When it comes to styling React applications, two powerful options have emerged: Tailwind CSS and Styled Components. Both of these tools offer unique approaches to styling React components, and as a React JS developer or a part of a React JS development company, it’s essential to understand the differences and determine which one best suits your needs.
In this post, we’ll compare Tailwind CSS and Styled Components in the context of React web development. We’ll explore their features, advantages, and trade-offs to help you make an informed decision when styling your next project, whether you’re developing React mobile apps or React website development. Let’s dive into the world of styling and explore these two approaches!
Introduction to Tailwind CSS and Styled Components
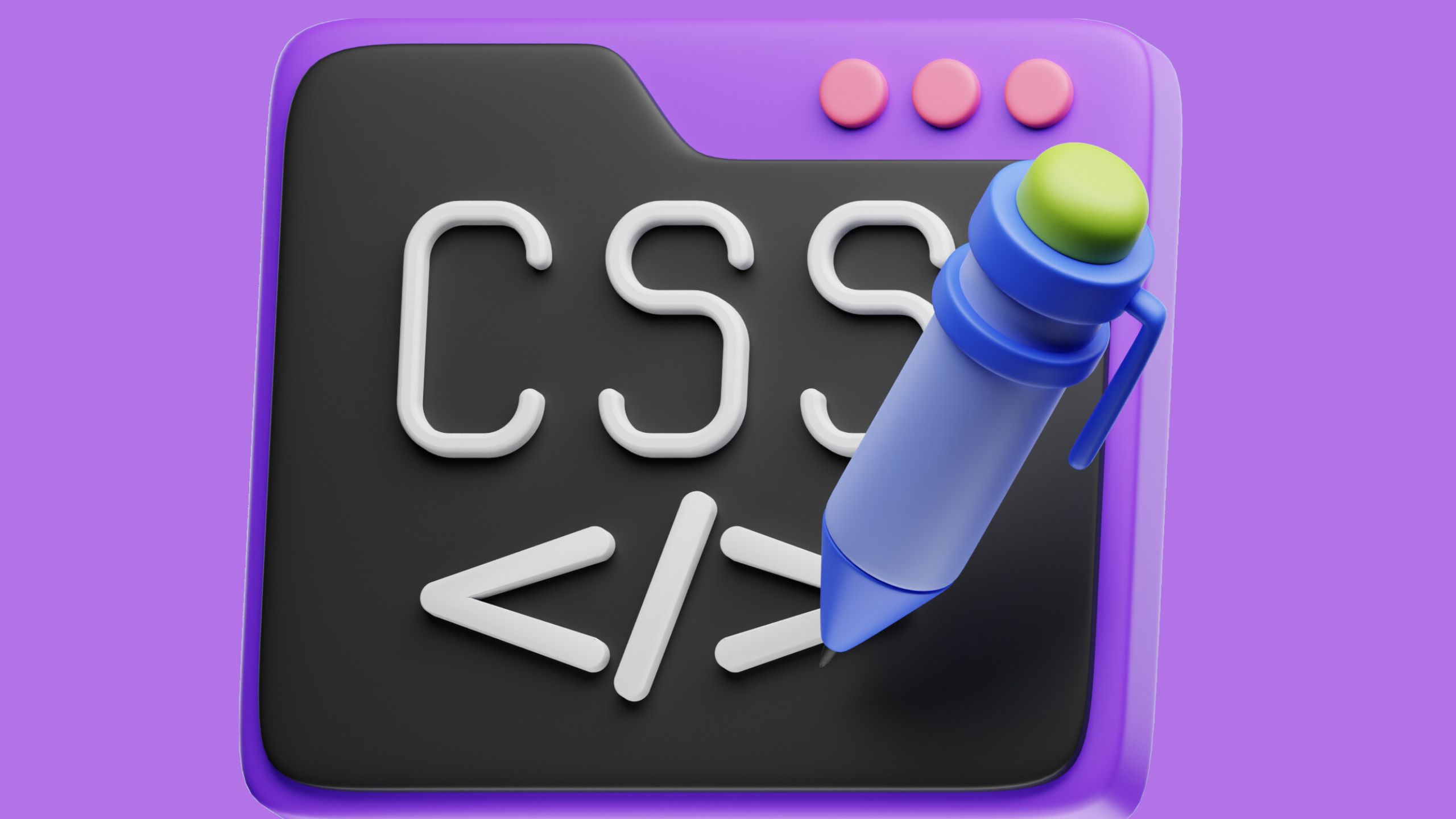
Before diving into the comparison, let’s first define what Tailwind CSS and Styled Components are, and how they approach styling in React apps.
