Introduction
Building a chess game using Python was both a challenging and rewarding experience. Python is known for its simplicity and ease of learning, making it a great language for beginners and experts alike. I’ve always been fascinated by chess, not only because of its rich history but also due to its intellectual depth and strategy. So, when I decided to take on this project, I knew it would involve both technical programming skills and a deep understanding of the game.
In this guide, I’ll take you through the steps I followed to create a fully functional chess game using Python, and I’ll discuss the process from setting up the environment to implementing the game mechanics, designing the user interface, and testing. If you’re interested in chess game development services or you’re part of a chess game development company, this guide will provide valuable insights. Whether you’re an aspiring developer or someone looking to understand how chess game development works, this step-by-step breakdown will be helpful.
Start your Chess game development today!
Launch your next big Chess game with our expert development team.
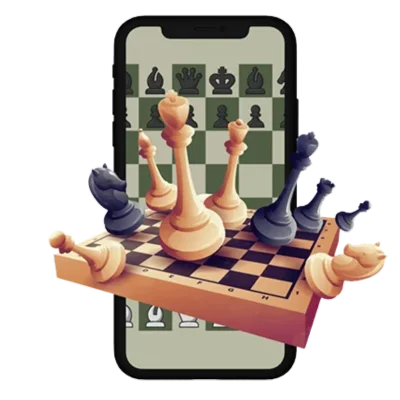
Step 1: Setting Up the Development Environment
The first step in creating a chess game in Python is setting up the environment. For this project, I used the Python programming language because of its simplicity and robust libraries. Here’s how I went about it:
- Install Python: If you don’t already have Python installed, you can download it from the official Python website. Make sure to install the latest version.
- Choose an IDE: An Integrated Development Environment (IDE) makes coding easier. I used PyCharm for this project, but you can use any other IDE like VS Code or Jupyter Notebooks.
Install Required Libraries: Python offers a variety of libraries that make building games easy. For this project, I used the pygame library, which is designed for writing video games. To install it, run:
bash
Copy code
pip install pygame
This simple setup allows us to start coding the chess game immediately.
Step 2: Understanding Chess Rules and Game Logic
Before writing any code, I needed a solid understanding of how chess works. Chess involves two players, each controlling 16 pieces, including pawns, rooks, knights, bishops, a queen, and a king. Each piece has its own movement rules. The game is won by putting the opponent’s king in checkmate.
The key components of the game that needed to be coded include:
- Board representation: An 8×8 grid where pieces can move.
- Pieces: The different chess pieces and their movement rules.
- Game rules: Checking for valid moves, check, and checkmate.
Turn-based system: Allowing players to alternate between turns.
Step 3: Designing the Chess Board
The first task was to create a digital chessboard. Chess is played on an 8×8 board, alternating between black and white squares. In Python, we can use a simple matrix to represent this board. Here’s an example:
python
Copy code
board = [
[“r”, “n”, “b”, “q”, “k”, “b”, “n”, “r”],
[“p”, “p”, “p”, “p”, “p”, “p”, “p”, “p”],
[” “, ” “, ” “, ” “, ” “, ” “, ” “, ” “],
[” “, ” “, ” “, ” “, ” “, ” “, ” “, ” “],
[” “, ” “, ” “, ” “, ” “, ” “, ” “, ” “],
[” “, ” “, ” “, ” “, ” “, ” “, ” “, ” “],
[“P”, “P”, “P”, “P”, “P”, “P”, “P”, “P”],
[“R”, “N”, “B”, “Q”, “K”, “B”, “N”, “R”]
]
In this setup, lowercase letters represent black pieces and uppercase letters represent white pieces. I used “r” for rooks, “n” for knights, “b” for bishops, “q” for queens, “k” for kings, and “p” for pawns.
Step 4: Creating the Game Pieces
Once the board was set, the next step was to create the individual pieces and define how they move. Each piece has specific rules for movement:
- Pawns: Move one square forward but capture diagonally.
- Rooks: Move horizontally or vertically any number of squares.
- Knights: Move in an “L” shape (two squares in one direction and one square perpendicular).
- Bishops: Move diagonally any number of squares.
- Queens: Can move horizontally, vertically, or diagonally any number of squares.
- Kings: Can move one square in any direction.
