Dashboards are an essential feature for many applications today. Whether you are building a data-driven application for analytics, performance tracking, or simply displaying real-time information, dashboards help to visualize data in an interactive and user-friendly way.
In this guide, we’ll show you how to create a dynamic and responsive dashboard using React and Chart.js. React JS development has become a popular choice for building modern web applications due to its efficiency, flexibility, and scalability. By combining React with Chart.js, a powerful and flexible charting library, you can create stunning dashboards that are both functional and visually appealing.
Let’s walk through the steps to build a basic dashboard, focusing on how to integrate Chart.js with React for data visualization.
What is React and Chart.js?
Before diving into the tutorial, let’s briefly explore what React and Chart.js offer.
React:
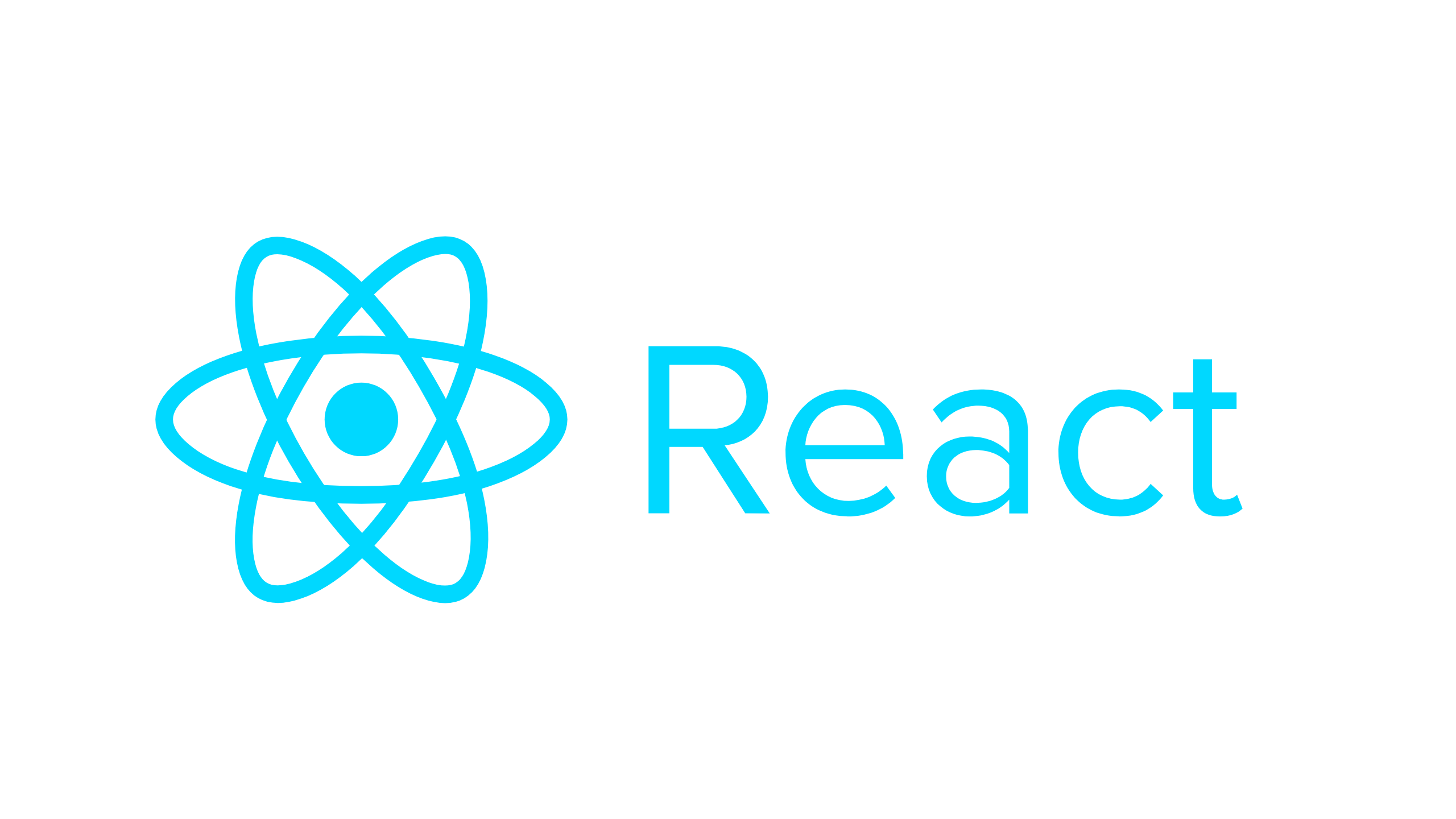
React is a JavaScript library for building user interfaces, developed by Facebook. It allows developers to build interactive UIs with reusable components. React web development offers a declarative approach to developing UIs, making it easy to manage dynamic content and state changes. Its component-based architecture also makes it ideal for building large applications like dashboards
Chart.js:
Chart.js is a popular open-source charting library that provides simple yet flexible ways to display various types of charts. It supports different types of charts such as line, bar, radar, and pie charts, and it’s widely used in React app development to visualize data dynamically.