What is Tailwind CSS?
Tailwind CSS is a utility-first CSS framework that provides a set of low-level utility classes to build custom designs directly in your markup. It follows the principle of “designing with classes” rather than writing traditional CSS. With Tailwind, you apply utility classes to your HTML elements to style them, without needing to write custom CSS rules.
What are Styled Components?
Styled Components is a popular CSS-in-JS library that allows you to style React components using JavaScript. Instead of writing separate CSS files, you define your styles within JavaScript using tagged template literals. This approach scopes the styles to individual components and gives you a way to manage CSS dynamically.
Both Tailwind CSS and Styled Components are widely used in React development services and React software projects. But which one is best suited for your project? Let’s break down their features, benefits, and drawbacks.
1. Philosophy and Approach
Tailwind CSS: Utility-First Approach
Tailwind CSS is built around the idea of a utility-first approach. Rather than creating complex CSS classes for each component, you use utility classes that apply specific styles. For example, instead of writing a custom CSS class for a button, you might use classes like bg-blue-500, text-white, and py-2 directly in your JSX to style it.
Pros of Tailwind CSS:
- Rapid Development: Tailwind speeds up development by eliminating the need to write custom CSS for each component.
- Consistency: Since you use a predefined set of utility classes, your UI will maintain a consistent design system.
- Customization: Tailwind allows you to customize the utility classes by modifying the configuration file. This gives you a high level of flexibility.
- No Global Styles: Tailwind ensures that all styles are scoped to the elements you apply them to, reducing the risk of CSS conflicts.
Cons of Tailwind CSS:
- Verbose Markup: Since you apply styles directly to your elements, your JSX can become cluttered with utility classes, making it harder to read.
