Introduction
In the ever-evolving world of modern web development, managing application state efficiently is crucial for ensuring smooth functionality and user experience. As a technology that simplifies this process, React Context has become a game-changer for developers building scalable applications. If you’re looking to hire a React JS development company or explore React JS development services, understanding React Context can help you leverage its power to streamline your application’s state management without adding unnecessary complexity.
Whether you’re a React JS developer or a business interested in React web development, this guide will provide an in-depth understanding of how React Context works, its benefits, and best practices to utilize it effectively.
What Is React Context?
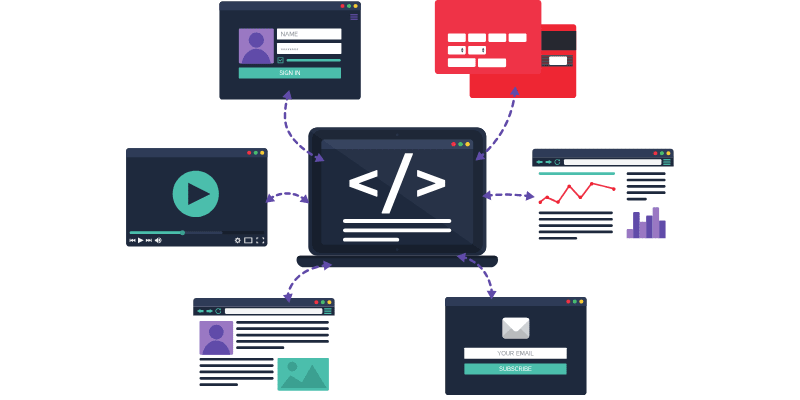
React Context is a feature introduced in React 16.3 that allows you to share state across your application without the need to prop-drill through every component. It provides a way to pass data through the component tree without having to pass props manually at every level.
Key Features of React Context:
- Centralized State Management: Unlike prop-drilling, where data must be passed through every component, Context makes state accessible globally.
- Simplifies Complex Applications: Ideal for managing global data such as themes, authentication, or user preferences.
- Reduces Boilerplate Code: Eliminates the need for intermediary components to serve as pass-throughs.
For businesses relying on React development services, understanding the power of Context ensures faster and more efficient development cycles, leading to cost savings and better performance.
Hire ReactJS Experts for Your Next Project!
Choose SDLC CORP for dynamic, responsive, and scalable ReactJS development.
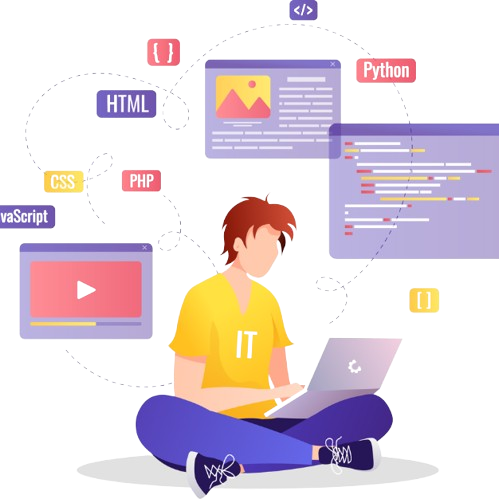
How React Context Works
The Building Blocks of React Context
React Context consists of three main elements:
- React.createContext(): Creates a Context object that holds the state and provides methods to access it.
- Provider Component: The Provider wraps around your component tree and supplies the context value.
- Consumer Component: The Consumer reads the context value
Here’s a simple example:
import React, { createContext, useContext } from ‘react’;
// Create a Context
const ThemeContext = createContext();
// Provider Component
const ThemeProvider = ({ children }) => {
const theme = ‘dark’;
return (
<ThemeContext.Provider value={theme}>
{children}
</ThemeContext.Provider>
);
};
// Consumer Component
const ThemeButton = () => {
const theme = useContext(ThemeContext);
return <button>{`Current Theme: ${theme}`}</button>;
};
// Application
const App = () => (
<ThemeProvider>
<ThemeButton />
</ThemeProvider>
);
export default App;
This example demonstrates how React Context simplifies the state-sharing process, making it an indispensable tool for React app development.
