Introduction
In today’s fast-paced world of React web development, mastering React Hooks has become a critical skill for any developer looking to build dynamic and efficient applications. Hooks, introduced in React 16.8, revolutionized the way developers write components by enabling functional components to use state and lifecycle features. Among these, useState and useEffect are the most widely used hooks and form the foundation of any React developer’s toolkit. Whether you’re a seasoned React JS developer or just diving into the world of React, understanding these hooks will elevate your skills.
If you’re working with a React JS development company or utilizing React development services, leveraging useState and useEffect can significantly enhance the performance and maintainability of your projects. Let’s dive deeper into these essential hooks to understand how they work and why they’re indispensable for modern React software development.
What is useState?
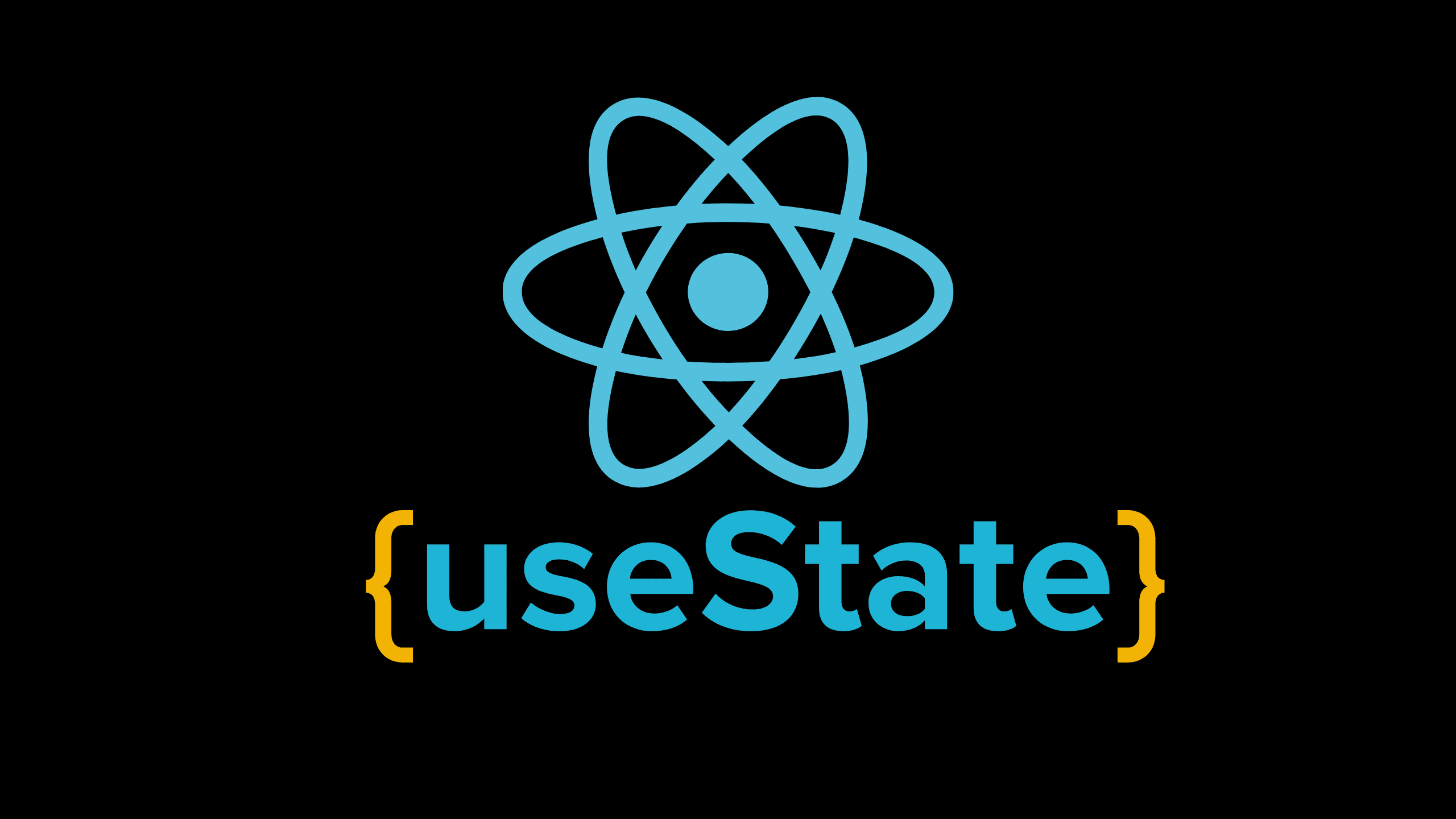
The useState hook is one of the simplest and most powerful tools in React. It allows you to add state to functional components, eliminating the need for class components. With useState, you can create dynamic, interactive user interfaces effortlessly.