- Learning Curve: If you’re new to utility-first design systems, there may be a learning curve to understand the class names and configurations.
- Lack of Component-Level Styling: Tailwind does not encourage component-specific styling, which may lead to challenges in larger projects.
Launch High-Performance ReactJS Applications!
Work with SDLC CORP to transform your ideas into stunning digital products.
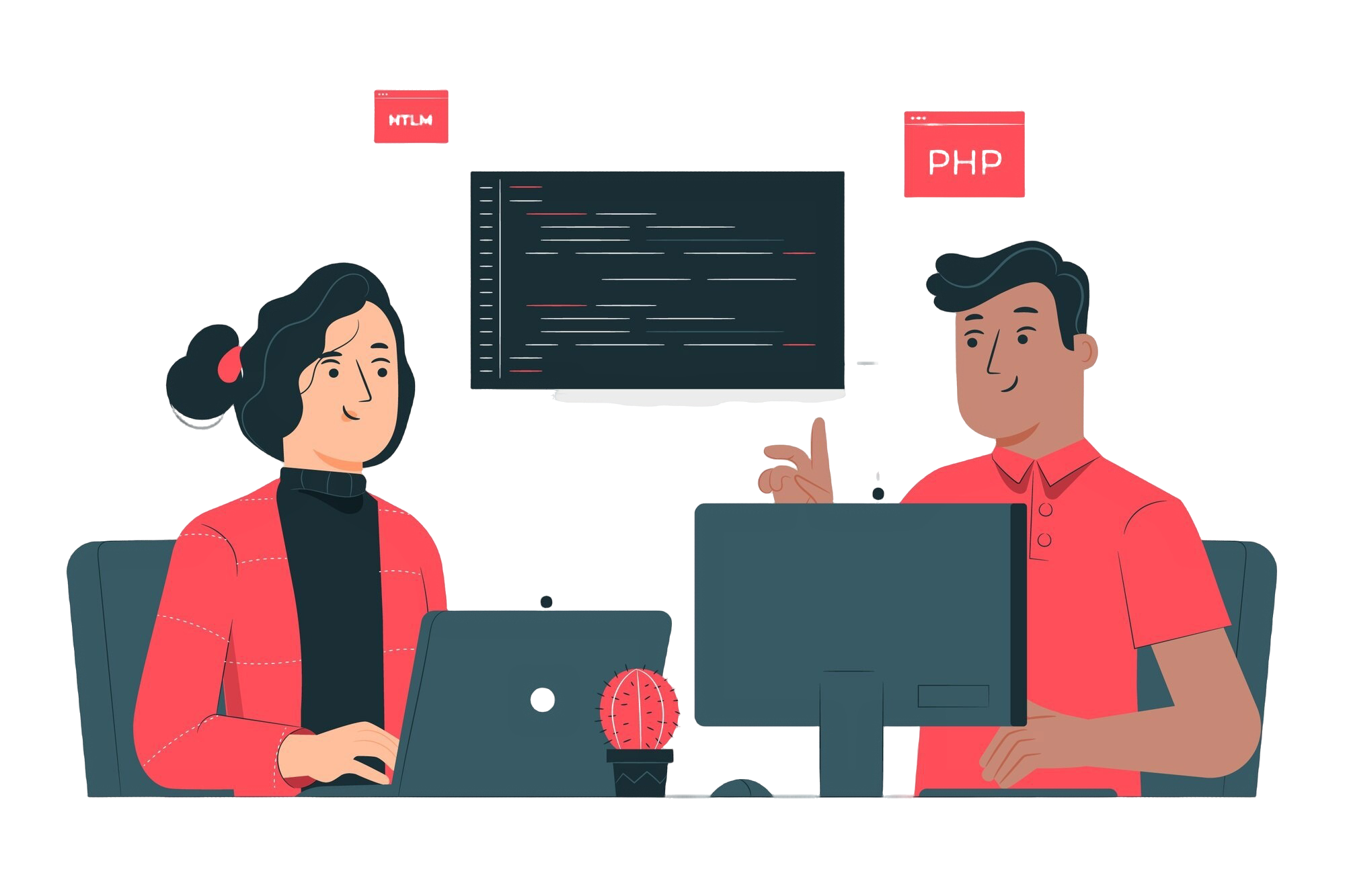
Styled Components: Component-Level Styling
Styled Components takes a different approach by using CSS-in-JS to style components. You write the styles directly inside your JavaScript files, scoped to the components they belong to. This means that your styles are tightly coupled with the components, ensuring that styles are isolated and not subject to leakage.
Pros of Styled Components:
- Component-Centric Styling: Styles are directly tied to the components, which makes the code more modular and maintainable.
- Dynamic Styling: Styled Components allow you to pass props into styled components, enabling dynamic styling based on component state or props.
- No Global Styles: Since styles are scoped to the component, there’s no risk of global styles overriding each other, which can often be a problem in traditional CSS.
- Easier to Read: Styles are in the same file as your components, which makes it easier to see both the markup and styling together.
Cons of Styled Components:
- Performance Overhead: Styled Components can introduce performance issues in larger apps because the styles are generated at runtime.
- Dependency on JavaScript: Since the styles are embedded within JavaScript, the app becomes more dependent on JavaScript, which can complicate server-side rendering (SSR) or static site generation.
CSS Duplication: If not handled properly, Styled Components can result in repeated styles across different components, leading to potential inefficiencies in larger apps.