Why Use React and Chart.js for Building Dashboards?
Building dashboards with React JS development services is a natural choice due to its performance and ease of use. By combining Chart.js with React, you get:
- Scalable Components: React allows you to break down your dashboard into smaller, reusable components, making it easier to maintain and scale over time.
- Responsive and Interactive: React’s ability to update and re-render components efficiently, coupled with Chart.js’s interactivity, makes it possible to create responsive, real-time dashboards.
- Ease of Integration: Chart.js integrates seamlessly with React, making it simple to create interactive charts that respond to state changes.
Launch High-Performance ReactJS Applications!
Work with SDLC CORP to transform your ideas into stunning digital products.
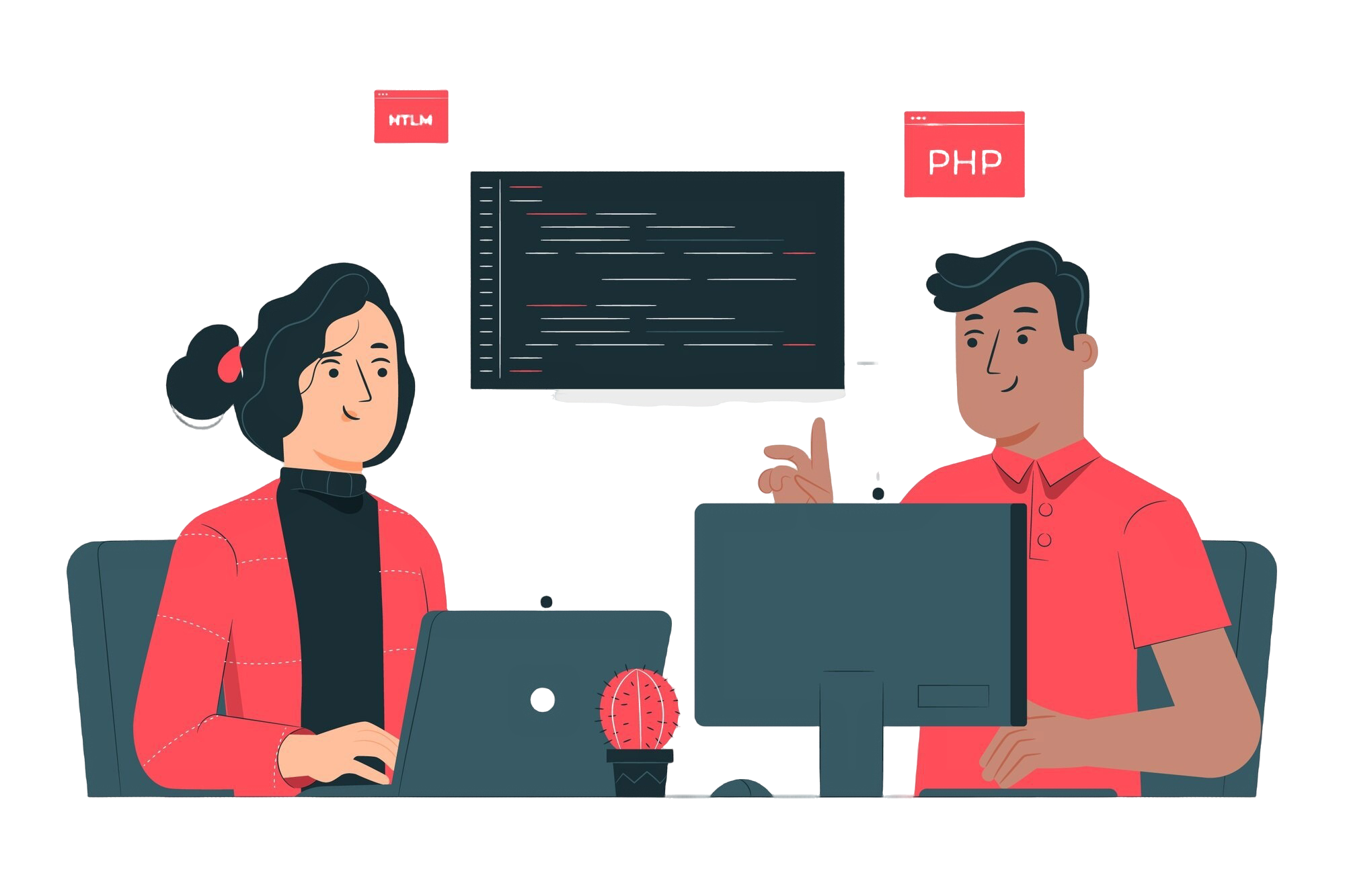
Steps to Build a Dashboard with React and Chart.js
Now, let’s go step by step through building a dashboard with React and Chart.js. We’ll focus on creating a simple dashboard that displays a few charts.
Step 1: Set Up Your React App
If you don’t have a React app set up yet, you can quickly create one using Create React App:
npx create-react-app react-dashboard
cd react-dashboard
This will create a new directory called react-dashboard
with a basic React project structure.
Step 2: Install Chart.js and React-Chartjs-2
