In modern web development, efficient data fetching is crucial for building fast, responsive, and scalable applications. When you build applications with React, one of the key challenges is managing and fetching data from various sources. While traditional REST APIs have been a go-to solution, GraphQL has emerged as a powerful alternative, offering more flexibility and efficiency in handling data.
GraphQL allows developers to fetch only the data they need, reducing over-fetching and under-fetching issues often seen in REST APIs. If you’re a React JS developer or part of a React JS development company, integrating GraphQL with React can significantly improve the performance and maintainability of your applications. Whether you’re working on a React mobile app or a large-scale React website development project, leveraging GraphQL can streamline your data management and enhance your app’s efficiency.
In this blog post, we’ll explore the benefits of using GraphQL with React, how to get started, and best practices for integrating GraphQL into your React development workflow.
What is GraphQL?
Launch High-Performance ReactJS Applications!
Work with SDLC CORP to transform your ideas into stunning digital products.
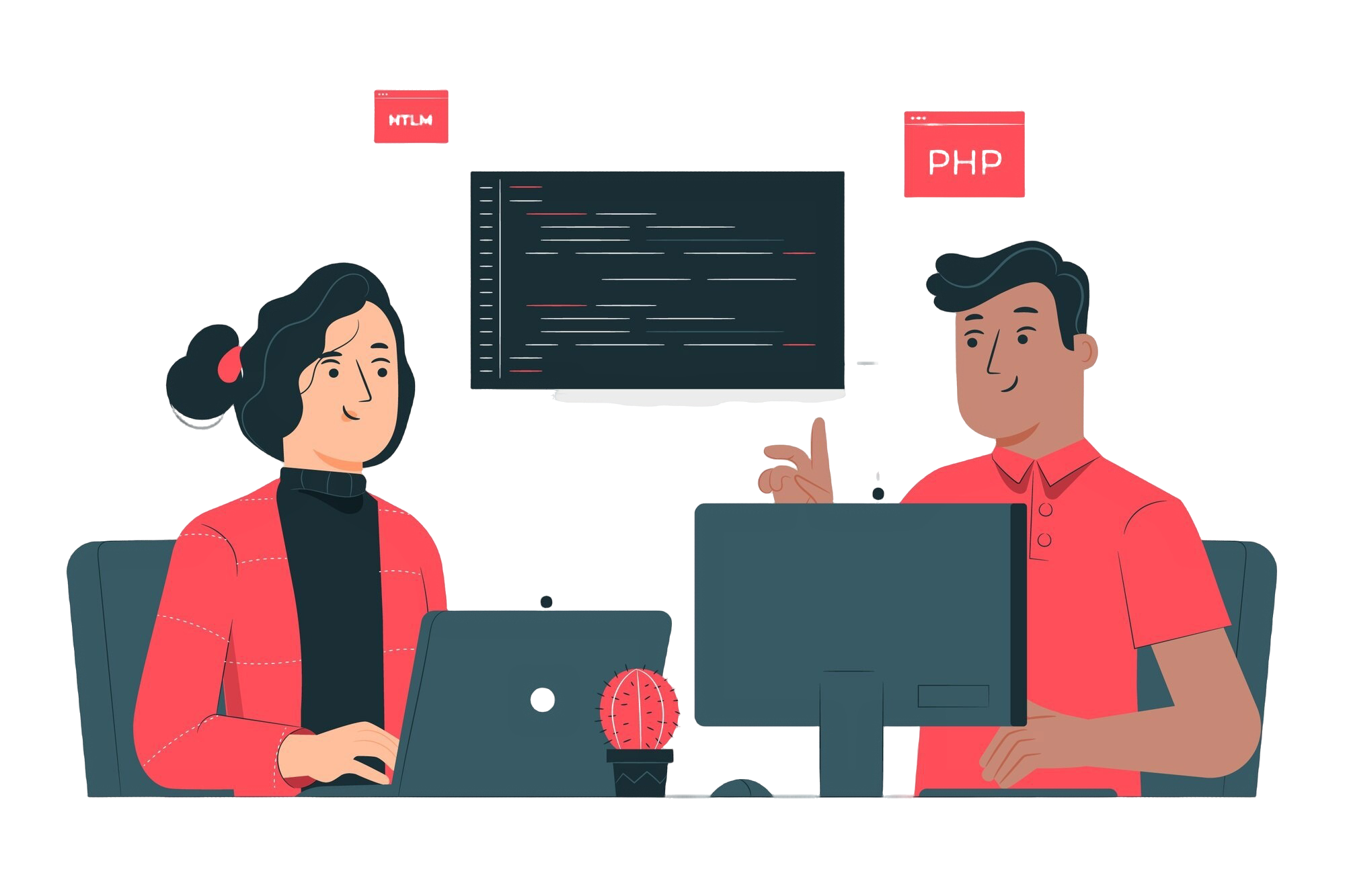
Before diving into the specifics of using GraphQL with React, let’s take a quick look at what GraphQL is and how it differs from traditional REST APIs.