2. Performance
Tailwind CSS Performance
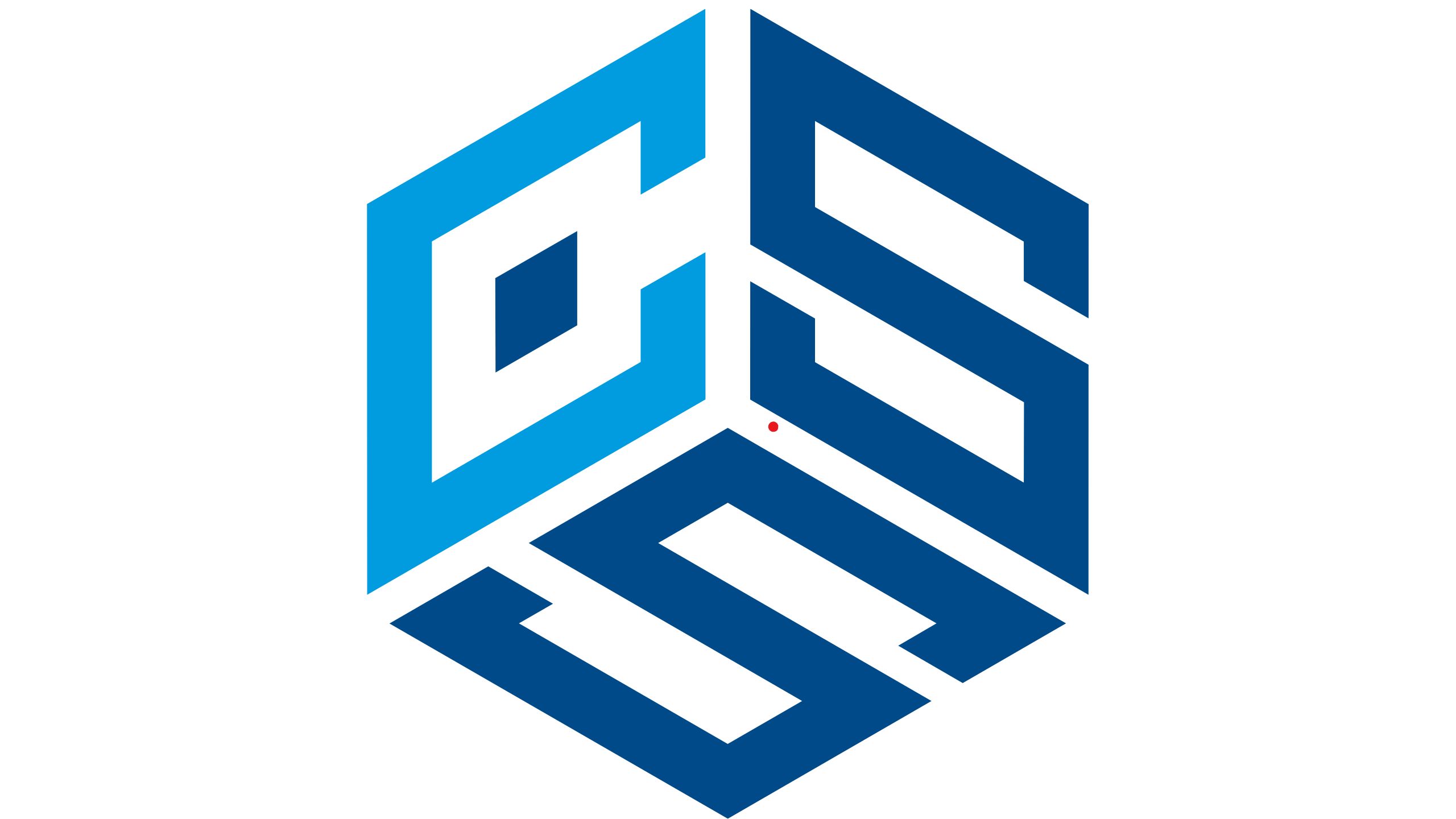
Tailwind CSS is optimized for performance right out of the box. Since you’re using utility classes, the final CSS bundle is highly optimized. The framework only includes the styles that you use in your markup, which can significantly reduce the size of your CSS file. Tools like PurgeCSS can be used to remove unused styles from the final build, ensuring that only the necessary styles are included.