To integrate Chart.js with React, we need to install both Chart.js and react–chartjs-2, a wrapper library that makes it easier to use Chart.js with React.
Run the following command to install the dependencies:
npm install chart.js react-chartjs-2
Step 3: Create a Chart Component
Now that you’ve set up the necessary packages, let’s create a simple line chart to display some data.
Create a new file under the
src
directory calledChartComponent.js
.Import the required modules and set up the chart:
import React from 'react';
import { Line } from 'react-chartjs-2';
import { Chart as ChartJS, CategoryScale, LinearScale, LineElement, PointElement, Title, Tooltip, Legend } from 'chart.js';
// Register the necessary chart components
ChartJS.register(CategoryScale, LinearScale, LineElement, PointElement, Title, Tooltip, Legend);
const ChartComponent = ({ data, options }) => {
return (
<div>
<h2>Sales Data</h2>
<Line data={data} options={options} />
</div>
);
};
export default ChartComponent;
In this code:
- We import the Line component from
react-chartjs-2
to render the line chart. - We also register the necessary Chart.js components to render the chart.
Build Fast, Scalable ReactJS Apps Today!
Partner with SDLC CORP for top-notch ReactJS development solutions.
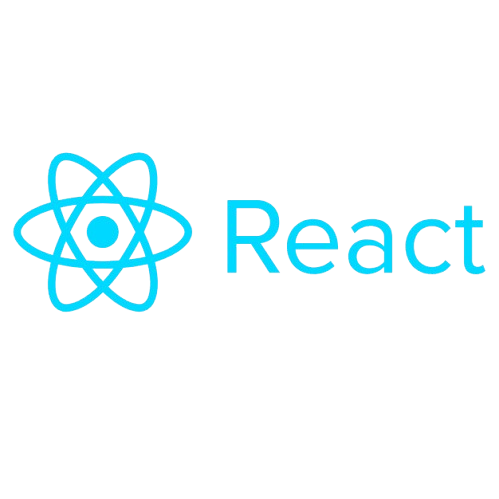
Step 4: Prepare Chart Data and Options
Before using the ChartComponent
, we need to define some chart data and chart options. You can modify the data dynamically based on your use case, but for now, we’ll use static data.
