Custom hooks are a powerful feature in React. They allow you to extract logic from components into reusable functions. Mastering them is essential for any developer. This guide explains everything you need to know about custom hooks. Whether you are a beginner or seasoned React JS development company professional, this guide has something for you.
What Are Custom Hooks in React?
Custom hooks are JavaScript functions that start with the word use. They follow the rules of hooks and allow developers to reuse stateful logic. Hooks like useState and useEffect are examples of built-in React hooks. However, you can build your own to streamline repetitive code. Creating custom hooks ensures cleaner, more modular components. It simplifies the development process and improves maintainability. Companies offering React JS development services often use custom hooks to improve efficiency. Understanding this concept can help you write better code and improve app performance.
Custom hooks are not complex to write. You simply wrap reusable logic into a function. Then, you call that function wherever needed. For instance, you may create a hook to manage forms, fetch data, or handle authentication. This approach reduces duplication and keeps components simple. Many React JS development experts rely on custom hooks to maintain clean architecture.
ReactJs Development Company
Top ReactJS App and web Development company Services
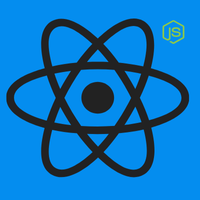
Why Use Custom Hooks in React?
Custom hooks offer significant benefits. They reduce code duplication, improve reusability, and simplify testing. By writing custom hooks, you can encapsulate complex logic into small, manageable functions. This improves code readability and maintainability. Custom hooks enable you to share logic between multiple components. If you’re working in a team or a React JS developer role, this can drastically improve productivity.
Another advantage is separation of concerns. Instead of keeping all the logic in components, you move reusable code into custom hooks. This allows components to focus on rendering UI. Custom hooks act as utility functions that handle specific tasks. Additionally, custom hooks make codebase refactoring easier. They provide an organized structure to complex projects. Businesses involved in React web development find this approach beneficial for building scalable applications.
For example, fetching data from an API often involves repetitive code. With a custom hook like useFetch, you can centralize this logic. You can then reuse it across components without rewriting it. This reduces bugs and improves performance.
Look also this: Boosting React Performance with Code Splitting and Lazy Loading
How to Create a Custom Hook in React
Creating a custom hook is straightforward. Follow these simple steps to build one:
- Identify Reusable Logic: Find code that is duplicated across components.
- Wrap Logic in a Function: Create a function and use useState or useEffect if needed.
- Return Values or Functions: The custom hook should return values or methods for components to use.
For example, let’s create a useFetch hook to fetch data from an API. Start with the function:
import { useState, useEffect } from ‘react’;
function useFetch(url) {
const [data, setData] = useState(null);
const [error, setError] = useState(null);
const [loading, setLoading] = useState(true);
useEffect(() => {
fetch(url)
.then((response) => response.json())
.then((data) => {
setData(data);
setLoading(false);
})
.catch((error) => {
setError(error);
setLoading(false);
});
}, [url]);
return { data, error, loading };
}
export default useFetch;
This hook can be used in components to fetch data. For example:
const { data, loading, error } = useFetch(‘https://api.example.com/data’);
Many React development teams create similar hooks for various tasks like authentication, input management, and subscriptions.
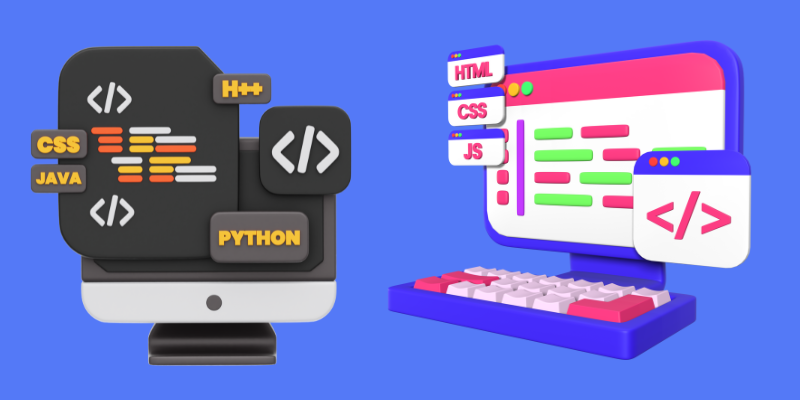
Best Practices for Writing Custom Hooks
When writing custom hooks, follow these best practices to ensure efficiency:
- Name Hooks Properly: Always start with use to indicate a hook. For example, useAuth or useInput.