Tailwind CSS Optimization:
- Purging Unused CSS: With Tailwind, unused styles are purged during the build process, reducing the size of the CSS file.
- Smaller Bundle Size: Since you only use the classes you need, the overall bundle size tends to be smaller compared to traditional CSS frameworks.
Styled Components Performance
Styled Components, on the other hand, introduces some runtime overhead because the styles are dynamically injected into the DOM. When a component is rendered, the corresponding styles are created and inserted into the DOM at runtime. While this is typically not a problem for small to medium-sized applications, larger React apps may experience performance bottlenecks, especially if many styled components are rendered at once.
Styled Components Optimization:
- Server-Side Rendering (SSR): Styled Components can be used with SSR, but it requires additional configuration to avoid issues with rendering styles on the server and client.
- CSS Caching: To optimize performance, Styled Components cache generated styles to avoid recalculating them for every render. However, this can still introduce overhead in complex apps.
Build Fast, Scalable ReactJS Apps Today!
Partner with SDLC CORP for top-notch ReactJS development solutions.
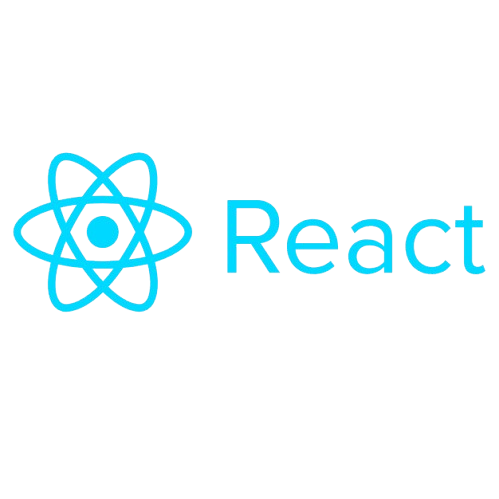
3. Developer Experience
Tailwind CSS Developer Experience
For developers used to traditional CSS, Tailwind may seem like a shift in mindset. However, once you get the hang of it, you may find that Tailwind’s utility-first approach makes it faster to build designs without needing to jump between HTML and CSS files.
Tailwind CSS Developer Experience Highlights:
- Rapid Prototyping: You can quickly prototype layouts and components by applying utility classes directly to the JSX.
- Intellisense Support: Tailwind has strong IDE support, with autocompletion for class names, making it easier to work with.
- Customization: Tailwind can be easily customized through its config file, allowing you to tailor it to your project’s design requirements.
Styled Components Developer Experience
Styled Components offers a highly flexible and dynamic approach to styling. The fact that styles are co-located with the components makes it easy to understand and maintain. Additionally, Styled Components allows for dynamic styling, which can be very useful when building complex components with varying states.
Styled Components Developer Experience Highlights:
- Component-Level Styling: The styling is scoped to the component, making it easy to reason about and maintain.
- Dynamic Styles: You can pass props into styled components to change their styles dynamically based on component state or external inputs.
- CSS Familiarity: Developers familiar with traditional CSS will feel right at home with the Styled Components approach, as it follows standard CSS syntax.
4. Use Case Scenarios
When to Use Tailwind CSS in React Development
If you’re working on a project where speed and consistency are crucial, Tailwind CSS might be the better choice. Tailwind shines in projects where you need to build fast, responsive layouts with minimal effort. It’s also an excellent choice for teams that need to enforce a design system or for projects that require a high level of customization but don’t want to spend time writing a lot of custom CSS.
