Introduction
In the fast-paced world of cryptocurrencies, real-time price monitoring is a critical feature for both users and exchange operators. The volatility of cryptocurrency markets makes it essential for traders to stay up-to-date with accurate, live price feeds. Whether you’re developing a crypto exchange platform from scratch or utilizing white-label crypto exchange software, implementing an effective real-time price monitoring system can significantly enhance your platform’s user experience.
In this blog, we will guide you through the importance of real-time price monitoring, the key steps to implement it, and how to ensure your platform remains competitive by leveraging advanced cryptocurrency exchange development services. Additionally, we’ll explore the role of crypto exchange development companies and the features of crypto exchange software development that help create robust, scalable, and responsive price monitoring systems.
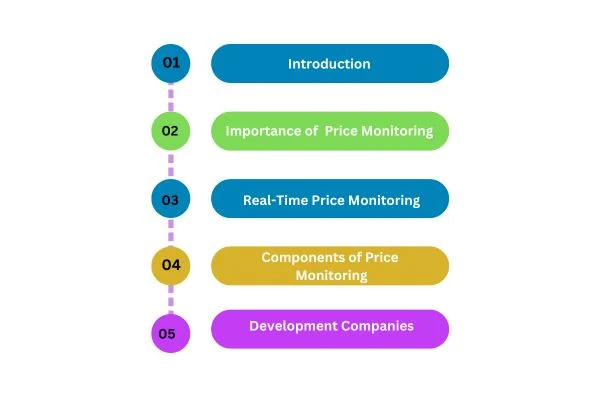
Steps to Implement Real-Time Price Monitoring
Now that we’ve covered the key components, let’s look at the steps to implement real-time price monitoring on a crypto exchange.
Step 1: Choose a Reliable Price Feed
Start by selecting the right price feed provider, whether it’s a third-party aggregator or a direct integration with other exchanges. Consider factors like data accuracy, frequency of updates, and the number of supported cryptocurrencies.
Step 2: Integrate WebSocket for Real-Time Updates
Integrate WebSocket into your exchange’s backend to push live price updates to users. WebSockets ensure low-latency, real-time communication, keeping the data flow uninterrupted.
Step 3: Build or Implement a Price Calculation Engine
If you’re using third-party price feeds, you might need to aggregate and normalize data into a consistent format. Alternatively, a custom price calculation engine can be developed to compute prices from various sources, adjust for spreads, and ensure accuracy.
Step 4: Set Up a High-Performance Database
Ensure you have a high-performance database to store historical price data and live updates. The database should be able to process a large amount of data quickly and efficiently, ensuring the accuracy and speed of price delivery.
Step 5: Optimize for Low Latency
Implement low-latency solutions, including CDN services, edge computing, and fast server infrastructure, to ensure that users receive price updates as quickly as possible without delays.
Step 6: Develop a User-Friendly UI
Design a user-friendly interface where traders can view live prices, historical trends, and set price alerts. Ensure the interface is responsive and scalable, capable of handling large traffic volumes during peak market hours.
Step 7: Test and Monitor
Once implemented, thoroughly test your system for any bugs, latency issues, or inaccuracies in the price data. Continuously monitor the performance of the system and make necessary adjustments to ensure it operates smoothly under high demand.
Why Real-Time Price Monitoring is Essential in Crypto Exchanges
Before diving into the technical details, let’s explore why real-time price monitoring is so crucial for crypto exchanges:
- Market Volatility: Cryptocurrencies are known for their price volatility. Prices can change rapidly, sometimes within seconds, so providing accurate, real-time price updates is essential for traders to make informed decisions.
- User Trust: Traders rely on timely and accurate data. If the price data is outdated or inaccurate, it can result in users losing trust in the platform, leading to reduced trading volume and user engagement.
- Arbitrage Opportunities: Many traders engage in arbitrage, exploiting price differences between exchanges. Real-time price monitoring enables users to quickly spot such opportunities, ensuring they can act before the market shifts.