How Does useState Work?
When you call useState, it returns an array with two elements:
- State Variable: The current state value.
- State Setter Function: A function to update the state value.
Here’s a basic example:
import React, { useState } from ‘react’;
function Counter() {
const [count, setCount] = useState(0); // Initial state set to 0
return (
<div>
<p>Count: {count}</p>
<button onClick={() => setCount(count + 1)}>Increment</button>
</div>
);
}
Build Modern Web Applications with ReactJS!
SDLC CORP ensures high-quality, responsive apps for all your business needs.
Key Features of useState
- Initial State: You can initialize the state with any data type, including strings, numbers, arrays, or objects.
- State Updates: Calling the setter function replaces the previous state value.
- Multiple States: You can use useState multiple times in a single component to manage different state variables.
Best Practices for useState
- Keep State Simple: Avoid deeply nested objects as state. Instead, split state into manageable pieces.
- Avoid Direct Mutation: Always use the setter function to update the state.
- Default Values: Always provide a sensible default value to avoid errors.
Understanding useState is fundamental for React app development, as it simplifies managing component-specific data.
- Keep State Simple: Avoid deeply nested objects as state. Instead, split state into manageable pieces.
Also check our latest blog : Server-Side Rendering with React: Best Practices
What is useEffect?
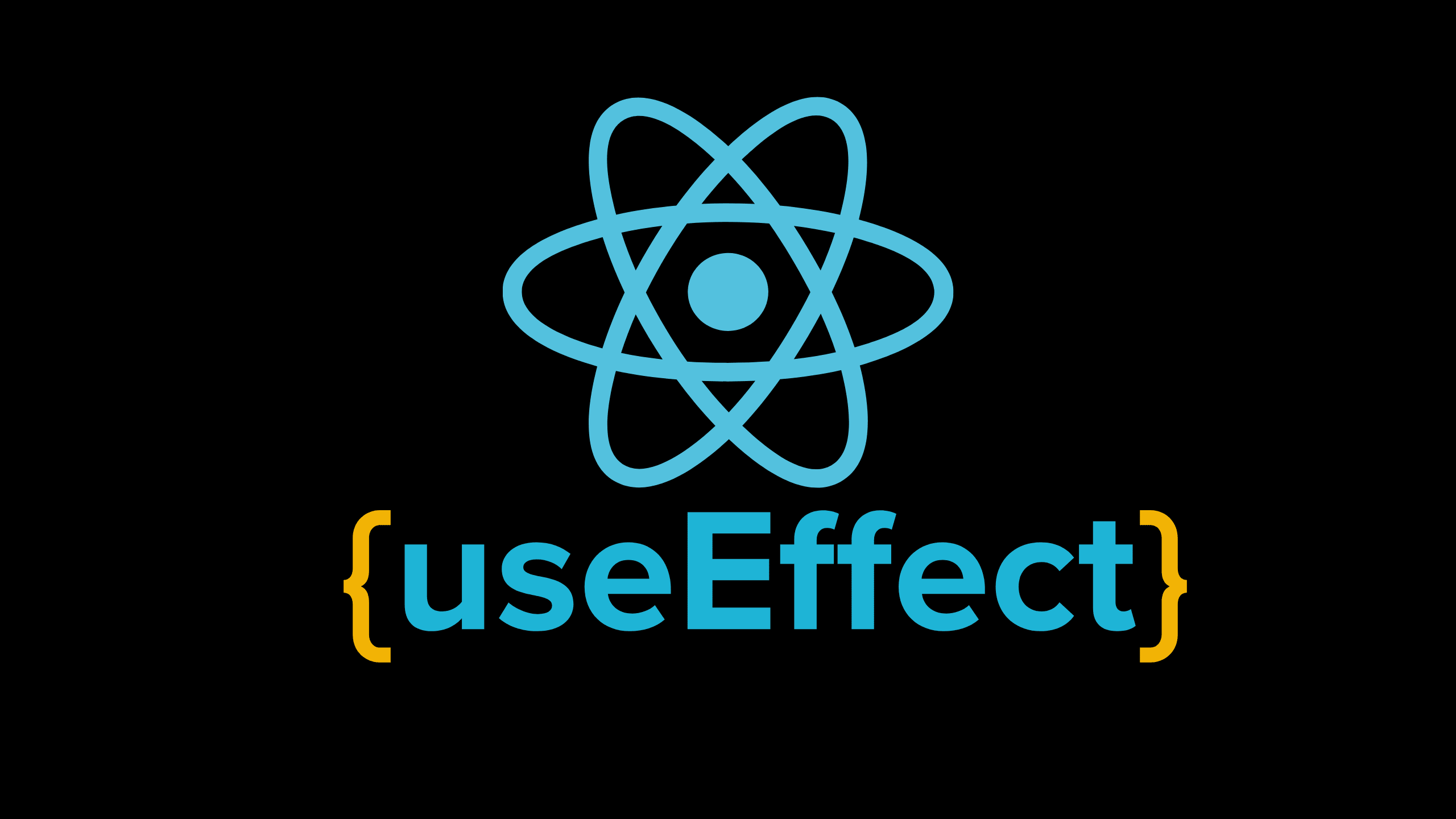