GraphQL is a query language for your API that allows clients to request exactly the data they need, no more and no less. It was developed by Facebook and has quickly become popular due to its flexibility, efficiency, and developer-friendly nature. In contrast to REST, where multiple endpoints are used to fetch different sets of data, GraphQL provides a single endpoint for fetching data and gives clients the power to specify the structure of the response.
Key Benefits of Using GraphQL:
- Efficient Data Fetching: With GraphQL, you can request only the data you need, avoiding the problem of over-fetching or under-fetching data.
- Single Endpoint: Unlike REST APIs, which often require multiple endpoints for different resources, GraphQL allows you to use a single endpoint for all queries, mutations, and subscriptions.
- Strongly Typed Schema: GraphQL APIs are self-documenting. The schema defines the types and structure of data, making it easier to understand and work with.
- Improved Performance: By reducing unnecessary data loading, GraphQL improves the overall performance of your application, particularly in scenarios where data needs to be fetched from multiple sources.
Why Use GraphQL with React?
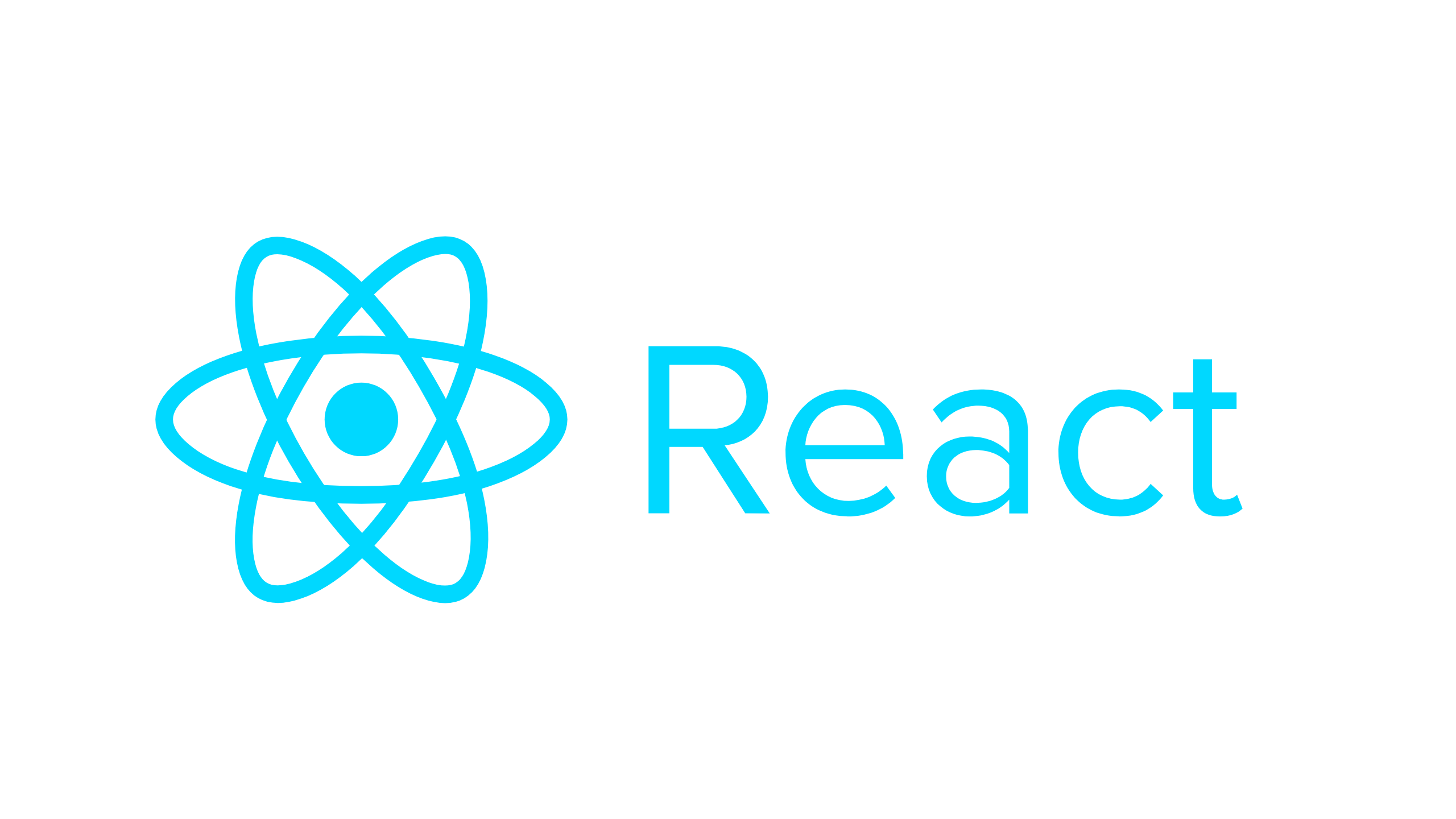
