React has become one of the most popular libraries for building user interfaces, and it’s easy to see why. With its component-based architecture, virtual DOM, and fast rendering, React allows developers to build dynamic and interactive web applications with ease. But what if you could make React development even more robust and scalable? Enter TypeScript—a powerful, statically typed superset of JavaScript that can catch errors early and improve the maintainability of your code.
In this blog post, we’ll walk through the benefits of using TypeScript with React, how to set it up in a React project, and some best practices to help you get started. Whether you’re a React JS developer looking to improve your workflow or part of a React JS development company exploring TypeScript for React web development, this guide will help you navigate the process.
What is TypeScript and Why Use It with React?
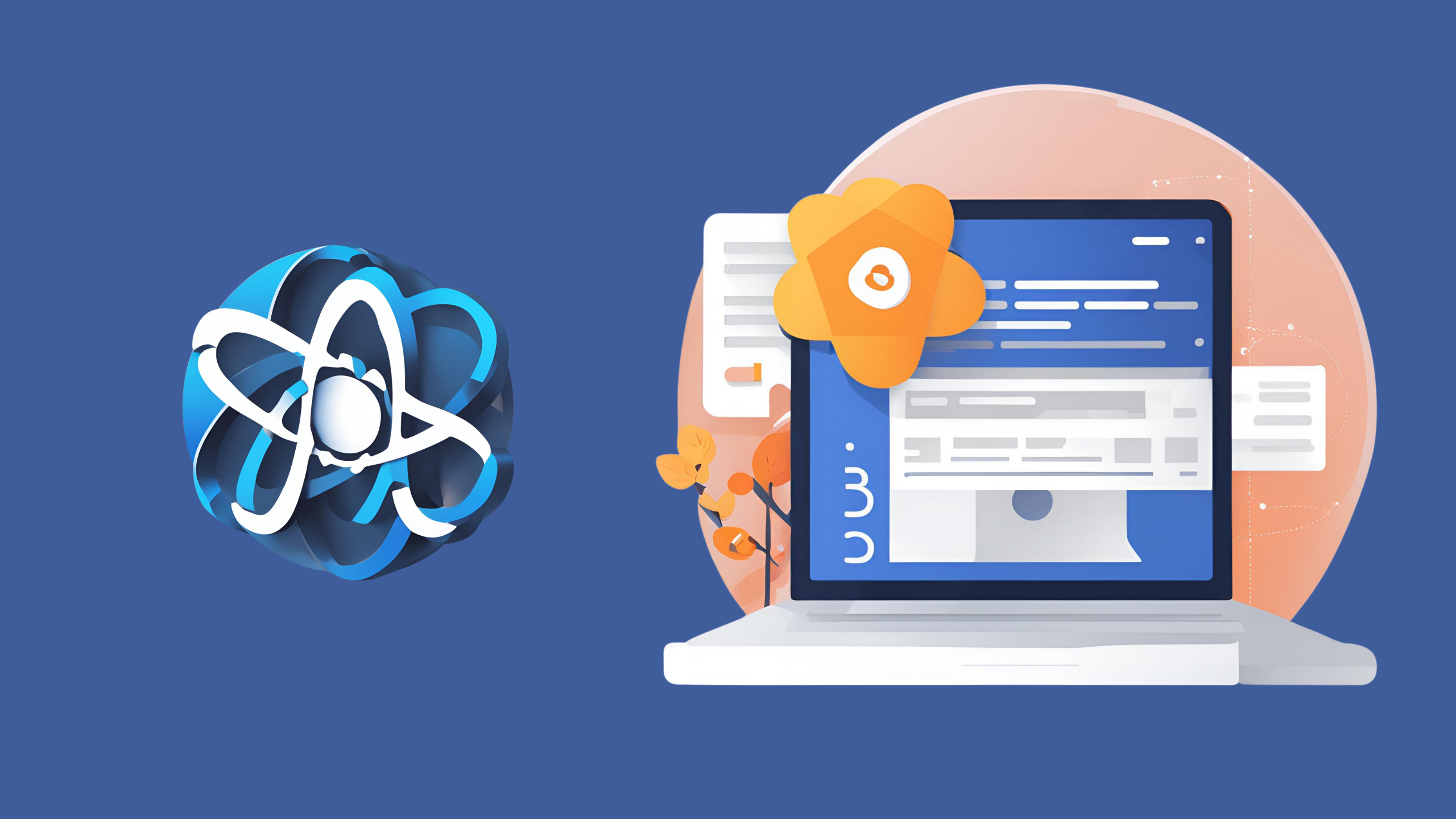
What is TypeScript?