Benefits of Using React Context
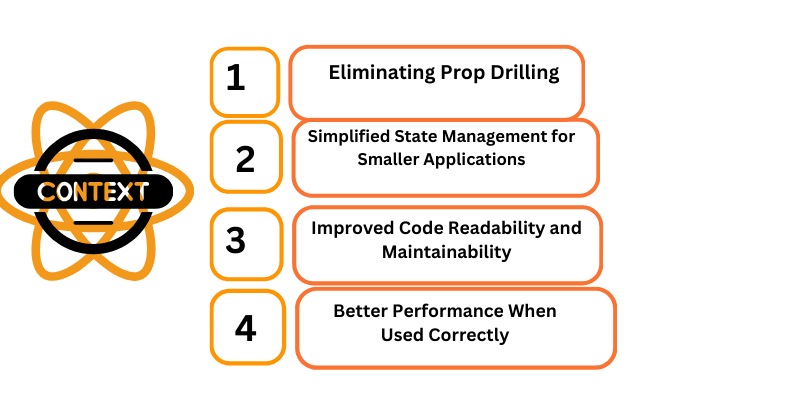
1. Eliminating Prop Drilling
Prop drilling occurs when you pass data down multiple layers of components, which can lead to unwieldy and error-prone code. With React Context, you eliminate this issue by sharing data directly at the desired level.
2. Simplified State Management for Smaller Applications
For applications that don’t require the full power of state management libraries like Redux, React Context is an excellent choice. It offers a simpler and lightweight solution for managing state.
Also check our latest blog : React 18 Concurrent Rendering: What You Need to Know
3. Improved Code Readability and Maintainability
When working on React software or a React mobile app, clean and maintainable code is essential. Context improves readability by reducing unnecessary complexity, making it easier to onboard new developers and debug issues.
4. Better Performance When Used Correctly
Although Context may lead to unnecessary re-renders if misused, combining it with techniques like memoization ensures optimal performance in even the most complex React website development projects.
When Should You Use React Context?
Ideal Use Cases
- Global Themes: Managing light and dark themes across your application.
- Authentication State: Sharing user login status and user roles.
- Language/Localization Settings: For applications requiring multilingual support.
- Cart or Shopping Data: Common in React app development for e-commerce platforms.
When Not to Use React Context
While React Context is a powerful tool, it’s not always the best fit for all scenarios. Avoid using it for:
- Highly Dynamic State: For frequently updated states, consider using dedicated libraries like Redux or Zustand.
- Small and Isolated State: If the state is specific to a single component or a small group of components, React Context might add unnecessary complexity.
- Global Themes: Managing light and dark themes across your application.
Transform Ideas into ReactJS Solutions!
SDLC CORP delivers cutting-edge, user-friendly ReactJS applications.