React’s component-based architecture pairs perfectly with GraphQL’s flexibility. The main advantage of using GraphQL with React lies in its ability to fetch data efficiently, leading to improved performance and better user experience. Whether you’re working on React web development or React app development, the integration of GraphQL with React allows you to manage your data fetching with greater ease and precision.
Here’s why you might want to use GraphQL with React:
Launch High-Performance ReactJS Applications!
Work with SDLC CORP to transform your ideas into stunning digital products.
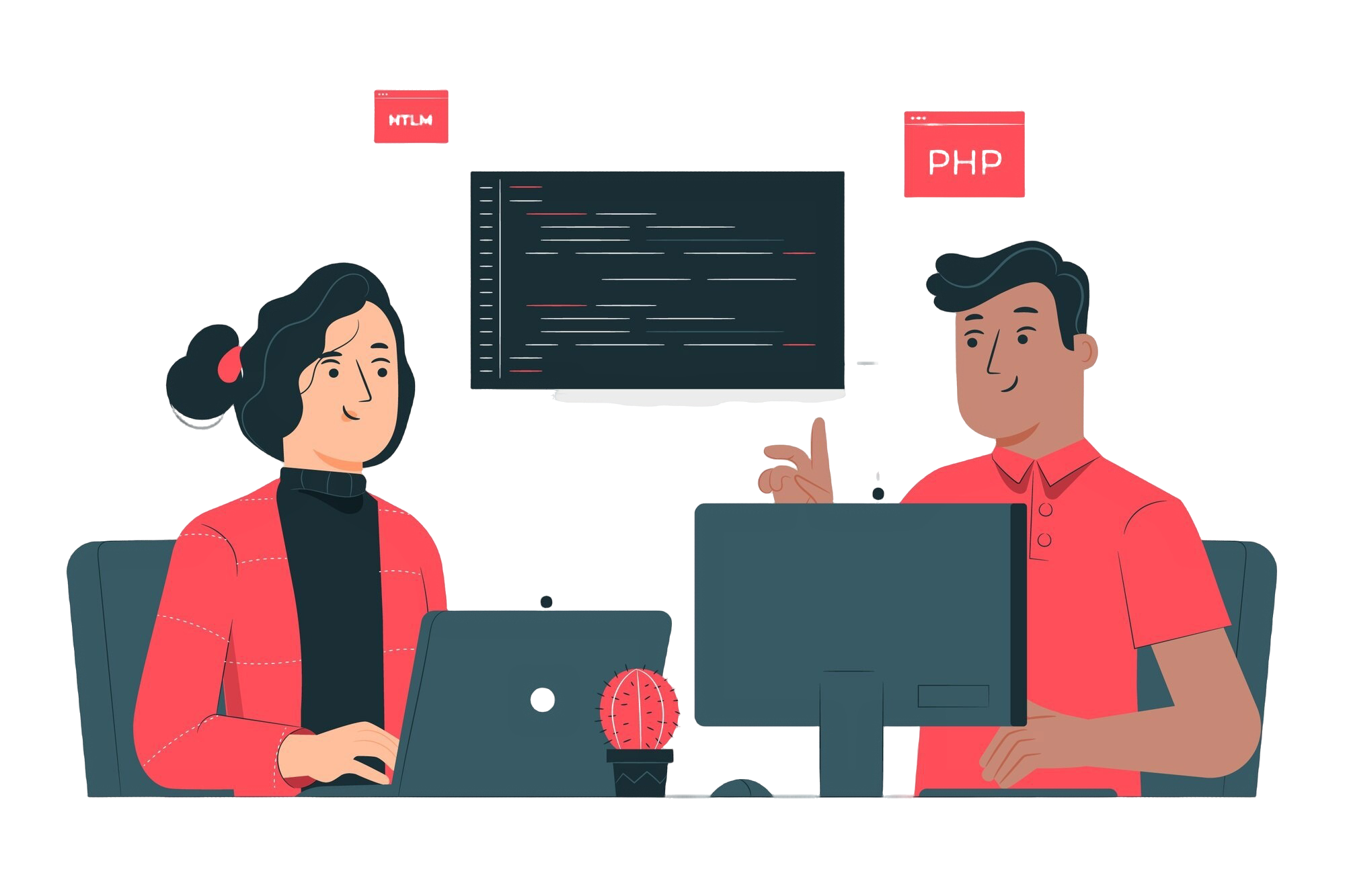
1. Declarative Data Fetching
React promotes declarative programming, and GraphQL aligns perfectly with this philosophy. With GraphQL, you can specify the exact data you need for a component without worrying about the complexities of managing multiple API requests. This declarative approach makes your code cleaner and easier to maintain.
Instead of dealing with multiple fetch
or axios
calls for different endpoints, you can use a single GraphQL query to retrieve exactly what the component needs. This makes it easier to maintain and reduces the risk of fetching redundant or unnecessary data.
2. Reduced Number of Network Requests
In traditional REST APIs, it’s common to make multiple network requests to fetch related data. For example, you might need to make separate requests for user data, post data, and comments. This can result in a performance bottleneck, especially in mobile apps where bandwidth and latency can be issues.