The useEffect hook is the go-to solution for managing side effects in functional components. Side effects include data fetching, DOM manipulation, subscriptions, and more. With useEffect, you can seamlessly integrate these functionalities into your components.
How Does useEffect Work?
The useEffect hook takes two arguments:
- Effect Function: Contains the code for the side effect.
- Dependency Array (optional): Specifies the variables that the effect depends on.
Here’s a simple example:
import React, { useState, useEffect } from ‘react’;
function Timer() {
const [seconds, setSeconds] = useState(0);
useEffect(() => {
const interval = setInterval(() => {
setSeconds(prev => prev + 1);
}, 1000);
return () => clearInterval(interval); // Cleanup function
}, [ ]); // Empty dependency array ensures it runs once
return <p>Seconds: {seconds}</p>;
}
Dependency Array in useEffect
The dependency array determines when the effect runs:
- Empty Array ([ ]): Effect runs only once after the initial render.
- Specific Dependencies: Effect runs when specified dependencies change.
- No Array: Effect runs after every render.
Best Practices for useEffect
- Cleanup Functions: Always clean up subscriptions, intervals, or event listeners to avoid memory leaks.
- Minimize Dependencies: Include only necessary variables in the dependency array.
- Split Effects: Break down complex effects into smaller, manageable ones.
The power of useEffect makes it a cornerstone of React mobile app and React website development projects, enabling developers to handle dynamic data and interactions efficiently.