- Market Making: For those who provide liquidity on the exchange, having access to real-time price data is crucial for making accurate buy and sell decisions.
- Regulatory Compliance: In some jurisdictions, exchanges are required to provide transparent and up-to-date price data. Real-time monitoring ensures your exchange complies with these regulations.
Given the critical nature of this feature, let’s explore how to implement real-time price monitoring effectively.
Key Components of Real-Time Price Monitoring
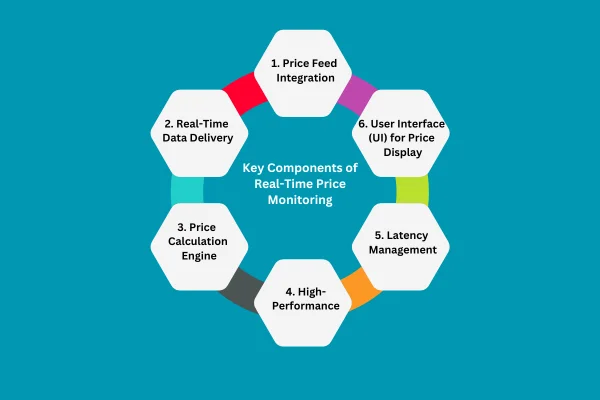
A real-time price monitoring system for a cryptocurrency exchange involves several key components. These components work together to fetch, process, and display price data in real-time for users. Below are the essential elements to consider when implementing real-time price monitoring:
1. Price Feed Integration
The most crucial component for real-time price monitoring is a reliable price feed. These feeds provide the market prices for cryptocurrencies, typically from various exchanges or aggregators. You can source price feeds in a few different ways:
- Direct API Integration: You can integrate directly with other exchanges’ APIs (like Binance, Kraken, or CoinMarketCap) to fetch the latest market prices. This method provides raw data from other trusted sources, but it requires ongoing maintenance to handle different API structures.
- Aggregators: Using aggregators like CoinGecko, CryptoCompare, or Nomics can save time and effort. These aggregators consolidate price data from various exchanges, ensuring you get accurate market data without integrating with individual platforms.
- Custom Price Feeds: If you’re running a large platform, you may choose to build custom price feeds using market-making algorithms. These algorithms calculate prices based on a variety of factors, such as trading volume, liquidity, and historical data.
2. WebSocket for Real-Time Data Delivery
WebSockets are essential for real-time communication. Unlike traditional HTTP protocols, which require frequent polling for updates, WebSockets maintain an open connection between the server and the client. This allows data to flow continuously and instantly as new price information becomes available.
With WebSocket integration, users will receive live price updates directly in their browser without needing to refresh the page or request new data manually. This provides a seamless and uninterrupted experience for users, ensuring they have the most up-to-date prices at all times.
3. Price Calculation Engine
The price calculation engine is responsible for processing incoming data and calculating the accurate price of cryptocurrencies. This system can include:
- Spot Prices: The current market price of a cryptocurrency.
- Market Average: The average price across several exchanges to avoid discrepancies from individual exchanges.
- Spread: The difference between the buy and sell price of a cryptocurrency, which can indicate liquidity.
The price calculation engine must be fast, efficient, and capable of handling large amounts of data in real time. This is essential for ensuring the smooth operation of your platform during high trading volumes.
Also Read: Innovati ve Crypto Exchange Development Trends Shaping the Future
4. High-Performance Database
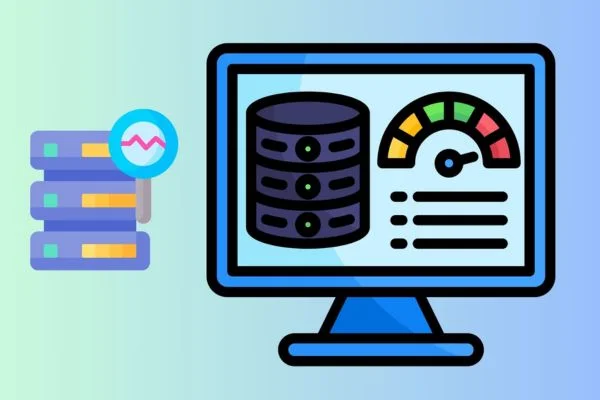
Since real-time price monitoring involves handling vast amounts of data, your exchange needs a robust database to store and process information. Key requirements include:
- Speed: The database must be able to handle high-frequency updates without slowing down the system.