GraphQL resolves this by allowing you to fetch all the related data in a single request. If you’re building a React mobile app or a React website, this can greatly reduce the load time and improve the user experience.
3. Handling Complex Data Structures
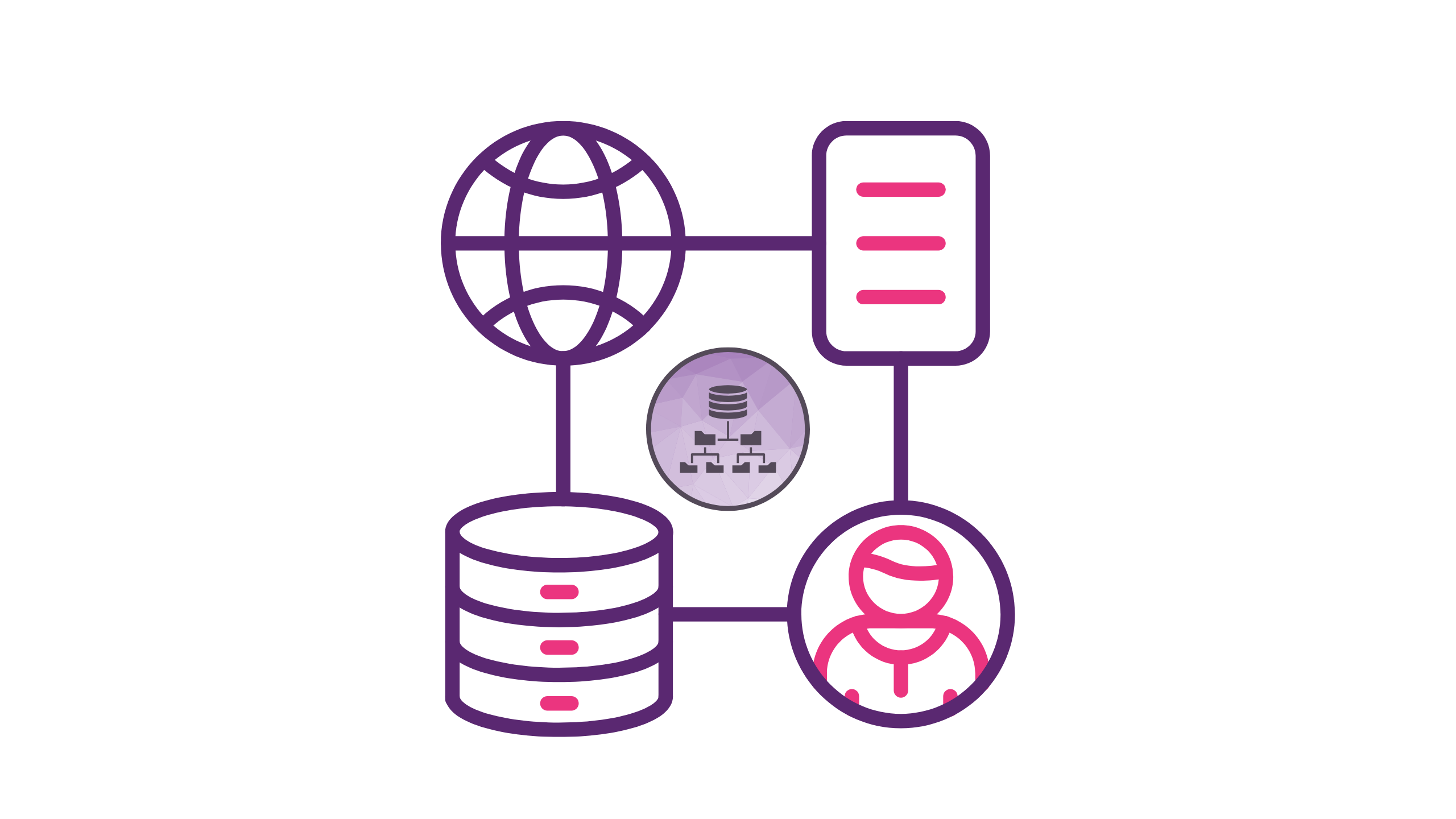
In large applications, you often need to fetch complex, nested data. With GraphQL, you can request precisely the fields you need from deeply nested data structures, avoiding the hassle of dealing with deep object manipulations and overly large responses.
If you’re working on a React development services project that involves complex data relationships, like product catalogs, social feeds, or user-generated content, GraphQL can simplify the way you work with that data.
4. Better State Management Integration
In React, managing state effectively is a critical aspect of any application. When you integrate GraphQL, you can streamline state management by using tools like Apollo Client. Apollo Client is a comprehensive state management library that works seamlessly with GraphQL. It automatically handles caching, network requests, and data synchronization between your UI and the backend, making it easier to focus on building features instead of managing state manually.
