Dynamic forms have become a staple of modern web applications. Whether you’re building a React mobile app, a React website development project, or a full-scale React software solution, the ability to create responsive, adaptable forms is critical. React, with its powerful state management and component-based architecture, provides a solid foundation for developing dynamic forms that respond to user input and change in real time.
In this blog post, we’ll dive into the essential tips and tricks for creating dynamic forms in React, explore some best practices, and discuss why dynamic forms can be so effective for modern React app development. Whether you’re a seasoned React JS developer or just getting started with React JS development services, the insights shared here will help you streamline your form-building process and create better user experiences.
Why Dynamic Forms?
Before we dive into the specifics of creating dynamic forms, it’s essential to understand why dynamic forms are beneficial for both developers and users.
1. Enhanced User Experience
Dynamic forms offer a more personalized user experience by only showing the fields that are relevant to the user at any given time. For example, when filling out a sign-up form, if the user selects a particular country, the form might dynamically display fields related to postal codes or additional location-specific data. This helps avoid overwhelming the user with unnecessary fields.
2. Improved Data Collection
By dynamically adapting the form structure based on previous inputs, you ensure that the data collected is more accurate and tailored to the user’s needs. This can be especially important in cases where forms collect complex information, like product configurations, surveys, or multi-step processes.
3. Streamlined Development
Building dynamic forms using React web development practices not only improves the user experience but also streamlines the development process. React’s declarative approach to UI management makes it easier to control what gets rendered based on the state, allowing you to build forms that are both functional and easy to maintain.
4. Adaptable and Scalable