Here’s an example of the data and options:
const data = { labels: ['January', 'February', 'March', 'April', 'May', 'June'], datasets: [ { label: 'Sales', data: [30, 20, 40, 60, 80, 90], fill: false, borderColor: 'rgb(75, 192, 192)', tension: 0.1, }, ],
};const options = { responsive: true, plugins: { title: { display: true, text: 'Monthly Sales', }, },
};
- labels represent the X-axis values (e.g., months).
- datasets hold the actual data points to be plotted, including the color and style of the line
Step 5: Build the Dashboard Layout
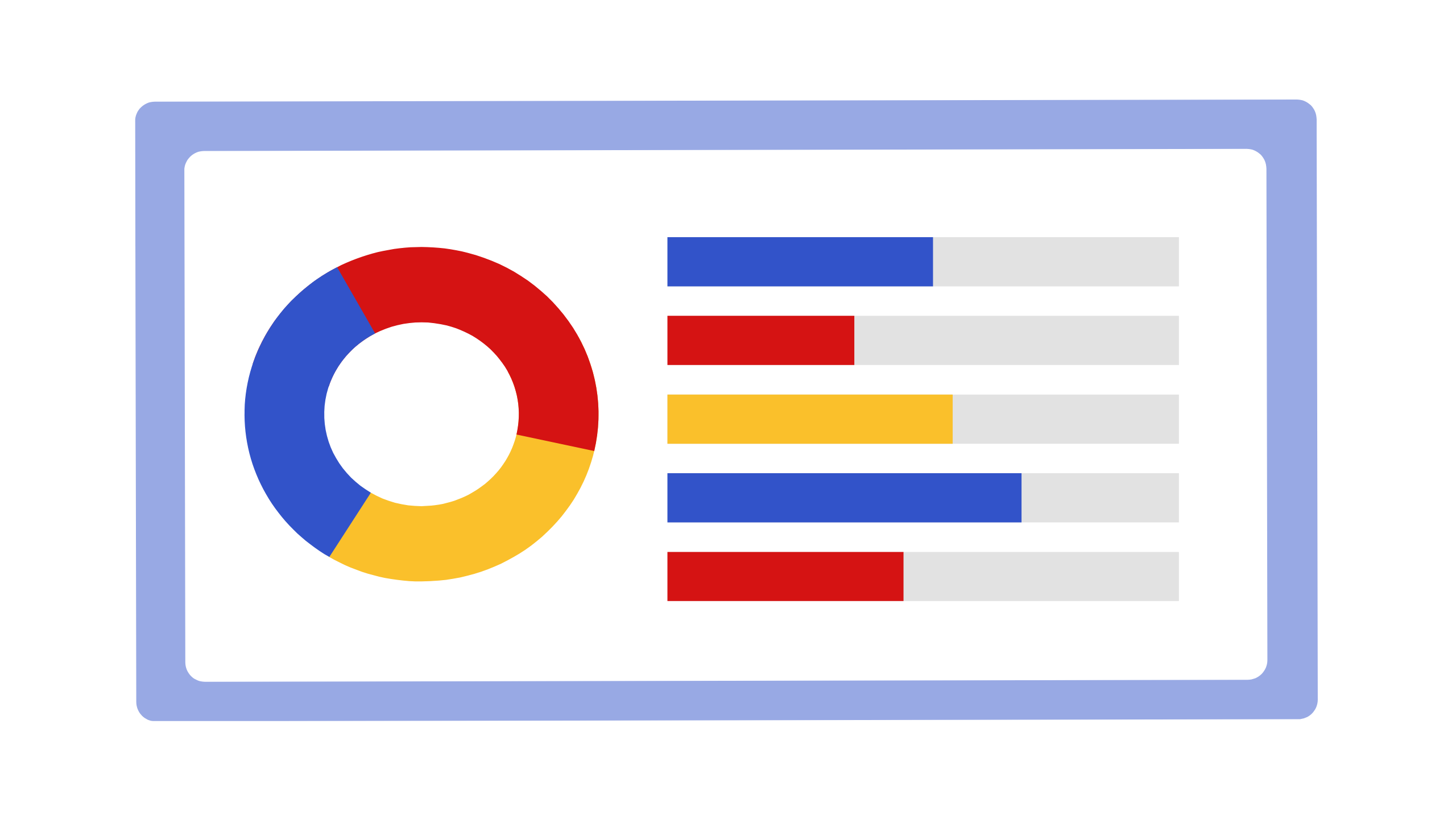
Now, let’s build the dashboard layout and include our chart component. You can use any layout you like, but a basic grid layout will work for this example.