If your project involves multiple components that need to access shared data, using Apollo Client for React software solutions can help centralize data management and improve maintainability.
How to Get Started with GraphQL and React
Now that we understand the benefits of using GraphQL with React, let’s explore the basics of integrating GraphQL into a React app.
1. Set Up a GraphQL Server
Before you can interact with GraphQL from your React application, you’ll need a GraphQL server. If you’re using a React JS development company for your project, they may have already set up a GraphQL server for you. But if you’re building your own app, there are several ways to set up a server, including using libraries like Apollo Server, Express-GraphQL, or GraphQL Yoga.
For demonstration purposes, let’s assume you’re interacting with an existing GraphQL API, whether it’s your own or from a third-party service.
Build Fast, Scalable ReactJS Apps Today!
Partner with SDLC CORP for top-notch ReactJS development solutions.
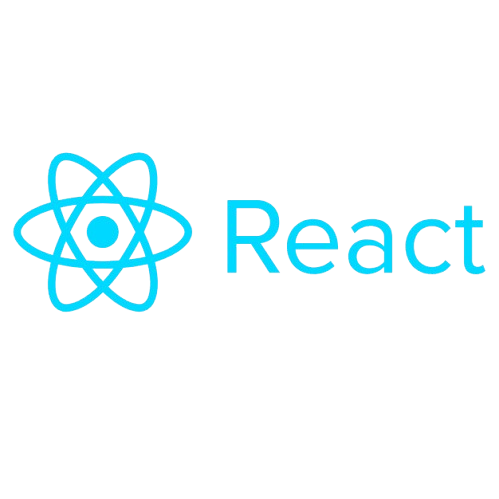
2. Install Apollo Client
The most popular way to connect React with GraphQL is through Apollo Client, a powerful library that integrates GraphQL queries with React components. To install it, simply run:
npm install @apollo/client graphql
Apollo Client simplifies data management and interaction with a GraphQL server. It takes care of caching, data synchronization, and query execution, allowing you to focus on building your app.