Use Tailwind CSS for:
- Rapid prototyping and MVPs: If you need to quickly get a project off the ground, Tailwind’s utility-first approach makes it easy to build out designs without worrying too much about custom styles.
- Design systems: If you’re building a design system that needs to be scalable and consistent, Tailwind CSS is a great option.
- Small to medium-sized projects: For projects where you don’t need complex component-level styling, Tailwind works well.
When to Use Styled Components in React Development
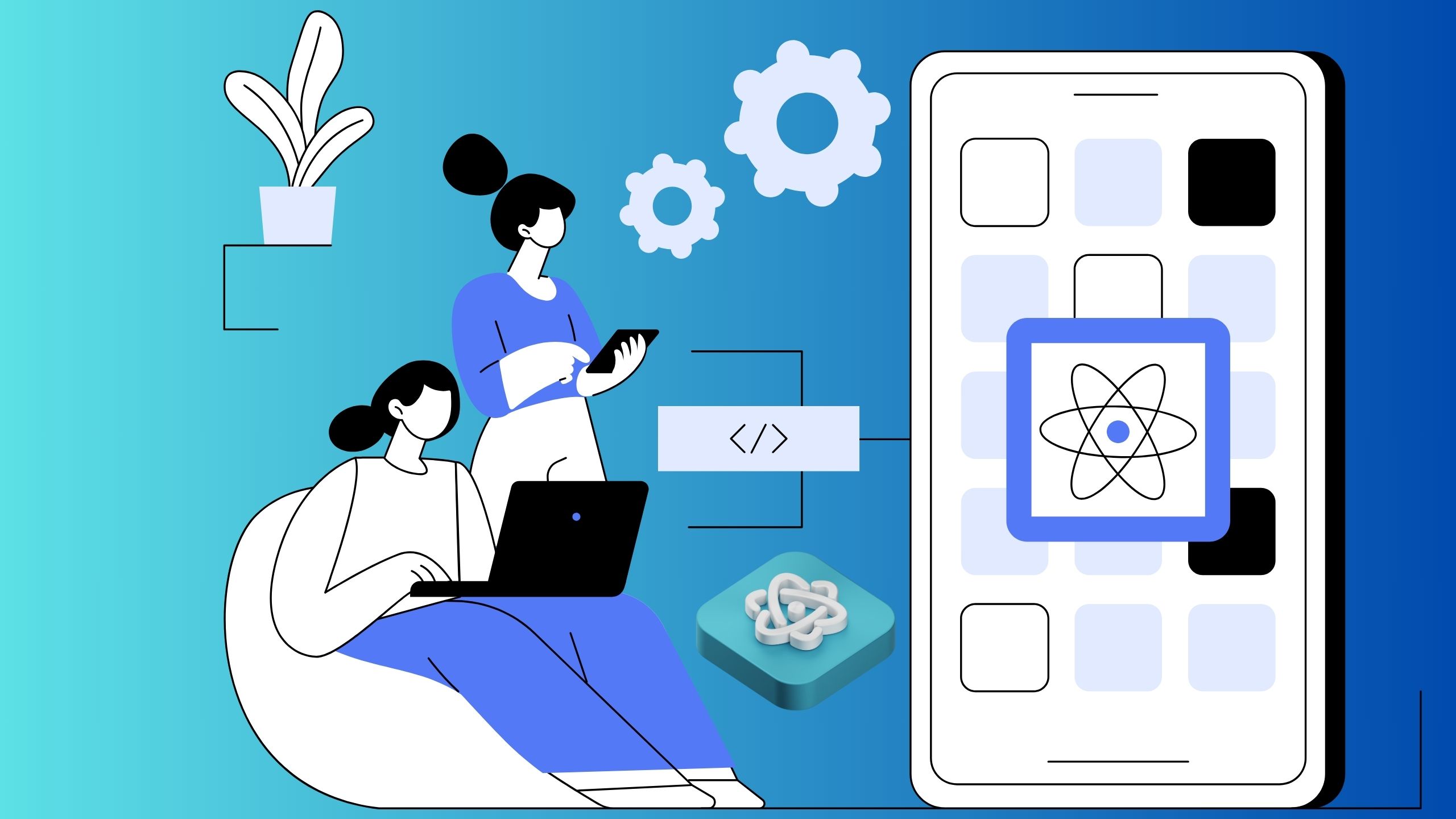
If your project involves complex components with dynamic styling needs, Styled Components might be the better option. Styled Components is ideal for applications that have a lot of interactive elements and where the styles are dependent on props or state. Additionally, if you prefer keeping styles co-located with your components, Styled Components is the way to go.
