Algorithmic trading has emerged as a revolutionary approach in the cryptocurrency market, enabling traders to execute buy and sell orders at optimal times through a set of predefined rules and algorithms. Given the high volatility and 24/7 nature of crypto markets, algorithmic trading has the potential to maximize profitability while reducing the emotional and human errors involved in manual trading. This guide dives into the foundational elements, strategies, and practical steps needed to implement algorithmic trading on crypto exchanges, tailored for both the novice and the experienced developer.
Introduction
The exponential rise of the cryptocurrency market has brought algorithmic trading to the forefront. With daily trading volumes hitting billions of dollars, crypto markets remain extremely volatile. Algorithmic trading—defined as the use of computer programs to execute trades based on a predefined set of rules—offers the speed and efficiency required to capitalize on market opportunities. This guide will take you from a basic understanding of algorithmic trading through to writing your own bot, with sample code, real-world statistics, and effective trading strategies.
Build Your Secure Crypto Exchange Today!
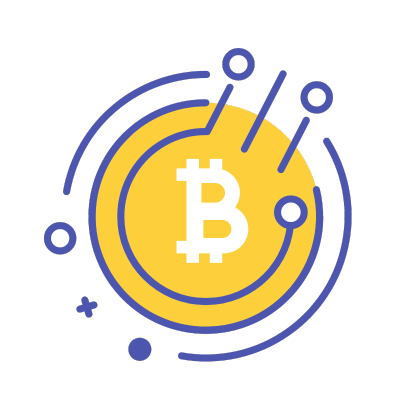
What is Algorithmic Trading?
Algorithmic trading involves creating and implementing a trading strategy using a coded algorithm that automatically makes buy and sell decisions on behalf of a user. This removes emotional decisions and ensures consistent execution.
Key Components of Algorithmic Trading
- Data Sources: Access to accurate, real-time market data.
- Trading Strategy: The core rules the algorithm follows.
- Execution: Placing buy/sell orders on crypto exchanges.
- Backtesting: Testing the algorithm with historical data to evaluate performance.
- Risk Management: Stop-loss and take-profit levels for risk reduction.
Benefits of Algorithmic Trading in Cryptocurrency Markets
- 24/7 Market Coverage: Crypto markets operate around the clock, which algorithmic trading can accommodate.
- Speed: Algorithms can analyze and execute trades within milliseconds.
- Precision: Predefined criteria reduce human error.
- Backtesting Ability: Allows users to test strategies on historical data before live execution.
According to recent studies, over 60% of all crypto trades are now algorithmic due to the benefits in efficiency and risk management that algorithms provide.
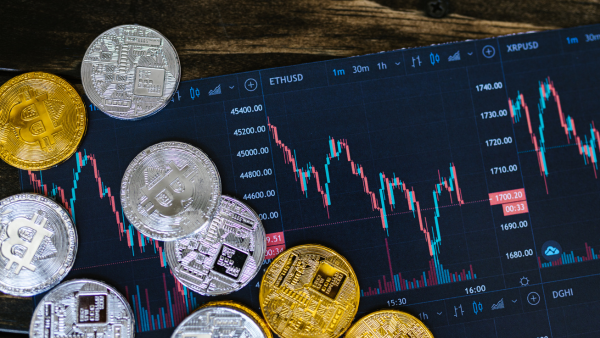
Step-by-Step Guide to Building a Crypto Trading Algorithm
1. Define Your Trading Strategy
- Start with a clear strategy—whether that’s a market-making, trend-following, or arbitrage strategy.
- Set key parameters, such as entry/exit conditions, risk tolerance, and asset allocation.
2. Select a Crypto Exchange with an API
- Exchanges like Binance, Coinbase Pro, and Kraken provide robust APIs for trading.
- Security Note: Use an API key with permissions limited only to the required actions (avoid withdrawal permissions).
3. Collect and Analyze Data
- Historical Data: Essential for backtesting. Most exchanges offer data retrieval via API.
- Real-Time Data: Access real-time market data to enable live trading decisions.
4. Develop the Algorithm
- Select a programming language (Python is popular for its data-handling libraries).
- Structure your code to handle data collection, analysis, and order execution.
5. Backtest Your Strategy
- Run your algorithm on historical data to check its effectiveness.
- Evaluate metrics like Sharpe Ratio and Maximum Drawdown.