TypeScript is a superset of JavaScript that adds static typing to the language. This means you can define types for your variables, function parameters, return values, and objects. TypeScript then compiles to plain JavaScript, which can be run in any browser or JavaScript environment.
For example, in JavaScript, you might write:
javascript
Copy code
let message = “Hello, World!”;
In TypeScript, you can specify the type of the message variable:
typescript
Copy code
let message: string = “Hello, World!”;
While this may seem like an extra step, it helps catch type-related errors before they occur in production, improving the reliability of your codebase.
Launch High-Performance ReactJS Applications!
Work with SDLC CORP to transform your ideas into stunning digital products.
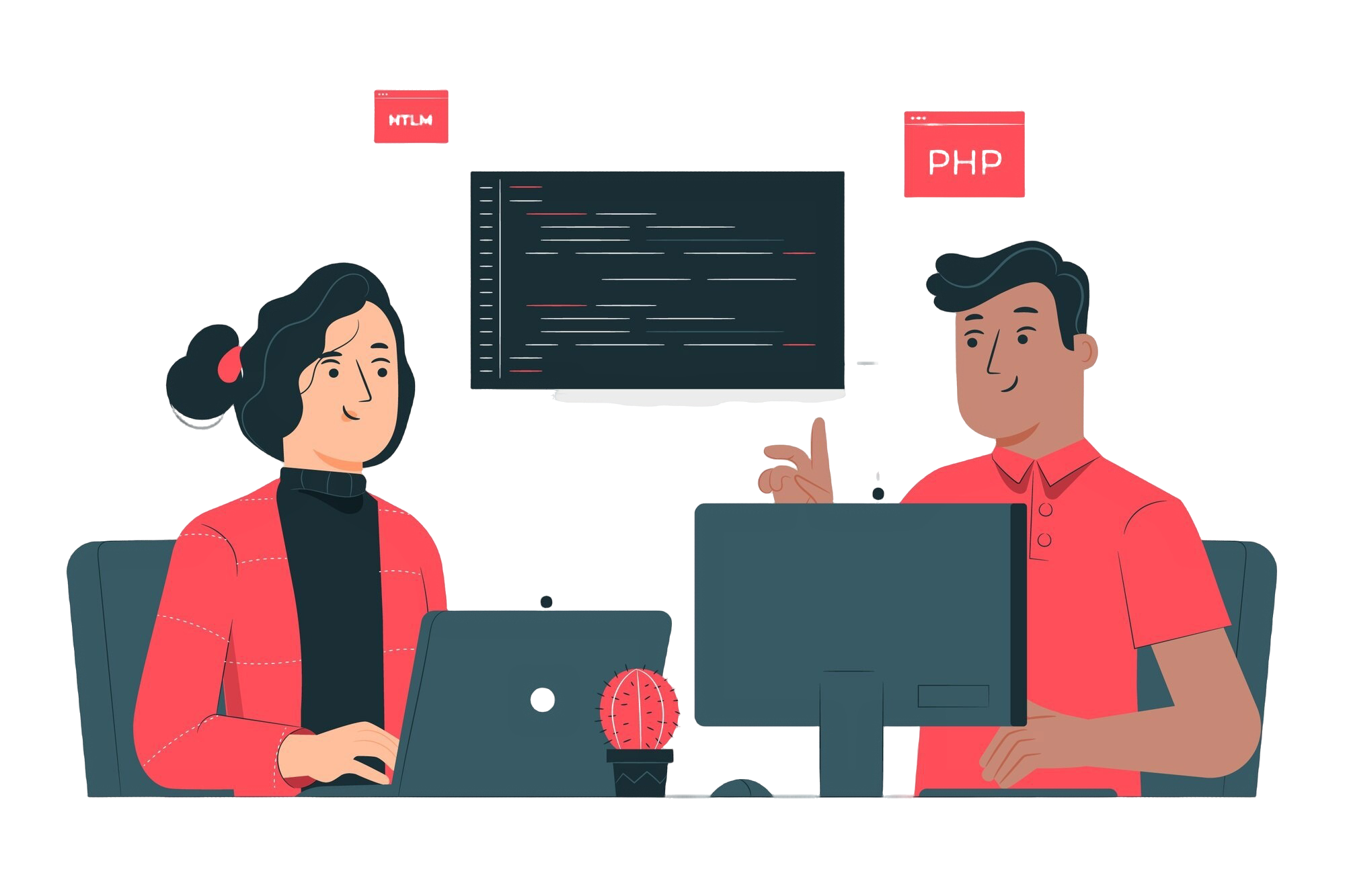
Why Use TypeScript with React?