As your application grows, forms often evolve in complexity. Whether you’re dealing with product options in an e-commerce app, or multi-step forms in a React mobile app, dynamic forms allow you to scale and modify the form’s behavior as the project develops, without having to rewrite large chunks of code.
Launch High-Performance ReactJS Applications!
Work with SDLC CORP to transform your ideas into stunning digital products.
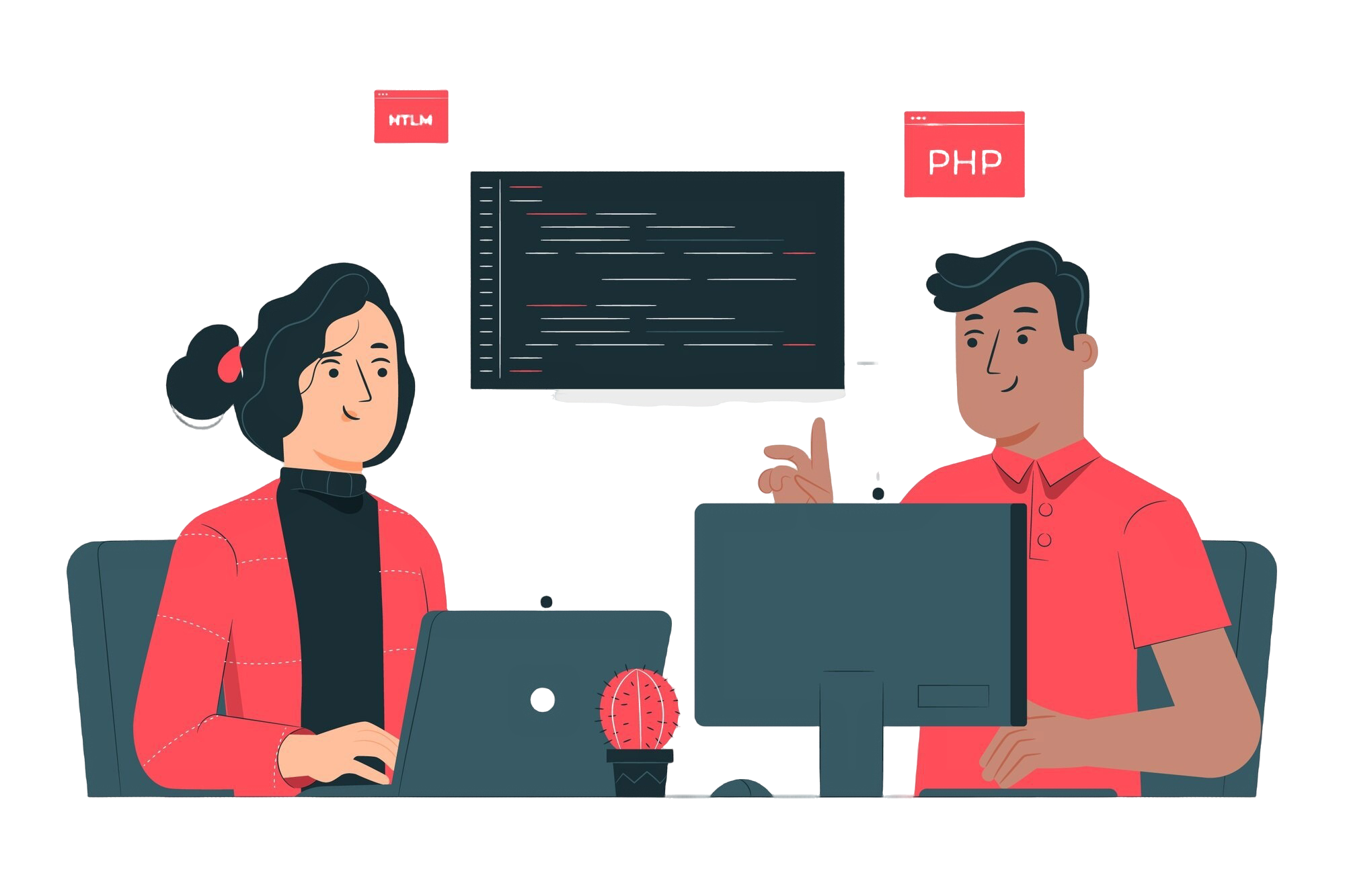
Tips and Tricks for Building Dynamic Forms in React
Now that we understand the importance of dynamic forms, let’s explore some key strategies and tips for creating them effectively in React development.
1. State Management is Key
In React, the state of a form determines the value of each input field. For dynamic forms, managing state efficiently is essential. The most common approach is to store form data in a centralized state object. By using hooks like useState, you can easily manage form field values and update them in response to user interactions. A well-managed state ensures that your form reacts to input changes and renders the correct fields based on those inputs.
For instance, if you’re building a React mobile app or a React web development project that includes user registration, you might want to hide or show certain fields based on the user’s selections. React’s state management allows you to store these changes and dynamically render fields accordingly.
2. Conditional Rendering for Dynamic Fields
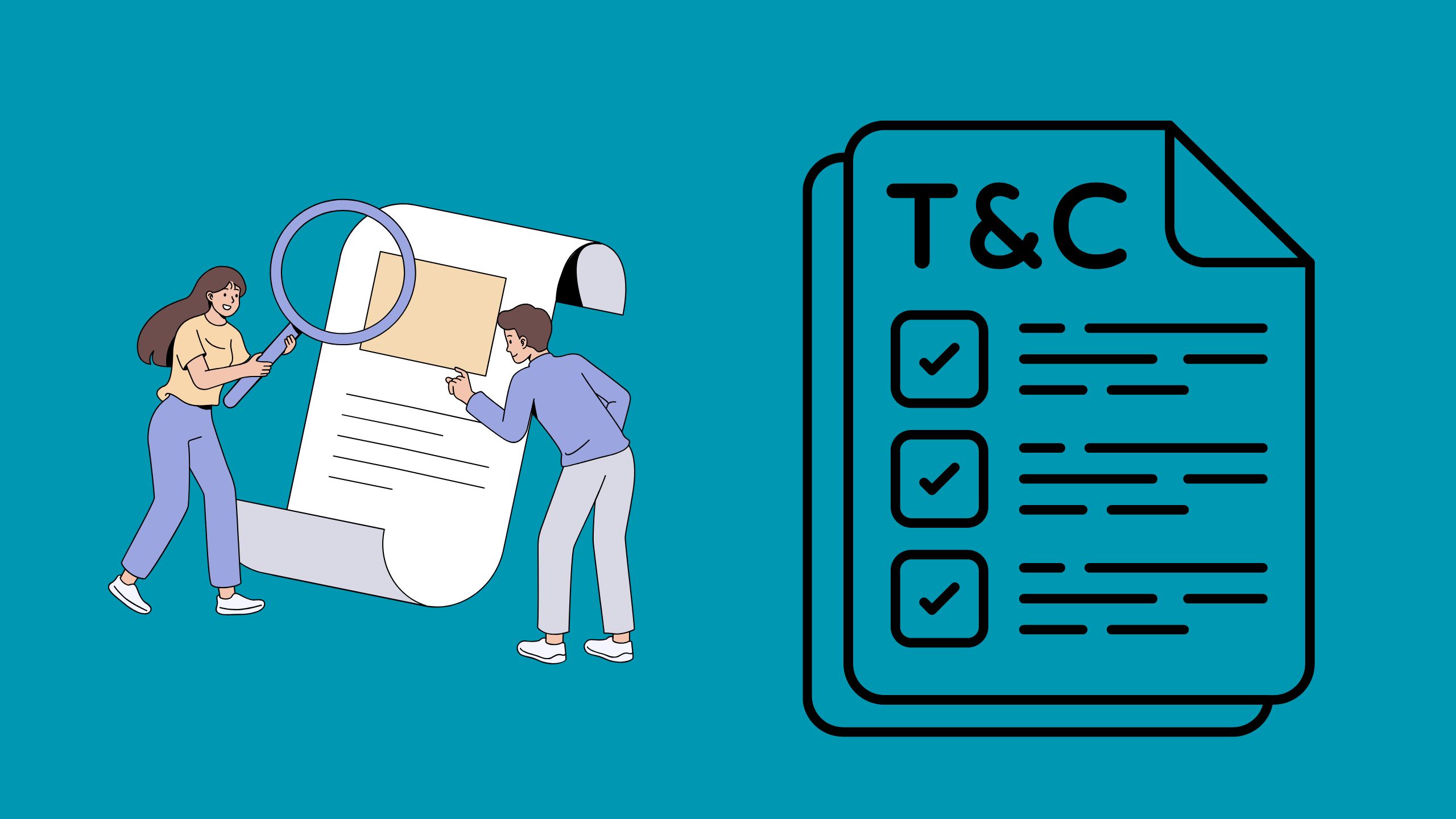
One of the key benefits of React’s component-based architecture is the ability to conditionally render elements. This is crucial for building dynamic forms. For example, when the user selects a specific option, you can dynamically render additional input fields. This not only saves space but also provides a more intuitive form for the user.
Conditional rendering works well in scenarios like:
- Show additional questions based on previous answers: If the user selects “Yes” for a certain option, you can render a follow-up question.
- Display country-specific input fields: Depending on the country the user selects, you might show or hide certain fields, like postal codes, address details, or state-specific options.
React provides the flexibility to add as many conditions as necessary, and you can apply them to both simple and complex forms.
3. Use Dynamic Form Arrays
