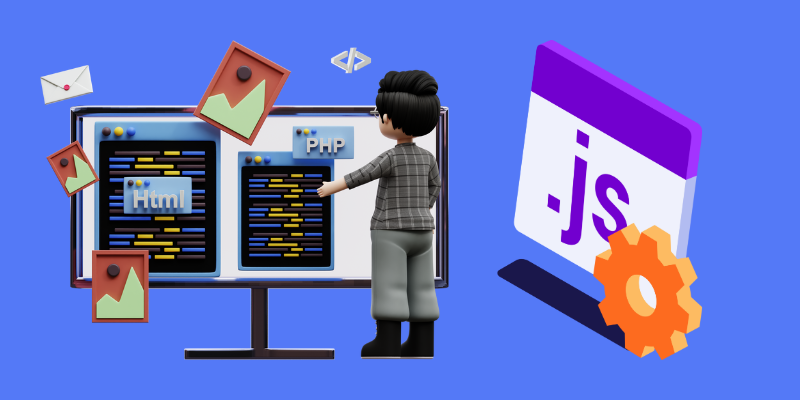
Conclusion
Code splitting and lazy loading are essential techniques for optimizing React applications. They help reduce load times, improve performance, and ensure resource efficiency. Working with a professional React JS development company can make a significant difference in delivering high-quality applications.
By implementing these strategies, businesses can build scalable and high-performing apps. Whether you’re looking for React JS development services or hiring a skilled React developer, optimizing performance should be a priority. Leveraging these tools allows businesses to stay ahead in today’s competitive market.
Boost Profits with Expert ReactJs Development Company Services
Top ReactJS App and web Development company Services
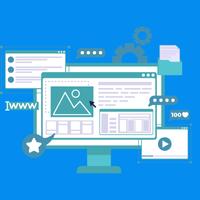
SDLC CORP ReactJs Development Services Overview
SDLC Corp offers top-notch ReactJS development services, delivering fast, scalable, and interactive web applications tailored to your business needs. Our expert developers leverage ReactJS’s component-based architecture to build dynamic user interfaces, ensuring seamless performance, flexibility, and superior user experiences for businesses of all sizes. Partner with us for innovative React solutions.