Integrating TypeScript into your React development can provide numerous benefits:
- Early Error Detection: TypeScript’s static typing helps catch potential errors during development rather than at runtime. This leads to fewer bugs and better-quality code.
- Improved Code Quality and Maintainability: With type annotations, your code becomes easier to understand and maintain. As a result, it’s easier to collaborate with other developers, especially in larger teams.
- Better IntelliSense and Autocompletion: TypeScript provides improved autocompletion and code suggestions in IDEs like Visual Studio Code. This helps speed up development and reduces the chances of making mistakes.
- Scalability: As your application grows, TypeScript helps you scale by ensuring that your types remain consistent, reducing the risk of introducing breaking changes.
- Strong Integration with React: TypeScript is designed to work seamlessly with React. TypeScript supports React’s JSX syntax and helps define types for props, state, and event handlers, which is crucial in large-scale applications.
If you’re working on a React mobile app, React app development, or React website development, TypeScript can significantly improve the robustness of your app by providing type safety and clearer API contracts.
Setting Up TypeScript with React
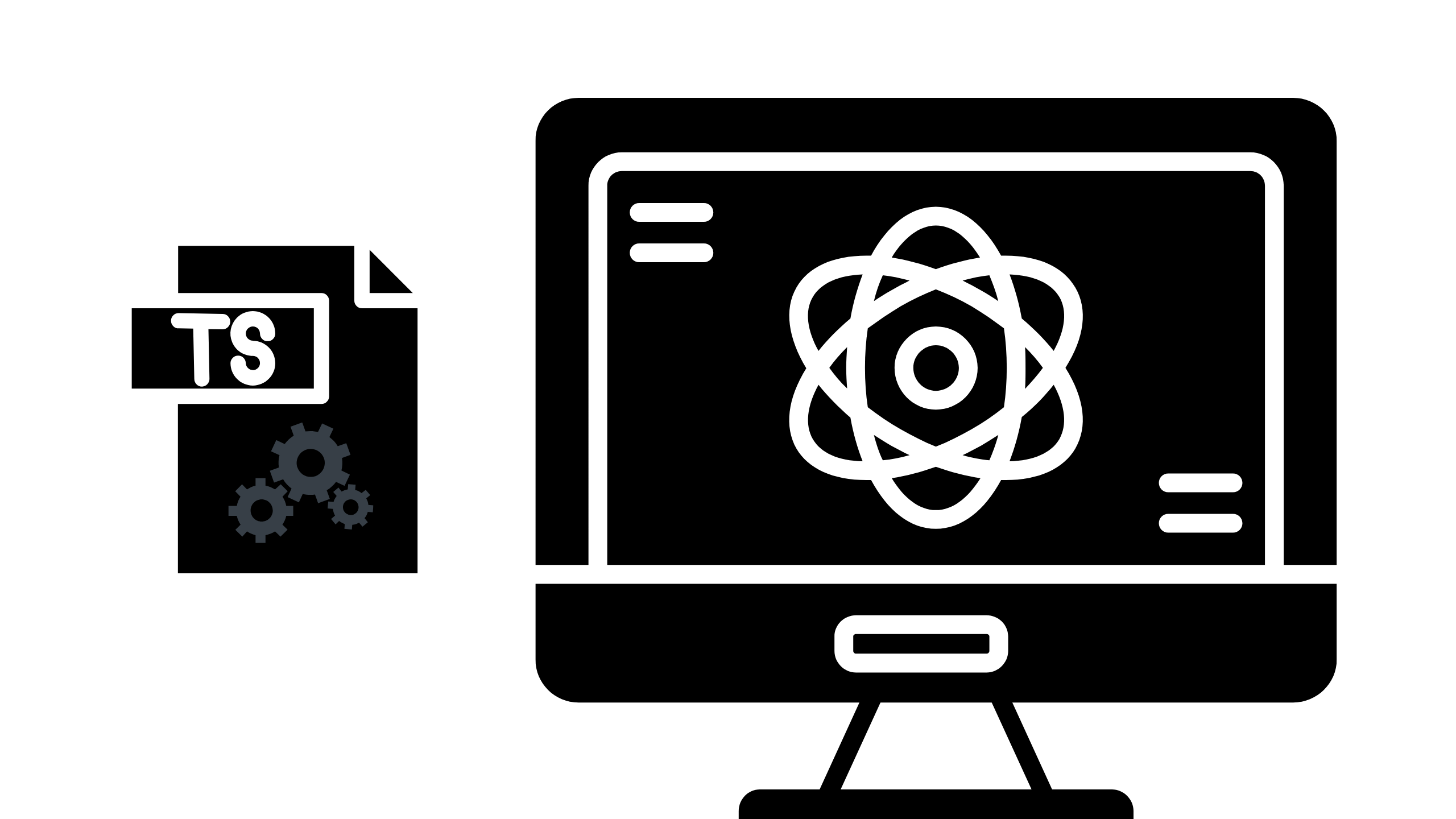
Setting up TypeScript with React is easier than ever, thanks to the official support from React and the React community. Let’s go through the steps to get started.