- Scalability: As your exchange grows, the system should scale seamlessly to accommodate more users and trading pairs.
- Data Integrity: It’s important that the database accurately reflects the latest prices, ensuring no discrepancies.
A NoSQL database, such as MongoDB, is often preferred for its flexibility and speed when dealing with high-velocity data.
5. Latency Management
Latency refers to the delay between when data is generated and when it is reflected on the user interface. Low latency is crucial for real-time price monitoring systems, as high latency can lead to inaccurate or outdated prices.
You can reduce latency through several techniques:
- Content Delivery Networks (CDNs) to ensure that price data is distributed efficiently to users around the world.
- Edge computing to process data closer to the user, reducing the distance that information has to travel.
- Optimizing server performance through dedicated servers and cloud infrastructure to ensure fast processing of price updates.
6. User Interface (UI) for Price Display
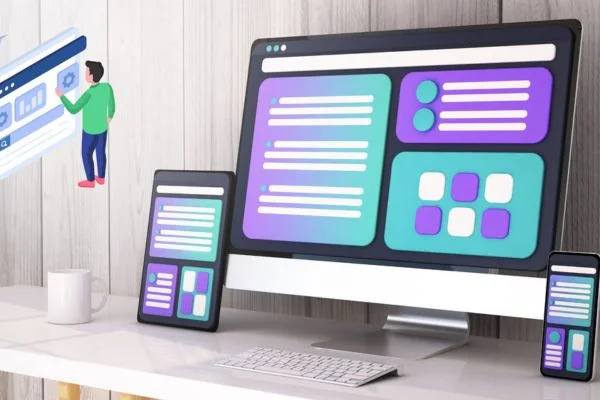
The user interface is where users will view the real-time prices. For an optimal user experience, the price monitoring interface should include:
- Live Price Tickers: Displaying live market prices for various cryptocurrencies.
- Price Charts: Interactive charts that visualize price trends over time, such as candlestick charts or line graphs.
- Price Alerts: Allow users to set price alerts to be notified when a cryptocurrency reaches a specific price threshold.
These features should be visually appealing, intuitive, and responsive to ensure users can easily track prices across different cryptocurrencies and trading pairs.
Also Read: Building a Global Crypto Exchange Challenges and Solutions
Leveraging Crypto Exchange Development Companies for Price Monitoring
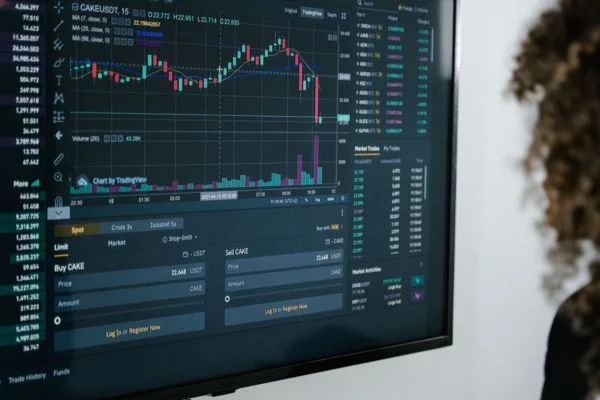
Implementing real-time price monitoring can be a complex task, and it’s essential to work with a crypto exchange development company that has experience in building high-performance platforms. These companies specialize in cryptocurrency exchange development, and they can provide the expertise needed to build scalable and efficient systems.
Why Hire a Crypto Exchange Development Company?
- Expertise in Crypto Platforms: Experienced developers have the knowledge to implement complex features like real-time price monitoring with minimal issues.