To implement these movements in Python, I created individual classes for each piece type. For example, here’s a simplified version of the code for a knight’s movement:
python
Copy code
class Knight:
def __init__(self, color):
self.color = color
def valid_moves(self, position):
x, y = position
moves = [
(x+2, y+1), (x+2, y-1),
(x-2, y+1), (x-2, y-1),
(x+1, y+2), (x+1, y-2),
(x-1, y+2), (x-1, y-2)
]
return [move for move in moves if 0 <= move[0] < 8 and 0 <= move[1] < 8]
This function returns a list of valid moves for the knight based on its position.
Start your Chess game development today!
Launch your next big Chess game with our expert development team.
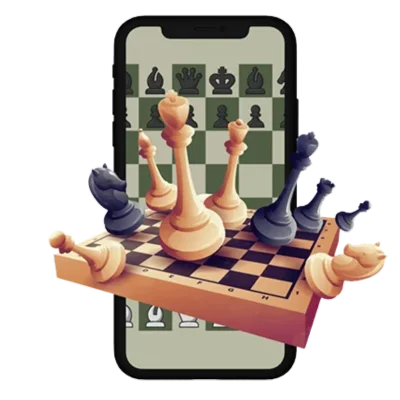
Step 5: Implementing the Turn-Based System
Next, I needed to implement a system that allows players to take turns. In chess, players alternate turns, starting with white. I added a simple check at the beginning of each move to determine which player’s turn it is:
python
Copy code
def switch_turn(turn):
return “white” if turn == “black” else “black”
This function alternates between “white” and “black” after each turn.
Step 6: Checking for Check and Checkmate
In chess, check occurs when a player’s king is under immediate threat of capture, while checkmate occurs when a player’s king is in check and cannot escape. To implement these checks, I created a function that evaluates the positions of all pieces on the board and determines whether the king is in danger:
python
Copy code
def is_check(board, king_position, opponent_pieces):
for piece in opponent_pieces:
if king_position in piece.valid_moves():
return True
return False
Checkmate is more complex, requiring the evaluation of every possible move to see if the king can escape.
Step 7: Building the User Interface (UI)
Once the game mechanics were in place, it was time to create a visual representation of the game. Using the pygame library, I built a simple UI to display the chessboard and allow players to interact with it. Pygame makes it easy to draw images, handle events, and create animations.
Here’s how I created the chessboard using pygame:
python
Copy code
import pygame
# Initialize pygame
pygame.init()
# Define constants
WIDTH, HEIGHT = 600, 600
WIN = pygame.display.set_mode((WIDTH, HEIGHT))
def draw_board():
WIN.fill((255, 255, 255))
for row in range(8):
for col in range(8):
if (row + col) % 2 == 0:
pygame.draw.rect(WIN, (255, 255, 255), (col*75, row*75, 75, 75))
else:
pygame.draw.rect(WIN, (0, 0, 0), (col*75, row*75, 75, 75))
pygame.display.update()
# Main game loop
running = True
while running:
for event in pygame.event.get():
if event.type == pygame.QUIT:
running = False
draw_board()
pygame.quit()
This code draws an 8×8 grid where each square alternates between black and white, mimicking a chessboard. The next step would be to add the pieces, handle mouse clicks, and update the game state based on player input.
Step 8: Testing the Game
After implementing the game mechanics and UI, it was time to test the game. I played several rounds to ensure that all pieces moved correctly, that turns alternated, and that check and checkmate were accurately detected. During this process, I also tested edge cases, such as castling, pawn promotion, and en passant, to ensure that the game followed the official rules of chess.
Start your Chess game development today!
Launch your next big Chess game with our expert development team.
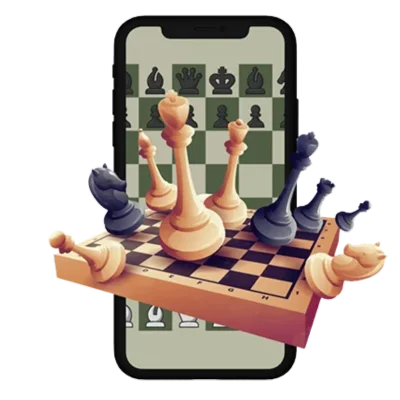
Conclusion
Developing a chess game using Python was an incredibly fulfilling project. It helped me sharpen my programming skills and taught me a lot about game development. Whether you’re working as part of a chess game development company or simply exploring chess game development as a hobby, Python provides a versatile platform for building such applications. If you’re looking for professional chess game development services or want to take your project to the next level, many chess game app development companies specialize in creating polished, feature-rich chess games.
I hope this step-by-step guide has been helpful, and I encourage you to try building your own chess game or improving upon the one I’ve outlined here.