Step 1: Create a New React Project with TypeScript
The easiest way to start using TypeScript with React is to use the create-react-app tool, which provides a template for TypeScript.
- Open a terminal and run the following command:
bash
npx create-react-app my-app –template typescript
This will create a new React project with TypeScript configured out of the box. If you already have an existing React web development project and want to add TypeScript, follow the instructions below.
Step 2: Install TypeScript in an Existing React Project
If you already have a React project and want to add TypeScript, you can install the necessary dependencies by running:
bash
npm install –save typescript @types/react @types/react-dom
This will install TypeScript along with type definitions for React and React DOM.
Step 3: Configure TypeScript
Once TypeScript is installed, the next step is to set up the TypeScript configuration file. Create a tsconfig.json file in the root of your project with the following content:
json
Copy code
{
“compilerOptions”: {
“target”: “es5”,
“lib”: [“dom”, “es2015”],
“allowJs”: true,
“jsx”: “react”,
“strict”: true,
“esModuleInterop”: true,
“skipLibCheck”: true,
“forceConsistentCasingInFileNames”: true
},
“include”: [“src”]
}
This configuration ensures that TypeScript compiles your JSX code correctly and enforces strict typing throughout the project.
Step 4: Rename Files to .tsx
In React projects, any file that contains JSX should have the .tsx extension instead of .js. For files that don’t contain JSX, you can use the .ts extension.
Rename your App.js file to App.tsx to let TypeScript know that this file contains JSX syntax.
Working with Types in React Components
Once TypeScript is set up, it’s time to start typing your React components. TypeScript allows you to define types for props, state, and events in your React components.
Typing Props
Let’s say you have a component that accepts a name prop. You can define the type for this prop like so:
tsx
Copy code
import React from ‘react’;
interface GreetingProps {
name: string;
}
const Greeting: React.FC<GreetingProps> = ({ name }) => {
return <h1>Hello, {name}!</h1>;
};
export default Greeting;
In this example:
- We define an interface GreetingProps that specifies the type of the name prop.
- The component is typed as React.FC<GreetingProps>, which is shorthand for React.FunctionComponent<GreetingProps>. This tells TypeScript that Greeting is a functional component that accepts props of type GreetingProps.
Typing State
In TypeScript, typing state in React helps ensure that the data stored in your component’s state is used consistently and safely throughout your app. Whether you’re working with functional components or class components, TypeScript provides powerful type-checking features to avoid bugs related to state management.
In functional components, you can type the state using the useState
hook. For example, if you’re managing a numeric value, you would define the type like this:
const [count, setCount] = useState<number>(0);
Here, useState<number>(0)
ensures the state will always be a number.
In class components, you define an interface to type the state and pass it as a generic to the Component
class. For example:
interface CounterState { count: number;
}class Counter extends React.Component<{}, CounterState> { state: CounterState = { count: 0 }; // Rest of the component}
By typing state, you reduce the risk of type errors and make your codebase more maintainable and readable.
Build Fast, Scalable ReactJS Apps Today!
Partner with SDLC CORP for top-notch ReactJS development solutions.