6. Deploy and Monitor the Algorithm
- Deploy on a cloud server for 24/7 operation (AWS or Google Cloud are suitable options).
- Continuously monitor performance and make adjustments as needed.
7. Implement Risk Management
- Stop-loss and take-profit measures should be integral parts of the algorithm.
- Adjust the algorithm based on real-time volatility and performance.
Start Your Centralized Exchange Today!
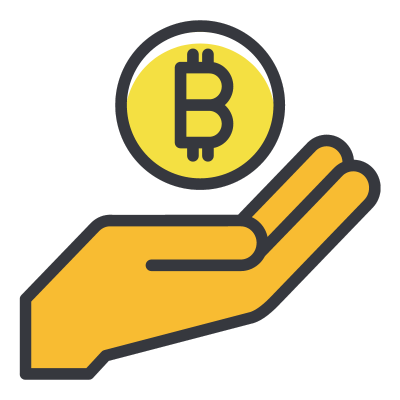
Popular Algorithmic Trading Strategies for Crypto
1. Mean Reversion
- Assumes that asset prices will revert to the mean over time.
- Example: If Bitcoin falls by 10% from a historical mean, the bot may buy, expecting the price to bounce back.
2. Arbitrage
- Capitalizes on price differences between exchanges.
- Real-World Example: If Bitcoin is trading at $30,000 on Binance and $30,100 on Kraken, a bot could buy on Binance and sell on Kraken for an instant profit.
3. Momentum Trading
- Buys assets trending upward and sells those trending downward.
- Indicators Used: Moving Average Convergence Divergence (MACD), Relative Strength Index (RSI).
4. Market Making
- Places buy and sell orders on both sides of the order book to capture the spread.
- Ideal For: Low-volatility trading pairs.
Implementing a Basic Trading Bot in Python
Below is a simplified Python script to illustrate algorithmic trading on Binance using the ccxt library.
Requirements
Install ccxt:
pip install ccxt
import ccxt
import time
# Initialize Binance API
api_key = ‘YOUR_API_KEY’
api_secret = ‘YOUR_API_SECRET’
binance = ccxt.binance({
‘apiKey’: api_key,
‘secret’: api_secret,
})
# Function to get the current price of BTC/USDT
def fetch_price(symbol=’BTC/USDT’):
ticker = binance.fetch_ticker(symbol)
return ticker[‘last’]
# Simple Moving Average (SMA) Strategy
def sma_strategy():
short_window = 5
long_window = 20
prices = []
# Loop to continuously update prices and execute orders
while True:
price = fetch_price()
prices.append(price)
# Check if enough data points for SMA calculation
if len(prices) >= long_window:
short_sma = sum(prices[-short_window:]) / short_window
long_sma = sum(prices[-long_window:]) / long_window
# Buy if short SMA is above long SMA
if short_sma > long_sma:
binance.create_market_buy_order(‘BTC/USDT’, 0.001)
# Sell if short SMA is below long SMA
elif short_sma < long_sma:
binance.create_market_sell_order(‘BTC/USDT’, 0.001)
# Run every minute
time.sleep(60)
# Run the SMA strategy
sma_strategy()
Explanation of Key Components
- fetch_price: Retrieves the latest price.
- sma_strategy: Implements a basic moving average strategy, buying when the short SMA is above the long SMA and selling otherwise.
Note: This is a basic illustration. Always perform extensive testing and incorporate error handling in a production environment.
Tips for Enhancing Algorithmic Trading Performance
- Optimize Parameters: Test and fine-tune variables like SMA lengths, stop-loss percentages, and order sizes.
- Diversify Across Exchanges: Implement arbitrage across multiple exchanges to capitalize on price differences.
- Use Leverage Wisely: Leveraged trades can amplify profits but also increase risk.
- Set Alerts and Notifications: Integrate notifications (e.g., via Slack or email) to monitor bot performance in real time.
Statistics: A study by AlgoTrader found that up to 92% of profitable trades resulted from diversified strategies across exchanges rather than single-exchange trading.
Conclusion
Algorithmic trading in crypto markets provides a highly efficient, emotionless method for executing trades. However, to succeed, it requires a deep understanding of strategy, risk management, and constant monitoring. By following the steps and using the sample code provided, traders can build a foundation for implementing algorithmic trading on crypto exchanges, with room for further customization and sophistication.