- Customization: Whether you’re using white-label crypto exchange software or developing a custom platform, they can tailor the features to your specific needs.
- Security: A crypto exchange software development company can help you implement the necessary security measures, including encryption and two-factor authentication, to protect user data.
- Scalability: They can design the system to handle a growing user base, ensuring your platform remains fast and efficient as it expands.
Secure Cryptocurrency Trading Solutions
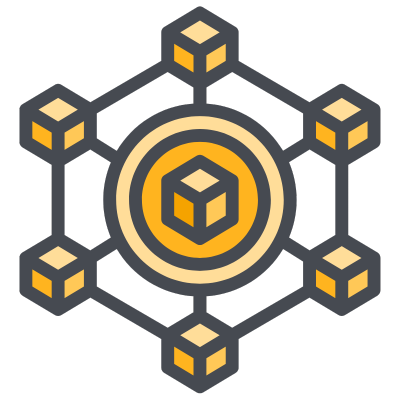
Conclusion
Real-time price monitoring is a core feature for any successful cryptocurrency exchange platform. By integrating accurate price feeds, using WebSocket technology for low-latency updates, and optimizing your platform for scalability, you can ensure that your users have the best possible trading experience. Whether you’re using white-label crypto exchange software or working with a crypto exchange development company, taking the right steps to implement real-time price monitoring will help you stay competitive in the dynamic world of cryptocurrency trading.
Also Read:
How to Attract New Traders to Your Crypto Exchange?
The Role of Arbitrage Trading in Crypto Exchanges
How to Manage Transaction Fees on Crypto Exchange Platforms?
FAQs
Why is real-time price monitoring important for crypto exchanges?
Real-time price monitoring is essential because the cryptocurrency market is highly volatile. Price fluctuations can occur within seconds, and users need accurate, up-to-date information to make informed trading decisions. Providing real-time price updates enhances user trust, supports arbitrage opportunities, and enables market makers to react quickly to price changes. Without this feature, users might face outdated information, leading to poor trading decisions and a loss of trust in the platform.
What are the key components required for real-time price monitoring on a crypto exchange?
There are several key components that work together to provide real-time price monitoring:
- Price Feed Integration: You need to integrate reliable price data sources, which could include APIs from other exchanges or price aggregators.
- WebSocket Technology: WebSockets allow real-time, low-latency communication between the server and clients. This ensures that users receive live price updates without needing to refresh their page.
- Price Calculation Engine: This engine processes data from multiple price feeds, calculates the accurate price, and accounts for spreads or liquidity differences.
- Database: A high-performance database stores price data, supports fast querying, and ensures real-time data updates are reflected across the platform.
- User Interface (UI): The UI displays real-time prices to users, often featuring price tickers, charts, and alerts for better trading decisions.
How do price feeds work in a real-time monitoring system?
Price feeds provide the raw data for real-time price monitoring. These can be sourced directly from exchanges using APIs or through aggregators that combine data from multiple exchanges. The feed updates regularly (usually every second or millisecond) and is processed by the price calculation engine to ensure accuracy. Depending on the configuration, you can either rely on a single source or aggregate data from various exchanges to present the most accurate market price.
What role does WebSocket play in real-time price updates?
WebSocket is a protocol that allows for full-duplex communication channels over a single TCP connection. In the context of real-time price monitoring on crypto exchanges, WebSockets enable live, continuous updates between the server and the client. Unlike traditional HTTP requests, which require clients to continuously poll for data, WebSockets allow the server to push price updates to users as soon as new data becomes available, making it more efficient and reducing latency.
What is a price calculation engine, and why is it important?
A price calculation engine is responsible for processing raw data from various price feeds and calculating the final price that will be displayed on your platform. It aggregates prices, adjusts for spreads, and determines the most accurate and fair price for a particular cryptocurrency or trading pair. This engine ensures that users are presented with reliable price information across multiple trading pairs, helping them make informed decisions.