Create a new file called Dashboard.js
:
import React from 'react';
import ChartComponent from './ChartComponent';
const Dashboard = () => {
const data = {
labels: [‘January’, ‘February’, ‘March’, ‘April’, ‘May’, ‘June’],
datasets: [
{
label: ‘Sales’,
data: [30, 20, 40, 60, 80, 90],
fill: false,
borderColor: ‘rgb(75, 192, 192)’,
tension: 0.1,
},
],
};
const options = {
responsive: true,
plugins: {
title: {
display: true,
text: ‘Monthly Sales’,
},
},
};
return (
<div className=“dashboard”>
<h1>React Dashboard</h1>
<div className=“charts”>
<ChartComponent data={data} options={options} />
</div>
</div>
);
};
export default Dashboard;
In this file:
- We import the
ChartComponent
and pass the data and options as props. - We create a simple layout for the dashboard with a title and a div to hold the chart.
Step 6: Add Styling
To make your dashboard look more polished, you can add some basic styles in the App.css
file. Here’s an example of a simple layout:
.dashboard { padding: 20px;
}.charts { display: flex; justify-content: space-between; gap: 20px;
}
This CSS will arrange the charts horizontally and give them some spacing.
Step 7: Run the Application
Now, you’re ready to see your dashboard in action. To run your app, execute the following command:
npm start
Visit http://localhost:3000
in your browser, and you should see a simple dashboard with a line chart displaying sales data.
Extending the Dashboard
Now that you have a basic dashboard up and running, there are many ways to extend it:
- Add More Charts: You can add different types of charts (bar charts, pie charts, radar charts, etc.) to your dashboard to represent various data points.
- Dynamic Data: Integrate your dashboard with a backend API to fetch real-time data, making your charts dynamic and always up-to-date.
- Responsive Design: Use CSS frameworks like Bootstrap or Material-UI to make your dashboard responsive and mobile-friendly.
- Interactivity: Chart.js supports features like tooltips, animations, and hover effects that can make your dashboard more interactive.
Accelerate Your Business with ReactJS!
Choose SDLC CORP for dynamic, responsive, and scalable ReactJS development.
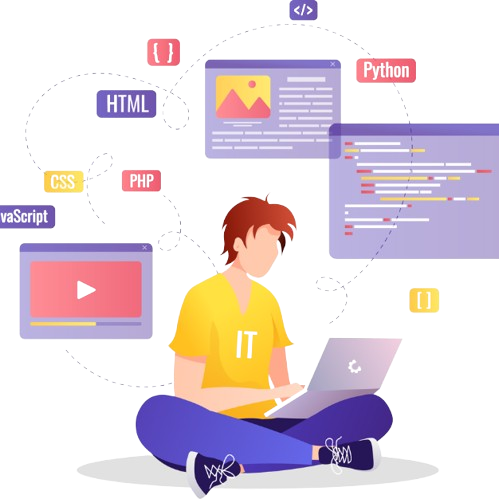
Conclusion
Building a dashboard with React and Chart.js is straightforward, and with these tools, you can easily create visually appealing and interactive data visualizations. By following this guide, you’ve learned how to integrate Chart.js into a React project, set up a basic line chart, and structure your dashboard layout.
If you’re working with a React JS development company or exploring React development services, this combination of React web development and Chart.js can help you create powerful, real-time dashboards. Whether you’re building a React mobile app, React app development, or React website development, this approach will allow you to build rich, data-driven applications with ease.
SDLC CORP ReactJS Development Services Overview
SDLC CORP offers top-notch React website development services to create dynamic, scalable, and user-friendly web applications. Our expert team leverages ReactJS to deliver high-performance solutions tailored to meet your business needs, ensuring a seamless user experience and robust functionality. Partner with us to build innovative, modern websites that drive growth and engagement.