In many applications, users may need to input multiple items of the same type, such as multiple phone numbers or email addresses. Instead of hard-coding a fixed number of input fields, dynamic form arrays allow users to add or remove fields on the fly.
For example, in a React app development project where users might need to enter multiple phone numbers, a dynamic array allows the form to grow and shrink depending on the number of items the user needs to input. This makes the form more flexible and user-friendly, while keeping the UI clean and concise.
By managing the array of data through React’s state, you ensure that each form element is tied to the state and can be updated as needed.
4. Form Validation for Dynamic Inputs
Validation is a crucial part of any form, but it can be more challenging with dynamic forms because the number and type of fields may change. For instance, you might want to enforce that a user must fill out a postal code only if they’ve selected a certain country, or that a specific field must not be left blank.
In React development services, form validation is often done by tracking the state of each input field and showing error messages accordingly. You can use libraries like Formik or React Hook Form to handle complex validations, or you can write your own custom validation logic to suit your needs. When working with dynamic forms, it’s essential to ensure that the validation rules update as the form changes, ensuring that all fields are correctly validated before submission.
5. Keep Components Modular
When creating dynamic forms, it’s easy to fall into the trap of building large, monolithic components. However, React’s modularity allows you to break the form into smaller, reusable components, which improves readability and maintainability.
For example, instead of building one giant form component, you could split the form into sub-components:
- FormField for individual fields
- SelectField for dropdown selections
- MultiStepForm for multi-step processes
This not only makes your code cleaner but also allows you to reuse components across different forms, reducing duplication and making your app more scalable.
Build Fast, Scalable ReactJS Apps Today!
Partner with SDLC CORP for top-notch ReactJS development solutions.