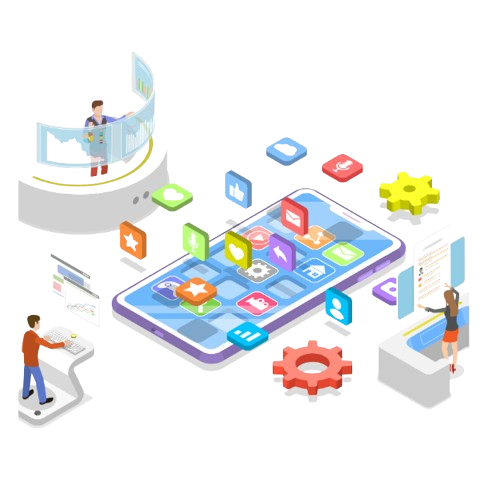
Best Practices for Using React Context
1. Combine Context with React Hooks
React hooks like useContext and useReducer enhance Context’s functionality. Using useReducer can mimic Redux-like functionality for more robust state management:
const reducer = (state, action) => {
switch (action.type) {
case ‘INCREMENT’:
return { count: state.count + 1 };
case ‘DECREMENT’:
return { count: state.count – 1 };
default:
return state;
}
};const CounterContext = createContext();
const CounterProvider = ({ children }) => {
const [state, dispatch] = useReducer(reducer, { count: 0 });
return (
<CounterContext.Provider value={{ state, dispatch }}>
{children}
</CounterContext.Provider>
);
};
Also check our latest blog :Creating Fast, Reliable Progressive Web Apps (PWAs) with React
2. Avoid Overusing Context
Overusing React Context can lead to performance issues. For large-scale applications, consider splitting Context into multiple smaller contexts.
3. Optimize Performance
Use memoization techniques such as React.memo and useMemo to avoid unnecessary re-renders when working on complex React development services.
React Context vs Redux: Which to Choose?
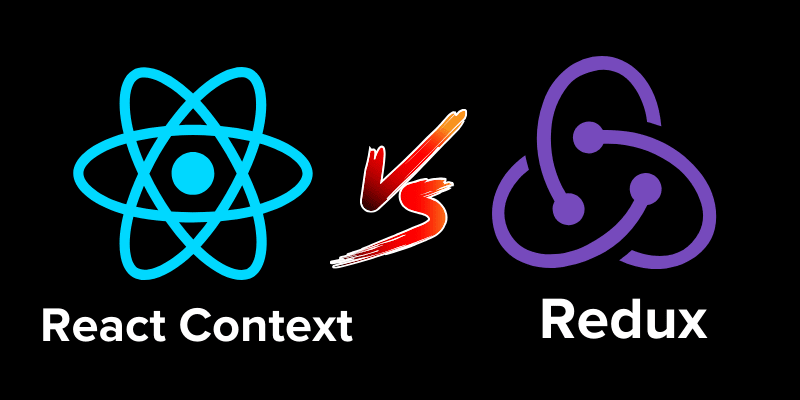
When to Choose React Context
- Small to medium-sized applications.
- Simple state requirements like themes or authentication.
- Projects requiring minimal setup and configuration.
When to Choose Redux
- Large-scale applications with complex state management needs.
- Scenarios involving asynchronous data fetching or middleware like redux-thunk or redux-saga.
For companies offering React JS development solutions, selecting the right tool depends on the project’s complexity and scalability requirements.
Common Mistakes to Avoid
1. Using Context for Everything
Not all state needs to be global. Evaluate if Context is necessary before implementation.
2. Forgetting to Optimize
Failing to optimize Context usage with React.memo or useMemo can lead to inefficient renders.
3. Ignoring Testing
Testing Context-based components can be tricky. Use tools like React Testing Library to validate Context functionality.
Build Modern Web Applications with ReactJS!
SDLC CORP ensures high-quality, responsive apps for all your business needs.
Conclusion
React Context is a powerful yet lightweight solution for efficient state management in your React applications. Whether you’re building a React mobile app, developing with a React JS development company, or creating a scalable React website development project, understanding and utilizing Context effectively can streamline your development process and improve code maintainability.
By embracing best practices and avoiding common pitfalls, you can unlock the full potential of React Context, ensuring your application remains scalable and efficient. If you need professional help, consider hiring a React JS developer or exploring React development services for expert guidance and support.
SDLC CORP ReactJS Development Services Overview
SDLC CORP is a leading React JS development company specializing in creating dynamic, scalable, and user-friendly web applications. Our expert developers leverage ReactJS to deliver high-performance solutions tailored to meet your business needs. From UI/UX design to custom development and seamless integration, we ensure your project stands out with cutting-edge technology and innovative features. Choose SDLC CORP for reliable and efficient React JS development services.