3. Set Up Apollo Provider
Once Apollo Client is installed, you need to set up the ApolloProvider component at the root of your application to provide the Apollo Client instance to the entire app:
import React from 'react';import ReactDOM from 'react-dom';import { ApolloProvider, InMemoryCache } from '@apollo/client';import App from './App';// Initialize Apollo Clientconst client = new ApolloClient({ uri: 'https://your-graphql-endpoint.com/graphql', // Your GraphQL endpoint cache: new InMemoryCache(),
});ReactDOM.render( <ApolloProvider client={client}> <App /> </ApolloProvider>, document.getElementById('root')
);
4. Querying Data with Apollo Client
After setting up the Apollo Client, you can use the useQuery hook to fetch data from the GraphQL API inside your React components. This hook simplifies data fetching and handles loading, error, and success states automatically:
import React from 'react';import { useQuery, gql } from '@apollo/client';// Define the GraphQL queryconst GET_USERS = gql` query GetUsers { users { id name email } }`;const UsersList = () => { // Use the useQuery hook to fetch data const { loading, error, data } = useQuery(GET_USERS); if (loading) return <p>Loading...</p>; if (error) return <p>Error: {error.message}</p>; return ( <ul> {data.users.map((user) => ( <li key={user.id}> {user.name} - {user.email} </li> ))} </ul> );
};export default UsersList;
5. Mutating Data with Apollo Client
Apollo Client allows you to perform mutations, which are operations that modify server-side data, such as creating, updating, or deleting records. To execute a mutation, use the useMutation
hook, providing a GraphQL mutation string. This hook returns a mutate function and a result object. Call the mutate function with variables matching the mutation’s requirements. You can also update the Apollo cache to reflect changes locally for a seamless UI experience. Error handling and loading states can be managed effectively within the result object.
6. Handling Errors and Optimistic UI
GraphQL allows for optimistic UI updates, which means the UI can display changes immediately, even before the server responds. This is particularly useful for creating a smooth user experience in apps where data needs to be updated quickly.
Accelerate Your Business with ReactJS!
Choose SDLC CORP for dynamic, responsive, and scalable ReactJS development.
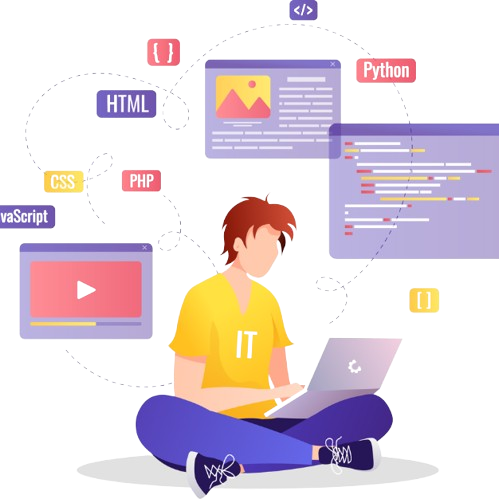
Conclusion
Integrating GraphQL with React allows you to fetch data efficiently, reduce the number of requests, and optimize your application’s performance. Whether you’re building a React mobile app, a React web development project, or a full-scale React software solution, GraphQL is an excellent tool to manage your app’s data fetching needs. With libraries like Apollo Client, you can streamline your state management and make your code more maintainable and scalable.
If you’re looking to integrate GraphQL into your project or need help with React JS development services, reaching out to an experienced React JS development company can ensure your application is built efficiently, providing a seamless user experience with optimal performance.
SDLC CORP ReactJS Development Services Overview
SDLC CORP offers top-notch React website development services to create dynamic, scalable, and user-friendly web applications. Our expert team leverages ReactJS to deliver high-performance solutions tailored to meet your business needs, ensuring a seamless user experience and robust functionality. Partner with us to build innovative, modern websites that drive growth and engagement.