Also check our latest blog : How to Build a Real-Time Chat App with React
Combining useState and useEffect
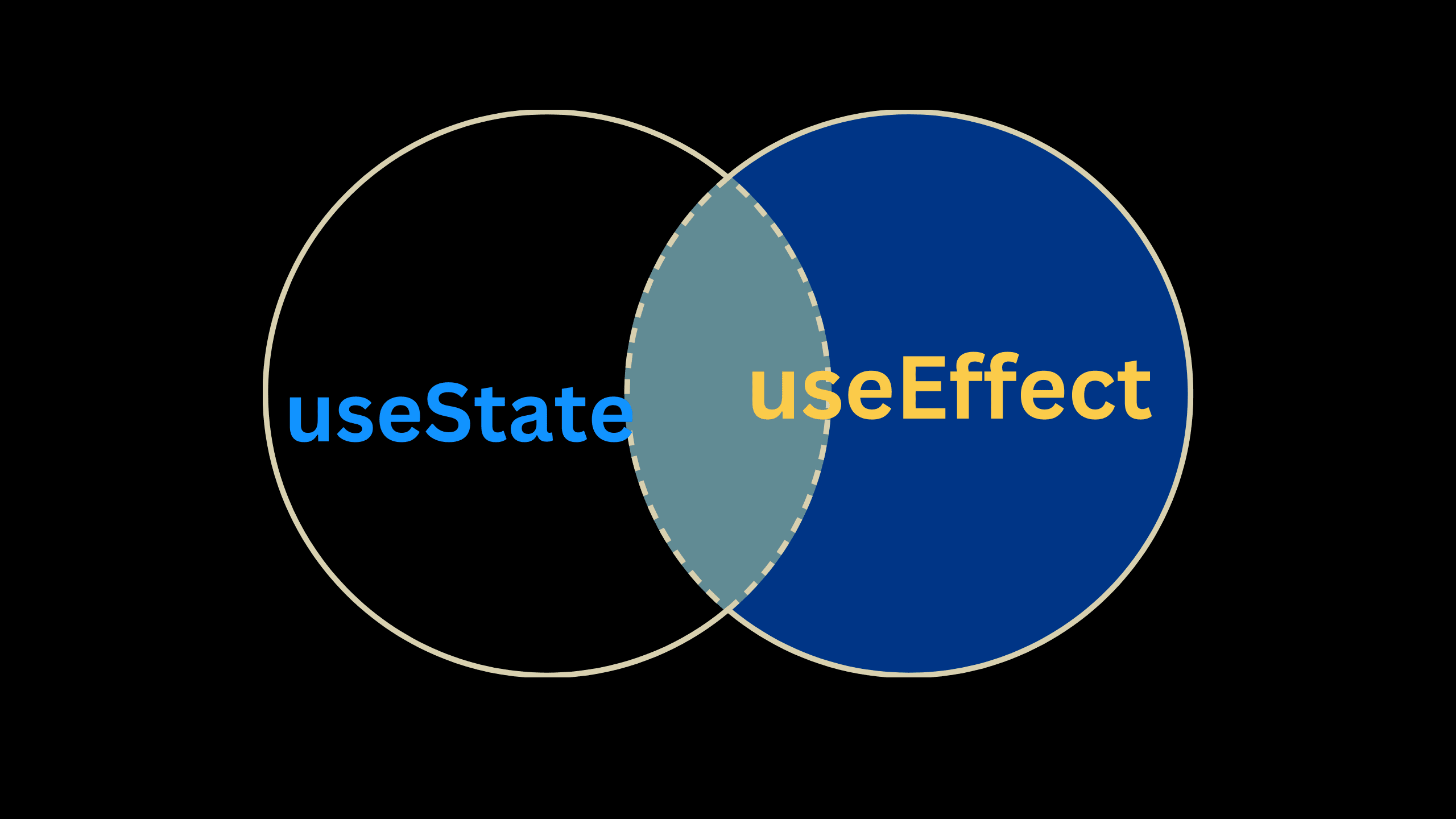
useState and useEffect often work together to create robust components. For example, you can fetch data from an API and store it in the state:
import React, { useState, useEffect } from ‘react’;
function DataFetcher() {
const [data, setData] = useState([ ]);
useEffect(() => {
fetch(‘https://api.example.com/data’)
.then(response => response.json())
.then(json => setData(json));
}, [ ]);
return (
<ul>
{data.map(item => (
<li key={item.id}>{item.name}</li>
))}
</ul>
);
}
This synergy is critical for developers in React JS development services looking to build applications that require real-time data.
Transform Ideas into ReactJS Solutions!
SDLC CORP delivers cutting-edge, user-friendly ReactJS applications.