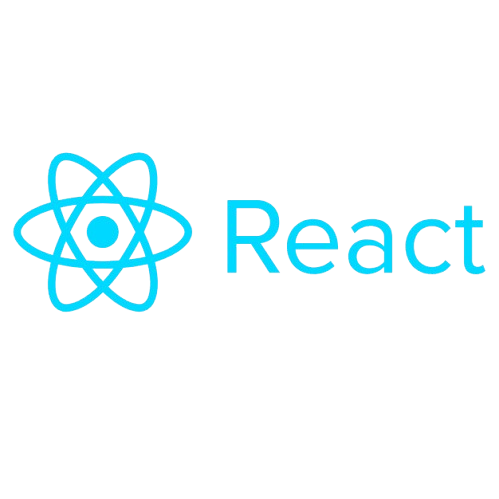
6. Avoid Excessive Re-renders
Performance is a key consideration when building dynamic forms. React re-renders components whenever the state changes, which can lead to performance issues, especially if the form is large or contains many dynamic fields. To avoid unnecessary re-renders, consider using the following techniques:
- React.memo: This React API can help prevent unnecessary re-renders by memoizing the components that don’t need to update when the state changes.
- useCallback: Use this hook to memoize event handlers and ensure they aren’t recreated on every render.
- useMemo: For expensive calculations that are triggered by form state changes, useMemo helps optimize the re-rendering process by caching the result until the dependencies change.
These performance optimization techniques can significantly improve the user experience, especially in large forms or when working with React software solutions where scalability is essential.
7. Leverage Third-Party Libraries
While React provides all the necessary tools to create dynamic forms, you don’t always have to reinvent the wheel. Third-party libraries like Formik, React Hook Form, or Yup (for validation) are excellent choices when you want to save time and effort.
These libraries simplify form handling by managing form state, validation, and submission, allowing you to focus on building the logic specific to your app. For instance, Formik automatically keeps track of the form’s state and handles validation rules, making it an excellent choice for larger applications or forms with complex validation logic.
8. User Feedback and Accessibility
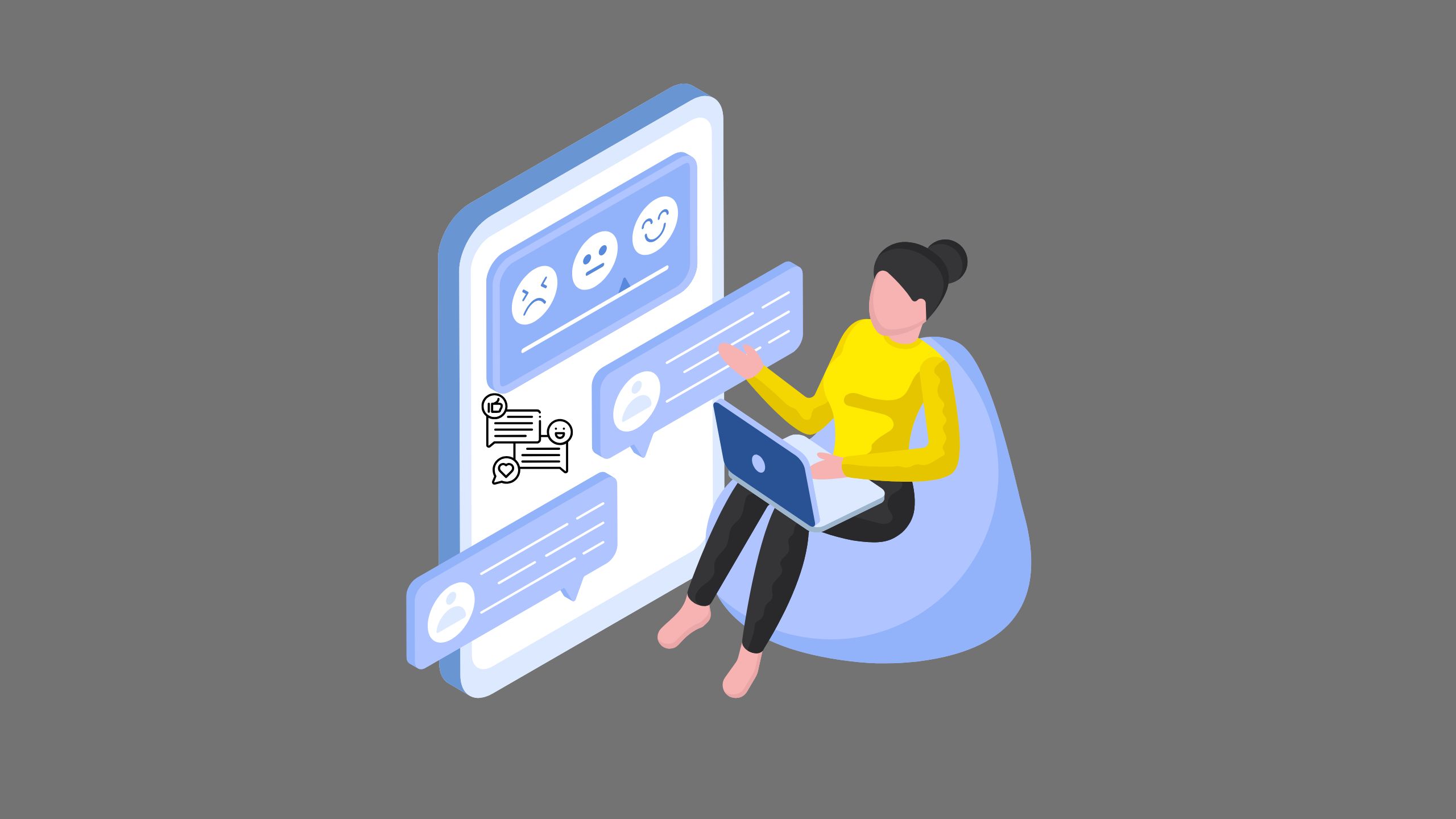
Dynamic forms need to be responsive not only in terms of functionality but also in terms of usability and accessibility. As form fields appear or disappear dynamically, it’s crucial to provide clear visual cues, such as labels, tooltips, or inline validation messages, to help guide the user through the process.
For accessibility, ensure that:
- Forms are navigable with a keyboard.
- Screen readers can detect form changes.
- Labels are correctly associated with input fields.