- Keep Hooks Small: Custom hooks should focus on one responsibility. Avoid bloated logic.
- Avoid Breaking Rules of Hooks: Ensure your custom hook calls other hooks in the correct order.
- Parameterize Hooks: Pass arguments to make hooks reusable in different scenarios.
- Return Useful Data: Make sure hooks return clear, usable values for components.
Following these practices ensures your hooks are clean and reusable. Teams delivering React development services often rely on these best practices to write scalable solutions. Writing hooks with clarity helps the whole team understand and use them effectively.
Hire ReactJs Developer
ReactJs Development services offer end-to-end solutions for creating engaging, high-quality ReactJs Development platforms.
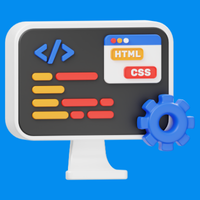
Custom Hooks for Mobile Development
If you’re working on React mobile app development, custom hooks can simplify tasks. Mobile apps often require features like geolocation, push notifications, and offline data. Custom hooks centralize these functions into reusable code. For example, you can create a useGeoLocation hook to fetch location data:
function useGeoLocation() {
const [location, setLocation] = useState(null);
useEffect(() => {
navigator.geolocation.getCurrentPosition((position) => {
setLocation(position.coords);
});
}, []);
return location;
}
With hooks like these, you simplify mobile app development and improve efficiency. Companies offering React app development solutions often use similar hooks to handle platform-specific features.
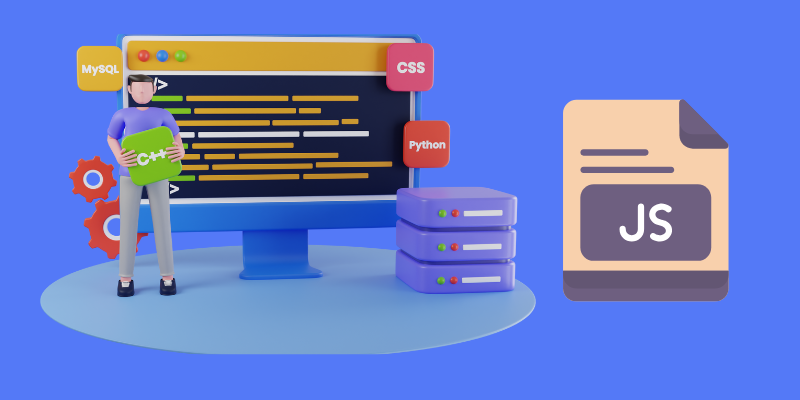
Benefits of Using Custom Hooks in Website Development
Custom hooks are equally beneficial for React website development projects. Websites require features like dynamic data, search, and animations. Instead of writing repetitive logic in components, developers use custom hooks to manage these features efficiently.
For example, implementing a search filter can be simplified with a hook like useSearch. It filters data based on a query and returns updated results. Custom hooks save time and keep your components clean. Teams specializing in React development services use hooks extensively for such tasks.
Moreover, custom hooks improve testing. Since they are pure functions, you can easily write unit tests for them. This enhances code reliability and ensures smooth development processes.
Conclusion
Mastering custom hooks is essential for React developers. They simplify logic, reduce code duplication, and improve app performance. Whether you are working on web, software, or React JS development services, custom hooks can transform your workflow. By encapsulating complex logic into reusable functions, you make your code cleaner and more maintainable.
Custom hooks play a significant role in modern React web development and mobile app solutions. By following best practices and focusing on reusability, you can build scalable applications. Partner with experienced teams for efficient development. Implementing custom hooks ensures you deliver high-quality applications while enhancing productivity and performance.
Boost Profits with Expert ReactJs Development Company Services
Top ReactJS App and web Development company Services
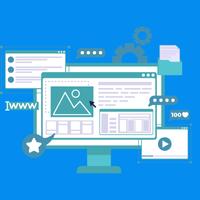
SDLC CORP ReactJs Development Services Overview
SDLC Corp offers top-notch ReactJS development services, delivering fast, scalable, and interactive web applications tailored to your business needs. Our expert developers leverage ReactJS’s component-based architecture to build dynamic user interfaces, ensuring seamless performance, flexibility, and superior user experiences for businesses of all sizes. Partner with us for innovative React solutions.