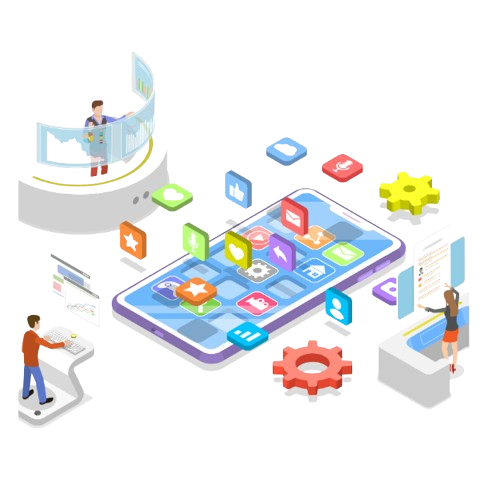
Common Pitfalls and How to Avoid Them
Overusing useEffect
Some developers use useEffect unnecessarily, causing performance issues. For example, calculations or state updates that can be handled within the component should not be placed in useEffect.
Infinite Loops
Leaving out the dependency array or incorrectly configuring it can cause infinite re-renders. Always ensure dependencies are correct.
Memory Leaks
Forgetting cleanup functions when using subscriptions or intervals can lead to memory leaks. Always return a cleanup function.
By addressing these pitfalls, React development services can ensure their applications remain performant and bug-free.
Advanced Tips for useState and useEffect
Lazy Initialization
If the initial state is expensive to compute, use lazy initialization:
const [value, setValue] = useState(() => computeExpensiveValue());
Custom Hooks
Combine useState and useEffect into reusable custom hooks:
function useFetchData(url) {
const [data, setData] = useState(null);
useEffect(() => {
fetch(url)
.then(response => response.json())
.then(setData);
}, [url]);
return data;
}
Custom hooks streamline complex logic and are invaluable in React app development projects.
Dependency Optimization
Use tools like eslint-plugin-react-hooks to detect dependency issues in useEffect.
Why React Hooks Matter in Real-World Development
React Hooks have transformed how developers approach state and lifecycle management. They simplify code, reduce boilerplate, and make components more readable. Whether you’re developing for the web, mobile, or building enterprise-grade React software, hooks like useState and useEffect are indispensable.
For companies offering React JS development or React website development services, embracing hooks ensures that your applications are built with modern, efficient practices. Developers can focus on delivering excellent user experiences while maintaining clean, scalable codebases.
Hire ReactJS Experts for Your Next Project!
Choose SDLC CORP for dynamic, responsive, and scalable ReactJS development.
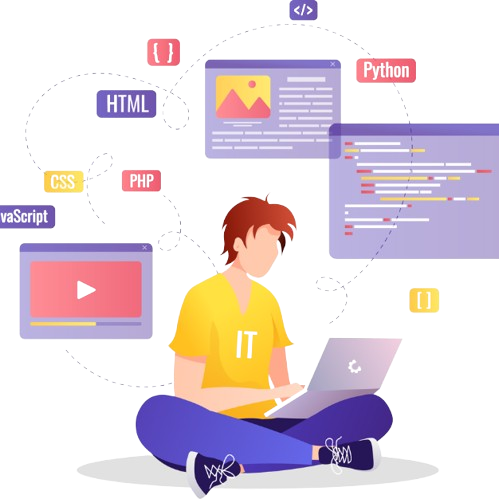
Conclusion
Mastering useState and useEffect is a must for anyone involved in React app development or working with a React development company. These hooks empower developers to build dynamic, responsive applications that are easy to maintain and scale.
Whether you’re an individual developer or part of a team delivering React development services, understanding these hooks will enhance your projects and position you as an expert in the field. As the foundation of modern React web development, these hooks enable you to harness the full power of React to build exceptional user experiences. Dive in, experiment, and make the most of these transformative tools!
SDLC CORP ReactJS Development Services Overview
SDLC CORP is a leading React development company offering top-notch solutions to build dynamic, high-performing, and scalable web applications. With a skilled team of ReactJS developers, we specialize in creating interactive user interfaces, seamless integrations, and tailored applications to meet diverse business needs. Choose SDLC CORP for innovative and reliable React development services.