Introduction
Creating an ERC-20 token allows you to participate in the thriving world of blockchain and decentralized finance. This guide will walk you through the essential steps to create your own ERC-20 token, from defining its purpose and specifications to deploying it on the Ethereum blockchain. Whether you’re an entrepreneur, developer, or enthusiast, understanding this process empowers you to tokenize assets, launch projects, or explore new financial possibilities in the evolving crypto ecosystem. Unlock the potential of ERC-20 tokens with this comprehensive introduction to token creation. Discover how the top 10 ERC-1155 tokens are leading innovation in digital asset management and multi-token standards
Step 1: Set Up Your Development Environment
Before you start coding your token, you’ll need to set up a development environment:
Install Node.js and npm:
- Node.js is a JavaScript runtime environment that allows you to execute JavaScript code outside of a web browser. It also includes npm (Node Package Manager), which is essential for managing dependencies and packages required for your project.
- Installation:
- Visit nodejs.org and download the latest stable version of Node.js.
- Follow the installation instructions for your operating system.
- After installation, verify that Node.js and npm are installed correctly by running node -v and npm -v in your terminal or command prompt.
Install Truffle:
- Truffle is an Ethereum development environment, testing framework, and asset pipeline that simplifies the process of smart contract development, compilation, testing, and deployment.
- Installation:
- Open your terminal or command prompt.
Install Truffle globally by running the following command:
npm install -g truffle
After installation, verify that Truffle is installed correctly by running the truffle version.
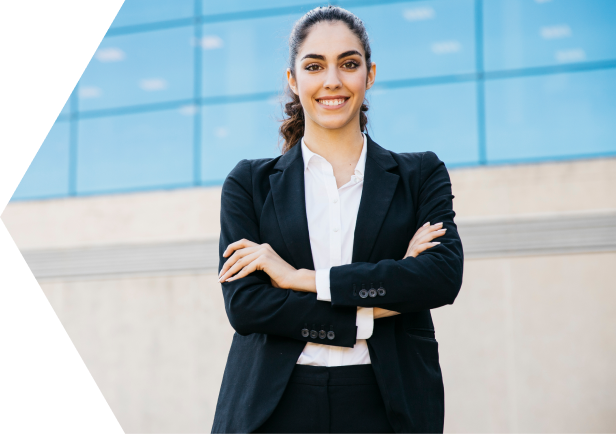
Set up an Ethereum Wallet:
- To interact with the Ethereum blockchain, deploy smart contracts, and manage transactions, you’ll need an Ethereum wallet. One of the most popular wallets for developers is MetaMask.
- Installation:
- Visit metamask.io and install the MetaMask extension for your web browser (Chrome, Firefox, Brave, or Edge).
- Follow the setup instructions to create a new Ethereum wallet or import an existing one.
- Connect MetaMask to the Ethereum test networks like Ropsten or Rinkeby for development purposes.
Get Some Ether:
- Ether (ETH) is the native cryptocurrency of the Ethereum blockchain and is required to deploy smart contracts and interact with the network.
- Using Test Networks:
- For development and testing purposes, you can obtain free Ether (test Ether) from faucets on Ethereum test networks such as Ropsten or Rinkeby.
- Visit the respective test network faucet websites, enter your MetaMask wallet address, and request test Ether.
Setting up your development environment ensures you have the necessary tools and resources to start coding and deploying your ERC-20 token on the Ethereum blockchain.
Step 2: Write the Smart Contract
Use Solidity to write your ERC-20 token contract. Here’s a basic example of what the contract might look like:
solidity
// SPDX-License-Identifier: MIT
pragma solidity ^0.8.0;
contract MyToken {
string public constant name = "MyToken";
string public constant symbol = "MTK";
uint8 public constant decimals = 18;
uint256 public totalSupply = 1000000 * (10 ** uint256(decimals));
mapping(address => uint256) balances;
mapping(address => mapping (address => uint256)) allowed;
event Transfer(address indexed from, address indexed to, uint256 tokens);
event Approval(address indexed owner, address indexed spender, uint256 tokens);
constructor() {
balances[msg.sender] = totalSupply;
}
function balanceOf(address tokenOwner) public view returns (uint256 balance) {
return balances[tokenOwner];
}
function transfer(address to, uint256 tokens) public returns (bool success) {
require(balances[msg.sender] >= tokens);
balances[msg.sender] -= tokens;
balances[to] += tokens;
emit Transfer(msg.sender, to, tokens);
return true;
}
function approve(address spender, uint256 tokens) public returns (bool success) {
allowed[msg.sender][spender] = tokens;
emit Approval(msg.sender, spender, tokens);
return true;
}
function transferFrom(address from, address to, uint256 tokens) public returns (bool success) {
require(tokens <= balances[from]);
require(tokens <= allowed[from][msg.sender]);
balances[from] -= tokens;
balances[to] += tokens;
allowed[from][msg.sender] -= tokens;
emit Transfer(from, to, tokens);
return true;
}
}
Step 3: Compile and Deploy Your Contract
Compile the Contract: Use Truffle to compile your contract, ensuring there are no errors in your Solidity code.
bash
truffle compile
Deploy the Contract: Write a migration script in Truffle to deploy your contract. Then, using Truffle and your MetaMask wallet connected to an Ethereum test network, deploy the contract.
bash
truffle migrate --network ropsten
Step 4: Verify and Interact with Your Contract
Interact with Your Token:
- Once you have deployed your ERC-20 token contract on the Ethereum blockchain, you can start interacting with it. This involves performing actions such as transferring tokens, checking balances, and approving token allowances.