Use Styled Components for:
- Dynamic and interactive components: When components need to adapt to different states or props, Styled Components gives you the flexibility to handle that.
- Large-scale React applications: For larger applications where you want to maintain a clear and modular structure, Styled Components keeps everything in one place and reduces the chance of style leakage.
- Server-side rendering (SSR): If your project involves SSR, Styled Components can be configured to work with React’s SSR setup.
5. Community and Ecosystem
Both Tailwind CSS and Styled Components have large, active communities, which can be a significant advantage when building applications. A vast ecosystem of plugins, tools, and tutorials is available for both libraries, making it easier to find support when needed.
Tailwind CSS Community:
- Extensive Documentation: Tailwind has excellent documentation, and there are many third-party tools and plugins available.
- Growing Adoption: More and more companies are adopting Tailwind CSS for their React projects, and the community is growing rapidly.
Styled Components Community:
- CSS-in-JS Ecosystem: Styled Components is part of the larger CSS-in-JS movement, which has seen widespread adoption in the React ecosystem.
- Robust Support: Styled Components has great community support, with lots of tutorials, resources, and integrations available.
Accelerate Your Business with ReactJS!
Choose SDLC CORP for dynamic, responsive, and scalable ReactJS development.
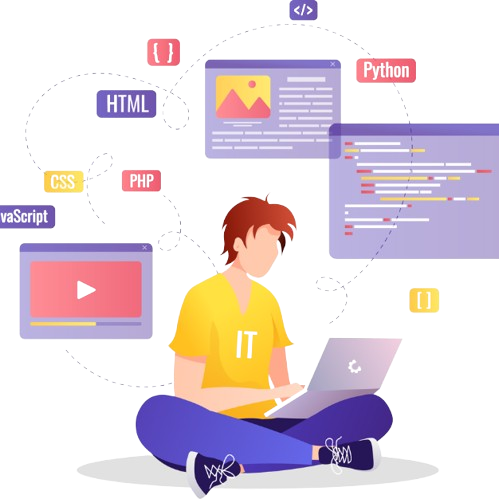
Conclusion: Tailwind CSS or Styled Components?
Both Tailwind CSS and Styled Components offer compelling advantages, but the choice ultimately depends on your project’s needs and the way you prefer to work.
- Tailwind CSS is a great choice if you prefer working with utility classes and need to quickly build responsive layouts without the overhead of writing custom CSS.
- Styled Components is the way to go if you prefer co-locating your styles with components and need dynamic styling that depends on props and state.
As a React JS development company or a React JS developer, the best tool depends on the complexity of your project, the scale of the application, and your personal preference. For projects where rapid prototyping and consistency are key, Tailwind CSS shines, while Styled Components excels in applications that require dynamic, component-specific styling.
Ultimately, both tools have a place in React development services, and the decision comes down to how you want to structure and maintain your application’s styles. Happy coding!
SDLC CORP ReactJS Development Services Overview
SDLC CORP offers top-notch React website development services to create dynamic, scalable, and user-friendly web applications. Our expert team leverages ReactJS to deliver high-performance solutions tailored to meet your business needs, ensuring a seamless user experience and robust functionality. Partner with us to build innovative, modern websites that drive growth and engagement.