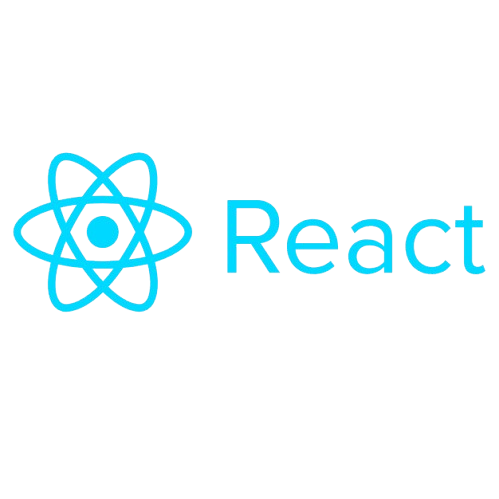
Typing Events
In TypeScript, typing events in React ensures that your event handlers are correctly typed and prevent runtime errors by providing type safety during development. When working with events in functional components, you can use the specific event types from React
(such as React.MouseEvent
, React.ChangeEvent
, etc.) to type your event handlers.
For example, typing a click event in a functional component would look like this:
const handleClick = (event: React.MouseEvent<HTMLButtonElement>) => {
console.log('Button clicked!');
};
In this case, React.MouseEvent<HTMLButtonElement>
ensures that event
is typed as a mouse event specifically for a button element.
For form elements, like handling input changes, you might type it like this:
const handleChange = (event: React.ChangeEvent<HTMLInputElement>) => {
setValue(event.target.value);
};
This ensures that event.target
is properly typed as an HTMLInputElement
, allowing access to properties like value
safely. By typing events, you prevent mistakes, making the code more robust and developer-friendly
Best Practices for Using TypeScript with React
When using TypeScript with React, here are some best practices that can help you write cleaner and more maintainable code:
- Use TypeScript Interfaces and Types: Use interfaces and types to define the shape of props, state, and other objects. This makes your code more predictable and easier to refactor.
- Enable Strict Mode: Set “strict”: true in your tsconfig.json file. This enables a range of type-checking options that help catch potential issues early.
- Type Your Event Handlers: Always type your event handlers properly to avoid runtime errors and take full advantage of TypeScript’s type checking.
- Use React.FC for Functional Components: React.FC (or React.FunctionComponent) provides implicit typing for children props and other React-specific features, reducing boilerplate code.
- Avoid any Type: While TypeScript allows you to use the any type, avoid it as much as possible, as it defeats the purpose of using TypeScript. Instead, try to define specific types for variables and props.
Accelerate Your Business with ReactJS!
Choose SDLC CORP for dynamic, responsive, and scalable ReactJS development.
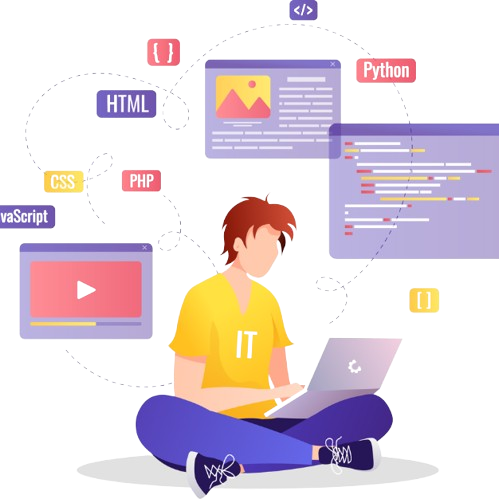
Conclusion
Incorporating TypeScript into your React development process can significantly enhance your development workflow. By providing static typing, TypeScript helps catch errors early, improves code maintainability, and makes your codebase more scalable. Whether you’re working on a React mobile app, React app development, or React website development, using TypeScript can lead to better quality and more reliable applications.
If you’re considering React JS development services or need help with React software, working with a skilled React JS development company can help you seamlessly integrate TypeScript into your project, ensuring high-quality and maintainable code that scales well in the long term.
SDLC CORP ReactJS Development Services Overview
SDLC CORP offers top-notch React website development services to create dynamic, scalable, and user-friendly web applications. Our expert team leverages ReactJS to deliver high-performance solutions tailored to meet your business needs, ensuring a seamless user experience and robust functionality. Partner with us to build innovative, modern websites that drive growth and engagement.