- Using Truffle Console:
- Truffle provides a console that allows you to interact with your deployed contracts directly from the command line interface.
Open your terminal or command prompt and run:
truffle console
Once inside the Truffle console, you can use JavaScript commands to interact with your deployed token contract. For example:
let instance = await YourTokenContract.deployed();
let balance = await instance.balanceOf(yourAddress);
console.log("Your token balance:", balance.toString());
- Integrating with Frontend Applications:
- Integrate your token into a front-end application using Web3.js or ethers.js to create a user-friendly interface for interacting with it.
- These JavaScript libraries allow your application to communicate with the Ethereum blockchain and interact with smart contracts.
- Use functions like transfer, balanceOf, and approve provided by your token contract to enable users to perform token transactions directly through your application.
Verify the Contract:
- Go to Etherscan.
- Navigate to the ‘Contract’ tab and enter your deployed contract address.
- Click on ‘Verify and Publish’.
- Provide necessary details such as the compiler version, optimization settings, and contract source code.
- If the verification is successful, your contract will be publicly visible on Etherscan, showing the source code and contact details.
Verifying and interacting with your ERC-20 token contract ensures that it functions correctly on the Ethereum blockchain and builds trust among users and stakeholders.
ethereum token development Solutions
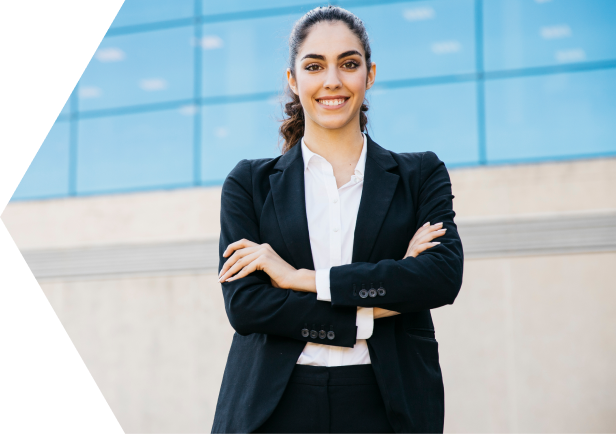
Step 5: Distribute Your Token
1. Transferring Tokens Manually:
- After deploying your ERC-20 token contract, you can manually transfer tokens from your main account (the account that deployed the contract) to other Ethereum addresses.
- Using MetaMask or Similar Wallets:
- Open your Ethereum wallet (e.g., MetaMask) that is connected to the Ethereum mainnet or the desired test network.
- Navigate to the ‘Send’ or ‘Transfer’ section.
- Enter the recipient’s Ethereum address and specify the amount of tokens to transfer.
- Confirm the transaction and wait for it to be included in a block on the Ethereum blockchain.
- The recipient will now see the tokens in their wallet associated with the specified ERC-20 token contract.
2. Creating a Claim or Purchase Function:
- Alternatively, you can create a function within your ERC-20 token contract that allows others to claim or purchase tokens directly.
- Example Implementation:
- Define a function in your token contract that checks certain conditions (such as eligibility or payment) before transferring tokens to the caller’s address.
- Implement access controls or conditions to ensure the function operates securely and as intended.
- Users can interact with this function either through direct contract calls or through a frontend interface that triggers the function using Web3.js or ethers.js.
3. Considerations for Distribution:
- Security: Ensure that any distribution mechanism is secure and protects the token holders’ interests.
- Gas Fees: Transactions on the Ethereum blockchain require gas fees. Consider these fees when distributing tokens, especially if you are transferring tokens to multiple addresses.
- Compliance: Depending on your jurisdiction and the nature of your token distribution, ensure compliance with relevant regulations and legal considerations.
4. Communicate Distribution Details:
- Clearly communicate to recipients how they can access and manage their tokens. Provide instructions on how they can view their token balances using blockchain explorers or wallets.
By effectively distributing your ERC-20 tokens, you enable users and stakeholders to participate in your project, utilize the tokens for their intended purposes, and contribute to the growth of your token ecosystem on the Ethereum blockchain.
Conclusion
Creating an ERC-20 token requires meticulous planning, coding, and rigorous testing to ensure security and functionality. Prioritize testing extensively on Ethereum’s test networks to identify and resolve any potential issues before deploying on the main network. Once deployed, your token integrates seamlessly into Ethereum’s expansive ecosystem.
It becomes tradable on decentralized exchanges, usable within decentralized applications (DApps), and can be held as a digital asset by token holders. Overview of wallets that support ERC-20 tokens, such as MetaMask, Trust Wallet, and MyEtherWallet, ensures accessibility and usability for a wide range of users. By adhering to best practices in smart contract development and deployment, you establish trust among users and stakeholders, fostering the adoption and utilization of your token in the broader blockchain community.