React provides features like the aria attribute, which can help improve accessibility. Additionally, consider providing helpful error messages when fields are invalid or missing, and use visual cues like highlighting the error fields to draw attention.
Best Practices for Dynamic Forms in React
Here are some additional best practices to follow when building dynamic forms:
- Consistency is key: Ensure that all form fields follow a consistent structure and layout. This makes the form easier to navigate and understand.
- Keep validation simple: Don’t overcomplicate form validation. Start with basic checks, and only add more complex rules when necessary.
- Test across devices: Ensure that your dynamic forms are responsive and work seamlessly across different screen sizes, especially if you’re building a React mobile app.
- Use form libraries when appropriate: Don’t hesitate to leverage libraries like Formik or React Hook Form for easier form management and validation.
- Ensure good user experience: Provide helpful error messages, success messages, and loading indicators to guide the user through the process.
Accelerate Your Business with ReactJS!
Choose SDLC CORP for dynamic, responsive, and scalable ReactJS development.
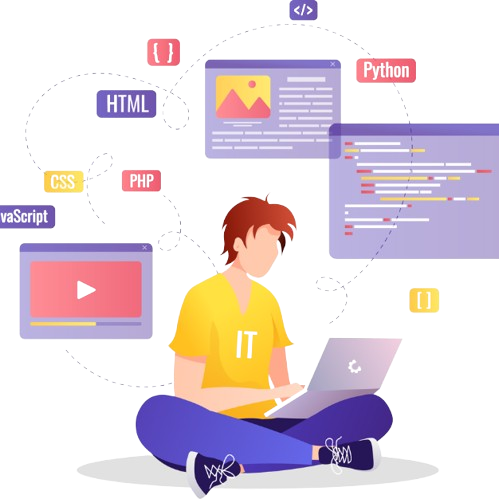
Conclusion
Dynamic forms are an indispensable part of modern web and mobile applications, offering a more personalized and efficient user experience. By mastering key techniques like state management, conditional rendering, and form validation, you can build dynamic forms that adapt to your user’s needs in real time.
Whether you’re working with a React JS development company to build a complex enterprise app or tackling a personal React app development project, the tips and tricks outlined here will help you create dynamic forms that are both functional and user-friendly.
If you’re ready to start building dynamic forms or need assistance with your React development services
SDLC CORP ReactJS Development Services Overview
SDLC CORP offers top-notch React website development services to create dynamic, scalable, and user-friendly web applications. Our expert team leverages ReactJS to deliver high-performance solutions tailored to meet your business needs, ensuring a seamless user experience and robust functionality. Partner with us to build innovative, modern websites that drive growth and engagement.